Question
Create a class called ArrayUtility.java which will contain methods to perform array manipulations. There are no instance variables required in this class. Write each of
Create a class called ArrayUtility.java which will contain methods to perform array manipulations. There are no instance variables required in this class. Write each of the methods to solve each task as described below. You have been provided with a test driver class. 2 Class diagram:
ArrayUtility
+ findPercentageGreaterThanTwenty(intArray:int[]):double
+ findSmallestInt(intArray:int[]):int
+ findLargestInt(intArray:int[]):int
+ findDifferenceLargestSmallest(int[] intArray):int
+ printReverseOrder(intArray:int[]):void
+ increaseValues(intArray:int[]):void
Requirements for each method. All of these methods receives an integer array of any length that has all of the elements filled. 1. findPercentageGreaterThanTwenty
a. Write the code to return the percentage of elements greater than 20
2. findDifferenceLargestSmallest
a. This method should use the findSmallestInt and findLargestInt methods and then calculate the difference of the smallest from the largest.
3. printReverseOrder
a. Write the code to print all of the values in the array to the screen in reverse order. Put each number on a separate line and label it with the element name.
b. Example Output of one element: intArray[9] = 22
4. increaseValues
a. Write the code to increase the value in each element of the array by a factor of 4.
Here is the ArrayDriver.java
package edu.ilstu;
/**
* Tester for ArrayUtility
*
*
*
*/
public class ArrayDriver
{
public static void main(String[] args)
{
ArrayUtility util = new ArrayUtility();
/*
* This is the array that will be used to test the methods
* to be written in the ArrayUtility class.
*/
int[] intArray = {14, 6, 27, 44, 3, 51, 36, 39, 11, 22};
//Test percentageGreaterThanTwenty
System.out.println("Problem 1 - find percentage greater than 20 ");
double percentage = util.findPercentageGreaterThanTwenty(intArray);
if (percentage == 0.6){
System.out.println("Correct answer: " + percentage);
} else {
System.out.println("Incorrect");
}
//Test find diffence between the largest and smallest
System.out.println(" Problem 2 - find difference");
int difference = util.findDifferenceLargestSmallest(intArray);
if (difference == 48){
System.out.println("Correct answer: " + difference);
} else {
System.out.println("Incorrect");
}
//Test printIntArray
System.out.println(" Problem 3 - print contents of array");
System.out.println(" Original array:");
for (int j = 0; j < intArray.length; j++){
System.out.println("intArray[" + j + "] = " + intArray[j]);
}
System.out.println(" Array in reverse order:");
util.printReverseOrder(intArray);
//Test increaseValue
System.out.println(" Problem 4 - increase the value of each element by multiplying by 4");
System.out.println(" Original array:");
for (int j = 0; j < intArray.length; j++){
System.out.println("intArray[" + j + "] = " + intArray[j]);
}
util.increaseValues(intArray);
System.out.println(" Array after increase:");
for (int j = 0; j < intArray.length; j++){
System.out.println("intArray[" + j + "] = " + intArray[j]);
}
}
}
And here is what I have so far in ArrayUtility.java
package edu.ilstu;
/**
* Array Utility
*
* @author
*
*/
public class ArrayUtility
{
public double findPercentGreaterThanTwenty(int[] intArray) {
}
public int findSmallestInt(int[] intArray)
{
int smallest = Integer.MAX_VALUE;
for (int i=0;i
if(smallest > intArray[i]) {
smallest = intArray[i];
}
return smallest;
}
}
public int findLargestInt(int[] intArray) {
int n = intArray.length;
for(int i=1;i
{
if(intArray[0]
intArray[0]=intArray[i];
}
return intArray[0];
}
public int findDifferenceLargestSmallest(int[] intArray) {
}
public void printReverseOrder(int[] intArray) {
}
public void increaseValues(int[] intArray) {
int arr[]=new int[intArray.length];
for(int i=0;i
arr[i]=intArray[i]+4*i;
}
for(int i=0; i
System.out.print(arr[i]+"");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
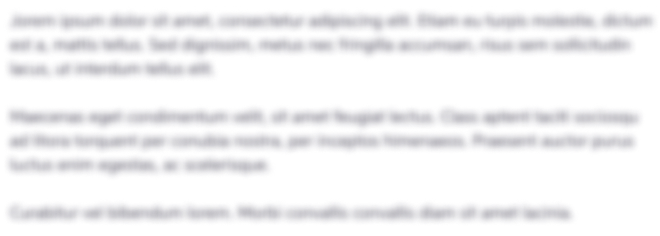
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started