Question
Create a class in java with the following information: The Payroll Class contains three fields and methods to calculate the wage of an employee. (1)
Create a class in java with the following information: The Payroll Class contains three fields and methods to calculate the wage of an employee.
(1) Please write an application that would allow the user to enter employees name, pay rate, and number of hours he/she worked last week. Use the information to instantiate objects of Payroll class, and add each object into an ArrayList.
(2) The user can enter any number of employees. The program should ask the user whether there is any more data to be entered.
(3) After the user finishes entering the payroll information, print all the payroll information. (NOTE: you can directly use the toString() method of ArrayList.
(4) Use a loop to traverse the ArrayList, get each object, use the calWage() method of Payroll to calculate the total wage the employer needs to pay this week.
This is the payroll class that should be used public class Payroll { public final double MINIMUM_RATE = 10.25; // defines the minimum hourly pay rate public final int REGULAR_HOUR = 40; // defines the number of regular hours public final double OVERTIME_RATE = 1.5; // defines the pay rate for overtime hours private String empName; // employee name private double payRate; // payRate for an employee private int numOfHours; // hours worked for an employee // no-arg constructors. It will set the empName to null, payRate and numOfHours to 0. public Payroll(){} public Payroll(String n, double r, int h) { empName = n; // if the rate is less than the minimum pay rate // set it to the minimum pay rate if (r < MINIMUM_RATE) payRate = MINIMUM_RATE; else payRate = r; // if the hours is negative, set it to 0 if (h<0) numOfHours = 0; else numOfHours = h; } // getters and setters for the three fields public String getEmpName() { return empName; } public void setEmpName(String empName) { this.empName = empName; } public double getPayRate() { return payRate; } public void setPayRate(double rate) { // if the rate is less than the minimum pay rate // set it to the minimum pay rate if (rate < MINIMUM_RATE) payRate = MINIMUM_RATE; else payRate = rate; } public void setPayRate(String rateString) { // convert the string to a double number double rate = Double.parseDouble(rateString); // if the rate is less than the minimum pay rate // set it to the minimum pay rate if (rate < MINIMUM_RATE) payRate = MINIMUM_RATE; else payRate = rate; } public int getNumOfHours() { return numOfHours; } public void setNumOfHours(int numOfHours) { // if the hours is negative, set it to 0 if(numOfHours<0) this.numOfHours=0; else this.numOfHours = numOfHours; } public void setNumOfHours(String hourString){ // convert String to a integer number int hours = Integer.parseInt(hourString); numOfHours = hours; } public double calWage(){ // Overtime pay if(numOfHours > REGULAR_HOUR) return payRate * ((numOfHours-REGULAR_HOUR)* OVERTIME_RATE+ REGULAR_HOUR); // regular pay else return payRate * numOfHours; } public String toString(){ return empName+" worked "+ numOfHours+ " hour(s) at $"+ payRate +" per hour. ";
Step by Step Solution
There are 3 Steps involved in it
Step: 1
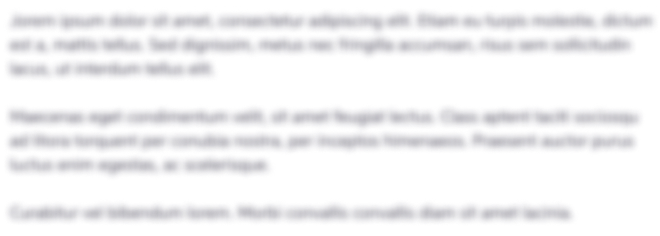
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started