Question
Create a Java program, RailcarSwitcher, that simulates a railroad switching station. -------------------------- -------------------------- Input --> / Output --> | ----------------------- ----------------------- | | |
Create a Java program, RailcarSwitcher, that simulates a railroad switching station.
-------------------------- --------------------------
Input --> \ / Output -->
|
----------------------- -----------------------
| |
| Y |
| a |
| r |
| d |
| |
Railroad engineers often have to switch cars on the trains that they manage. Because
of the lack of maneuverability of these railcars (and the fact that they can only move on
tracks), getting the correct cars off of one train and on to another can be problematic.
Using the above diagram as a guide, cars can enter from the right and are sent to the
output on the left by routing through the yard "stack". Each car can be brought into the
stack and removed at any time.
The RailcarSwitcher program will aid railroad engineers in determining the ordering of
outgoing cars. Design the program with the following specifications:
1.
The RailcarSwitcher class should have the following member variables:
String inSequence
: The railcars to evaluate as the incoming sequence.
String outSequence
: The target sequence that the program will attempt
to build.
final int maxCars = 10
: Largest number of cars that the entire program
can accept at any one time.
inSequence and outSequence should be obtained from the user.
2.
A queue to represent the original car arrangement.
3.
A stack to represent the yard.
4.
A second queue to represent the output arrangement.
5.
push
,
pop
, and
peek
methods to add, remove, and scan items from the stack.
6.
The program should work through the input queue until either the output
sequence is created in its entirety or the program cannot continue to build the
desired output.
Example:
An input of:
1 2 3 4
can be mapped to an output of
4 3 2 1
using the sequence:
push push push push pop pop pop pop
But: An input of:
1 2 3 4
cannot be mapped to an output of
4 2 3 1
The proceeding examples would produce the output:
Attempting to map the input
1 2 3 4
to the output
4 3 2
1
...
The sequence of moves are:
push push push push pop pop pop
pop
Attempting to map the input
1 2 3 4
to the output
4 2 3
1
...
The sequence of moves are:
push push push push pop
The input cannot be mapped to the output.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
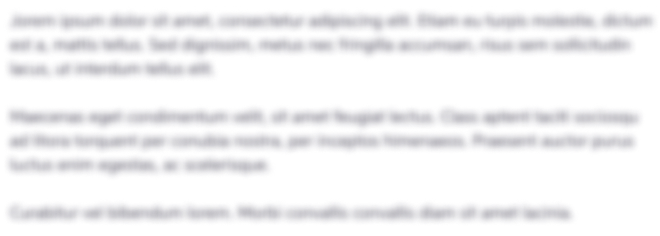
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started