Question
Create a java class FileHandler in a program that reads and writes grades data from a text file on disk and performs some simple calculations
Create a java class FileHandler in a program that reads and writes grades data from a text file on disk and performs some simple calculations on that data in the following ways: Use a looping (repetition) construct that allows the user to enter as much grade data as possible with a sentinel to end the loop and write the data to disk and close the file.
Use a second looping construct that allows the user to open and read a disk file and retrieve grades from it.
Part 2
Use the class you created in Part 1 and write grades into a text file and close the file. Open the file using your class functions and read the grade data into a simple data structure of your choosing and then calculate the GPA and print of the data in a nicely formatted command line display. Use the following grade/GPA allocations:
A = 4.0
A- = 3.7
B+ = 3.3
B = 3.0
B- = 2.7
C+ = 2.3
C = 2.0
C- = 1.7
D+ = 1.3
D = 1.0
D- = 0.7
F = 0.0
Part 3
Design a JavaFX GUI application that:
Uses the class FileHandler class internally to input the grades data from GUI into some data structure in the application class which will then be sent to disk.
Display the grade data read from a file and display it in a JavaFX GUI control element of your choosing.
Explain your choice of display control element i.e. what it is and how it works Remember to create an OK button to finally terminate the GUI application.
Lab Process
In this lab, the developer should do the following activities:
Part 1
Create a java class FileHandler that has a parameter constructor that takes 2 strings a read from and write to filename.
Create a private data member that is a file reading scanner object reference and a file writing Formatter object reference file handle which will be used to read and write text files from disk.
Create private data members that are strings representing names of read and write text files.
Create 2 separate public methods on the FileHandler class one to open a file to read returning Boolean true on success and one for opening a file to write returning Boolean true on success.
Create 2 separate public methods on the FileHandler class, one to write grades to a file given an ArrayList of strings representing grades returning Boolean true on success. The second method should read grades from a file and populate an ArrayList passed to it of strings representing grades. It should also return Boolean true on success.
Write a main function on your FileHandler class and test its functionality by writing test code to check the functionality of the functions you have added to the class.
Part 2
Create a main function and a test program to test your FileHandler class by creating this object. Open a file and write some example grades to it. Close the file. Then, open the file and try to read them back and see if the same data is retrieved from the file as was initially written to it. Also, remember to inspect the text file containing the grades to see if the correct grades are indeed in the file.
Part 3
Create a JavaFX project which builds a GUI called GradeIO which creates a basic GUI AnchorPane container. Make sure to see that the project has a .fxml file and controller class separate from the main app. Double click on the projects .fxml file and it should open Scene Builder for that project.
Add a private reference of type ArrayList of strings (named for example m_ gradesFromFile) to hold any grades read from file which should be instantiated using operator new in the initialize method of the controller class.
Add a private reference of type FileHandler which should be instantiated using operator new in the initialize method of the controller class to be then used for file IO.
Scene Builder Activities
Add a label that will display the GPA given a chosen grade named GPALabel.
Add a textbox control that will take input for grade filename to read place a default string filename in it via the properties tab in Scene Builder.
Add a button and label it Read Grades File. Add an event handler method named handleReadGrades. Implement the code to use the filehandler object to open a file named (given in the filename textbox you created above) and to fill the private ArrayList of strings container to store the read grades.
As an example for students as a control that can be chosen to display grades the demo has used a combo box control which is loaded with grades once the grade file is read. Then each selection on the combo box is a grade that was read from the file. Any particular selection of the combo box can then be converted into a grade by clicking the Calculate GPA button. It was chosen as it easily allows the program to know which selection (i.e. grade) the user has selected. (other options are radio buttons as another example)
Add a button and label it Calculate GPA. Add an event handler method named handleCalcGPA. Write a private method on the JavaFX controller class called convertGradeToGPA which when given a grade (which may be a selected grade from the grade display control above) returns the equivalent GPA in string form. This string can then be used to display into the GPALabel. In this handler, you will need to get the selected grade from the GUIs Grade control (e.g. combo box), call convertGradeToGPA, and display the returned string from that method in that label.
Add a button and label it OK. Add an event handler method named handleOKPressed. Implement the code to close the stage component of the application.
Add a button and label it Minimize. Do the research and find the API to minimize a JavaFX application.
please help me to do this when I run this program in IntelJ it gives me error about import file and other so please help me
Step by Step Solution
There are 3 Steps involved in it
Step: 1
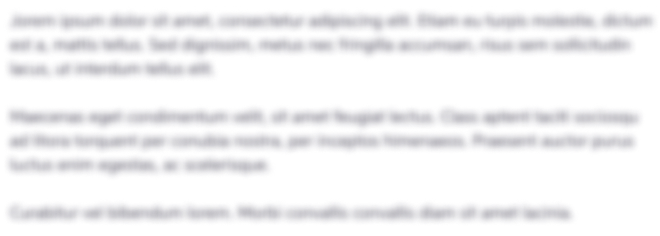
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started