Question
Create a class structure to represent animals housed at an animal shelter. The shelter will support cats, dogs, and reptiles. The application will maintain information
Create a class structure to represent animals housed at an animal shelter. The shelter will support cats,
dogs, and reptiles. The application will maintain information about the animals and support accepting
animals and the adoption of animals.
Service Classes
Animal, abstract class
Cat,
Dog,
Reptile
Design an Animal abstract class that defines fields and methods that are common among all animals at our
shelter. The Animal abstract class is to be extended by three subclasses representing the three different
types of animals taken in by our shelter: cats, dogs, and reptiles.
For each animal, we will note the animal's name, general description, approximate age and the arrival and
adoption dates. Each animal should also have a unique shelter id which is assigned when a pet is turned
over to the shelter. For Cats and Dogs, we will also record the animal's bred and sex. The type of reptile
(snake, turtle, lizard, etc) should be noted for Reptiles.
Shelter class
The Shelter class will manage Cat, Dog, and Reptile objects using a single ArrayList. The Shelter class
should implement the AnimalShelter interface (see included at bottom) The interface
represents the minimum requirements of the Shelter class. Additional methods may be implemented to
improve the class.
Client Application
Create a client application to support the Shelter. The end-user should be able to view pets with a variety of
options, adopt pets and turn over a pet to the shelter. The application should be menu-driven, allowing the
end-user to continue until exit is requested.
Hints:
A static class field is shared among all instances of a class. Any changes to a static class field will be
reflected in all instances. The static class field can be used to initialize the id field for an Animal and then
incremented. This will result in a unique id for each animal accepted at the shelter.
The Date and Calendar API classes can be used to support the required date fields. For example, the
current date and time can be retrieved using:
Date now = Calendar.getInstance().getTime();
The instanceof operator can be used to determine if an object instance is of a particular class:
Cat myCat= new Cat("Snow White","Domestic Short Hair","Cute little ball of fur",1,"F");
if (myCat instanceof Cat) // will be true
if (myCat instanceof Dog) // will be false
For testing purposes, initializing the shelter by creating a few pet instances within the client and
adding to the shelter.
At the end of this there will be 8 classes in total
Can you write this using jgrasp and fallow the directions given ?
AnimalShelter interface
public interface AnimalShelter { /** Maximum number of Cats sheltered */ public static final int CATSMAX = 30; /** Maximum number of Dogs sheltered */ public static final int DOGMAX = 20; /** Maximum number of Reptiles sheltered */ public static final int REPTILEMAX = 5; /** allAnimals displays all Animals sheltered including those that have * been adopted. */ public void allAnimals(); /** available produces a display of all Animals available for adoption. */ public void available(); /** adopted produces a display of all animals that have been adopted. */ public void adopted(); /** allType produces a display of all animals of a particular type. * @param int representing the type of animal - Cat, Dog, or Reptile * @param boolean indicator of whether to include adopted animals. */ public void allType(int animalType, boolean adopted); /** adopt changes an animal's status to adopted. * @param int id of the animal to be adopted * @return boolean indicator of success. False will be returned when the animal's status already * indicates adopted or the id was not found. */ public boolean adopt(int id); /** accepts an animal into the shelter. * @param Animal object to be added to the shelter * @return boolean indicator of success. False will be returned when the animal can not be accomodated. */ public boolean accept(Animal pet); /** accepts an animal into the shelter that was previously sheltered. * @param int id of the animal being returned to the shelter. * @return boolean indicator of success. False will be returned when the animal can not be accomodated. */ public boolean accept(int id); }
the original full question in its unedited format this should provide the details needed (if you need more info let me know)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
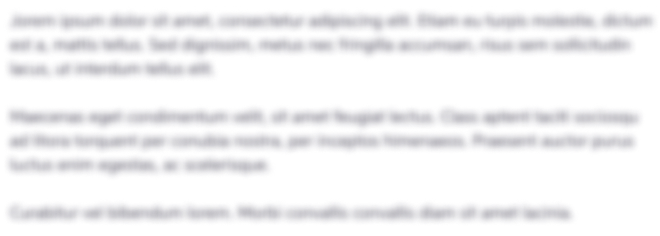
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started