Question
Create a class CustomerOrder to have the following member variables. ***IN C++*** NO COMPLICATED STUFF!!! class CustomerOrder{ private: int orderId; double orderAmount; string customerName; Write
Create a class CustomerOrder to have the following member variables. ***IN C++*** NO COMPLICATED STUFF!!!
class CustomerOrder{ private:
int orderId; double orderAmount; string customerName;
Write a program that creates a priority queue for CustomerOrder * (pointer to CustomerOrder objects). The priority is determined by the value of orderId where 1 has the highest priority. Insert the following three objects.
CustomerOrder c1(1, 100.0, "customer1"); CustomerOrder c2(3, 50.0, "customer3"); CustomerOrder c3(2, 300.0, "customer2");
Then create the priority queue named orders then insert pointers to the customer orders above. orders.insert(&c1); orders.insert(&c2); orders.insert(&c3);
Print the details of each object as you remove them from the priority queue. Output should be something like:
orderId:1, orderAmount:100, customerName:customer1 orderId:2, orderAmount:300, customerName:customer2 orderId:3, orderAmount:50, customerName:customer3
code is below, in c++ only please
**DO NOT USE #INCLUDE
//priorityQ.cpp
//demonstrates priority queue
#include
#include
using namespace std;
////////////////////////////////////////////////////////////////
class PriorityQ {
//vector in sorted order, from max at 0 to min at size-1
private:
int maxSize;
vector < double > queVect;
int nItems;
public:
//-------------------------------------------------------------
PriorityQ(int s): maxSize(s), nItems(0) //constructor
{
queVect.resize(maxSize);
}
//-------------------------------------------------------------
void insert(double item) //insert item
{
int j;
if (nItems == 0) //if no items,
queVect[nItems++] = item; //insert at 0
else //if items,
{
for (j = nItems - 1; j >= 0; j--) //start at end,
{
if (item > queVect[j]) //if new item larger,
queVect[j + 1] = queVect[j]; //shift upward
else //if smaller,
break; //done shifting
} //end for
queVect[j + 1] = item; //insert it
nItems++;
} //end else (nItems > 0)
} //end insert()
//-------------------------------------------------------------
double remove() //remove minimum item
{
return queVect[--nItems];
}
//-------------------------------------------------------------
double peekMin() //peek at minimum item
{
return queVect[nItems - 1];
}
//-------------------------------------------------------------
bool isEmpty() //true if queue is empty
{
return (nItems == 0);
}
//-------------------------------------------------------------
bool isFull() //true if queue is full
{
return (nItems == maxSize);
}
//-------------------------------------------------------------
}; //end class PriorityQ
//////////////////////////////////////////////////////////////// adding set and get to private
int main() {
class CustomerOrder
{
private:
int orderid;
double orderAmount;
string CustomerName;
};
PriorityQ thePQ(5); //priority queue, size 5
thePQ.insert(30); //unsorted insertions
thePQ.insert(50);
thePQ.insert(10);
thePQ.insert(40);
thePQ.insert(20);
while (!thePQ.isEmpty()) { //sorted removals
double item = thePQ.remove();
cout << item << " "; //10, 20, 30, 40, 50
} //end while
cout << endl;
return 0;
} //end main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
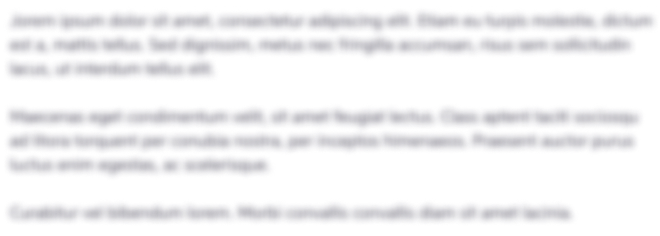
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started