Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Create a Hello C++! Program Create a program that simply outputs the text Hello C++! I love CS52 when you run it. This can be
- Create a Hello C++! Program
- Create a program that simply outputs the text Hello C++! I love CS52 when you run it. This can be done by using cout object in the main function.
- Before you do anything else, run this program and make sure that the program outputs the text without bug.
- Leave all code as is and move on to the next step. We will program everything in one single .cpp file.
- Create a Class and an Object
- In the same file as which contain the main function, define a new class called Bus. The word Bus must start with an uppercase because the name of a class should start with an uppercase by convention (so that it is different that a variable name). It can be an empty class, without property and functionality.
- In the main function, right below where you output Hello C++! I love CS52, create an object from the class. You can name the object however you like with a meaningful word.
- Run your program to make sure that it doesnt have an error.
- Basic Constructors
- Add a keyword public with a colon.
- Modify your Bus Class by adding a constructor, a default constructor. Make the constructor output the text Bus Default Constructor is called! This constructor will not have an argument.
- After running the program, the text from a default constructor will show.
- Leave all code as is and move on to the next step.
- Multiple Constructors
- Modify the Bus class so that it has another constructor. Adding a new constructor where it accepts a parameter called busDriverName of type String.
- Make this second constructor print the name parameter using cout object. Simply, taking the argument and use cout to output the name to the terminal screen.
- Add another object in your main function by calling the second constructor so that when you create the Bus object, you pass in a name. Please pass in your name to the constructor when you call the new constructor.
- Instance Variable, Also Know as Data Members
- Add a keyword private with a colon.
- Add a member variable to the Bus class. A variable with type string with a name name.
- Modify the second constructor. In your second constructor, instead of simply print out a driver name. The constructor will assign the parameter to a member variable.
- Assigning the parameter by using the keyword this->
- Use a single line comment to comment the cout line form this constructor.
- Methods
- Define a method (function) in the class.
- Add a method to your Bus class called printDriverName. This method is a void method. Make this method output the text Drivers name is followed by the value of the name instance variable.
- Run and call this method in the main function by using dot (.) operator.
- Get and Set Methods (getter and setter or it can also be called mutators and accessors)
- Add getDriverName and setDriverName methods to your class.
- The getDriverName method will return the variable name.
- The setDriverName will pass the parameter value to the variable name.
- Call those two methods in the main function. For the setDriverName method, please pass a different name other than your name and last name into the methods.
- And call the printDriverName() function again
- Now you are done, yeah!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
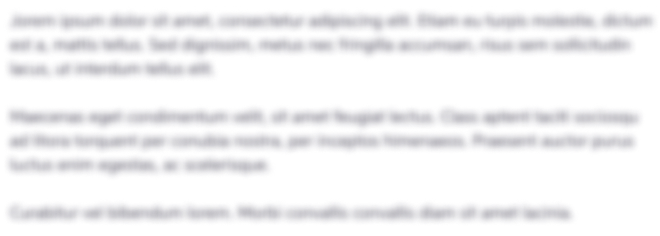
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started