Question
create a class in BlueJ called DoubleArrayMethods. You will add some static methods to this class that you will then use to write a program
create a class in BlueJ called DoubleArrayMethods. You will add some static methods to this class that you will then use to write a program that processes grades stored in an array. You must write your own methods and cannot use any existing library methods. Add a static method that sorts an array of double values in ascending order using the bubble sort algorithm.
Add a static method that sorts an array of double values in descending order using the bubble sort algorithm.
Add a static method that returns the average of an array of double values.
Add a static method that returns the minimum value of an array of double values.
Add a static method that returns the maximum value of an array of double values. For questions 13 to 19, create a class called GradeManager, in the same project as the DoubleArrayMethods, class that will be used to write a program that will allow users to enter grades into an array and perform some basic operations on the grades. This will be similar to the example demonstrated in class (the Driver class) The class should have two fields. One an array of double values called grades and the other an integer called numberOfGrades. This field will keep track of the number of grades currently stored in grades. The class should also have a constant called MAX_GRADES which will be the maximum number of grades and the initial length of the grades array.
Write a constructor that initializes the two fields appropriately.
Write a private method called displayMenu that displays the following menu of choices:
Enter the number of one of the following choices: 1. Add a new score. 2. Sort grades. 3. List all grades. 4. Print statistics. 5. Quit.
Write a private method called getUserChoice that returns the integer value of the menu choice made by the user.
Write a private method called getGrade that allows the user to enter only a single grade from the keyboard that will be placed at the end of the current list of grades. Be sure to check that the array is not full. If it is simply report that no more grades can be entered. You do not need to increase the size of the array. Write a private method called sorter that will ask the user if they want to sort the grades in ascending or descending order and then sorts the grades appropriately. This method does not display the grades. You should call the appropriate sorting method you wrote in the previous exercises.
Write a private method called printGrades that simply lists each grade, one per line.
Write a private method called printStats that prints the average of all the grades and the highest and lowest grades. You should call the appropriate method(s) you wrote previously.
Write a public method called start that does the following:
Displays the menu Gets the users menu choice. Use a switch statement to execute the users choice. Use a do while loop to execute until the user quits. When a user chooses to enter a new grade, only a single grade is entered at the end of the current list of grades. Be sure to check if the array is full. If it is, simply report that no more grades can be entered. You do not need to increase the size of the array.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
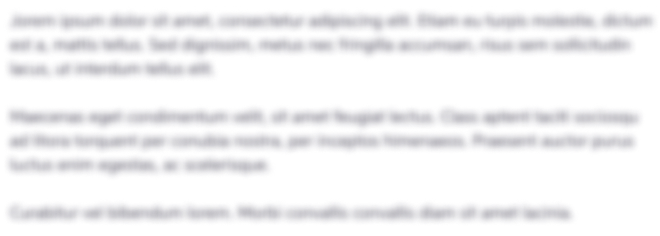
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started