Question
Create a Grid class for a battleship program. Make sure to include a Grid.java, and a GridTester.java. Include Javadoc, and comments. Grade 12 level coding
Create a Grid class for a battleship program. Make sure to include a Grid.java, and a GridTester.java. Include Javadoc, and comments. Grade 12 level coding style using java only. Thank you.
Now that we have written the Ship class and the Location class, the next thing is to write the Grid class. The Grid class represents a Battleship Grid both for the user player and the computer. This will be used both as a grid to track where your ships are, as well as track the guesses that the user or computer has made.
The main state to track in the Grid is a 2-dimensional array of Location objects.
A few important variables youll want to have here are
private Location[][] grid; // Constants for number of rows and columns. public static final int NUM_ROWS = 10; public static final int NUM_COLS = 10;
To start off, you should implement the basic functionality to make this Grid class a little easier to use than a simple 2D array. Here are the methods you need to implement.
// Create a new Grid. Initialize each Location in the grid // to be a new Location object. public Grid() // Mark a hit in this location by calling the markHit method // on the Location object. public void markHit(int row, int col) // Mark a miss on this location. public void markMiss(int row, int col) // Set the status of this location object. public void setStatus(int row, int col, int status) // Get the status of this location in the grid public int getStatus(int row, int col) // Return whether or not this Location has already been guessed. public boolean alreadyGuessed(int row, int col) // Set whether or not there is a ship at this location to the val public void setShip(int row, int col, boolean val) // Return whether or not there is a ship here public boolean hasShip(int row, int col) // Get the Location object at this row and column position public Location get(int row, int col) // Return the number of rows in the Grid public int numRows() // Return the number of columns in the grid public int numCols() // Print the Grid status including a header at the top // that shows the columns 1-10 as well as letters across // the side for A-J // If there is no guess print a - // If it was a miss print a O // If it was a hit, print an X // A sample print out would look something like this: // // 1 2 3 4 5 6 7 8 9 10 // A - - - - - - - - - - // B - - - - - - - - - - // C - - - O - - - - - - // D - O - - - - - - - - // E - X - - - - - - - - // F - X - - - - - - - - // G - X - - - - - - - - // H - O - - - - - - - - // I - - - - - - - - - - // J - - - - - - - - - - public void printStatus() // Print the grid and whether there is a ship at each location. // If there is no ship, you will print a - and if there is a // ship you will print a X. You can find out if there was a ship // by calling the hasShip method. // // 1 2 3 4 5 6 7 8 9 10 // A - - - - - - - - - - // B - X - - - - - - - - // C - X - - - - - - - - // D - - - - - - - - - - // E X X X - - - - - - - // F - - - - - - - - - - // G - - - - - - - - - - // H - - - X X X X - X - // I - - - - - - - - X - // J - - - - - - - - X - public void printShips()
Now we need to extend our Grid class with a new method.
/** * This method can be called on your own grid. To add a ship * we will go to the ships location and mark a true value * in every location that the ship takes up. */ public void addShip(Ship s)
This method should go to the Locations in the Grid that the Ship is in and set the boolean value to true to indicate that there is a ship in that location. You have some handy helper methods already to do this!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
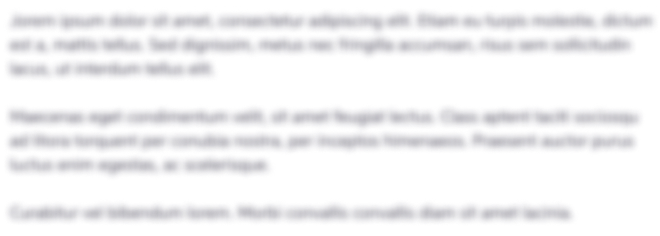
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started