Question
Create a java program which will recursively perform the following two actions: 1. Print all possible subsets using the first n integers from the set
Create a java program which will recursively perform the following two actions:
1. Print all possible subsets using the first n integers from the set of integers contained in the array int a[].
For example if int a[] = {1, 2, 3, 4, 5, 6, 7} and subsets(a, 3) was called, the following would be printed: 3, 2, 23, 1, 13, 12, 123
Use these method signatures:
static void subsets(int a[], int n) {
subsets("", a, n);
}
static void subsets(String s, int a[], int n) {
}
2. Print all possible permutations of String s.
For example for the string s = "abc", the permutations are abc, acb, bac, bca, cab, cba.
Assume S has no repeating characters contained in it.
Use these method signatures:
static void permutations(String S) {
permutations(S, 0);
}
static void permutations(String s, int position) {
}
My code so far, all that is needed now is the correct recursive code to consistently create the specific pattern for the subset and permutation methods:
import java.util.Scanner; public class JJL9 { static void subsets(int a[], int n) { subsets("", a, n); }
/*Method to recursively create the specific subset pattern whereas if the array is a[] = {1,2,3,4,5,6,7} and subsets(a, 3) called, the subset would be: 3, 2, 23, 1, 13, 12, 123*/
static void subsets(String s, int a[], int n) { }
static void permutations(String s) { permutations(s, 0); }
/*Method to recursively create the specific permutation pattern whereas if the string is s = "abc", the permutations would be: abc, acb, bac, bca, cab, cba*/
static void permutations(String s, int position) { }
public static void main(String[] args) { Scanner in = new Scanner(System.in); int a[] = {1, 2, 3, 4, 5, 6, 7}; String choice; do { System.out.println("1.) Check subsets for array "); System.out.println("2.) Check permutations for array "); System.out.println("3.) Exit "); System.out.print(" Choose an Option: "); choice = in.next(); switch(choice.charAt(0)) { case '1': //Case to print out the specific subset method subsets(a, 3); break; case '2': //Case to print the specific permutation method permutations("abc"); break; case '3': //Exit Code Case System.out.println(" Goodbye! "); break; default: System.out.println(" Invalid Input! Try Again! "); } }while(choice.charAt(0) != '3'); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
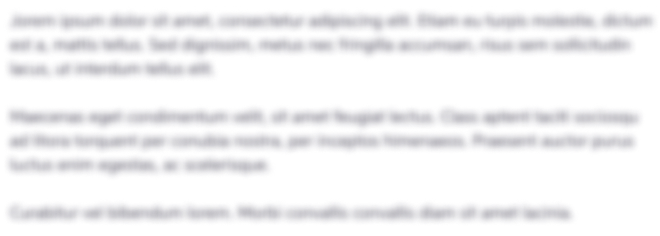
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started