Question
Create a main method and use these input files to test that you are able to input the Undergraduates, put them into an ArrayList, print
Create a main method and use these input files to test that you are able to input the Undergraduates, put them into an ArrayList, print out the list. Repeat for Graduate, Faculty, Staff. Do it all in ONE main. Then- Add a compareTo to each class (and any other code you need) so you can sort all your ArrayLists and print out the results. Your final output should be all the Undergraduates (sorted), all the Graduates (sorted), all the Faculty(sorted), and all the Staff (sorted). To sort: Sort undergrads and grads according to ID. Sort Faculty according to Department and Staff according to Salary.
Here are the files you need to implement in the main:
undergrad.in:
3333 3 John J. Porter Jr. 2846 1 Eliza Doone 3536 2 Steven Sutter 6453 4 Moses Baker 7733 2 Gerald Brooks
graduate.in:
4456 Masters no Julie Strange 9953 Doctorate yes Amy Burrell Fuller 6756 Masters yes John Doe 7867 Masters no Howard Long III 3322 Doctorate no Jimmy Peters
faculty.in:
44000.00 1999 3478 Music Associate_Professor Anna Key 67000.00 2010 4510 Philosophy Professor John Smith 56000.00 2011 3402 Psychology Assistant_Professor Luke Hope 46000.77 2014 3567 Art Instructor Elizabeth Moony 67000.50 2015 6666 Science Assistant_Professor Kim Nagle
staff.in:
34567.50 2010 5674 Facilities 3 John Joe Jr. 31478.49 2014 4457 Media_Services 2 Alice Foreman 45000.00 2015 6789 IT 5 Kate Space 44000.00 2016 1234 CIDAT 18 Wesley Winchester III
Here is what I have:
Person.java
public class Person { protected String name; public Person(){ name = null; } public Person(String name){ this.name=name; } public String getName(){ return name; } public void setName(String Name){ this.name=name; } public boolean hasSameName(Person b){ if (this.name == (b.name)) return true; else return false; } public void writeOutput(){ System.out.print("Name: "+ name); } }
Student.java
public class Student extends Person { protected int studentNumber; public Student(){ super(); studentNumber= 0; } public Student(String name, int studentNumber){ super(name); this.studentNumber = studentNumber; } public int getStudentNumber(){ return studentNumber; } public void setStudentNumber(int studentNumber){ this.studentNumber=studentNumber; } public boolean equals(Student b){ if (this.studentNumber == b.studentNumber) return true; else return false; } public void writeOutput(){ System.out.println("Name: "+name + " Student Number: "+ studentNumber); } }
Undergraduate.java
public class Undergraduate extends Student { protected int level; public Undergraduate(){ super(); level = 0; } public Undergraduate(int studentNumber, int level, String name){ super(name, studentNumber); this.level = level; } public int getLevel(){ return level; } public void setLevel(int level){ this.level=level; } public boolean equals(Undergraduate b){ if (this.studentNumber == b.studentNumber) return true; else return false; } public void writeOutput(){ super.writeOutput(); System.out.println(" Student Level: "+ level); } public String toString(){ return " Name: "+name+ " Student Number: "+studentNumber+ " Student Level: "+ level; } }
Employee.java
public class Employee extends Person{ protected double salary; protected int yearHire; protected int emplID; protected String dept; public Employee(){ super(); salary = 0.0; yearHire = 0; emplID = 0; dept = null; } public Employee(String name, double salary, int yearHire, int emplID, String dept){ super(name); this.salary = salary; this.yearHire = yearHire; this.emplID = emplID; this.dept = dept; }
public double getSalary() { return salary; }
public void setSalary(double salary) { this.salary = salary; } public int getYearHire() { return yearHire; } public void setYearHire(int yearHire) { this.yearHire = yearHire; }
public int getEmplID() { return emplID; }
public void setEmplID(int emplID) { this.emplID = emplID; }
public String getDept() { return dept; } public void setDept(String dept) { this.dept = dept; } public boolean equals (Employee b){ if (this.emplID == b.emplID) return true; else return false; } public void writeOutput(){ super.writeOutput(); System.out.println(" Salary: "+ salary + " Year Hired: "+ yearHire + " Employee ID: "+ emplID + " Department: "+ dept); } }
Graduate.java
public class Graduate extends Student{ private String degree;
public Graduate(){ super(); degree=" "; } public Graduate(String initialDegree, String initialName, int initialStudentNumber){ super(initialName, initialStudentNumber); degree=initialDegree; } public boolean thesis(){ return false; }
public String getDegree() { return degree; }
public void setDegree(String degree) { this.degree = degree; } public void writeOutput(){ super.WriteOutput(); System.out.println("Degree: "+ degree); } public boolean equals(Graduate otherGraduate){ return this.degree == otherGraduate.degree; } }
Faculty.java
public class Faculty extends Employee { private String title; public Faculty(){ super(); title= " "; } public Faculty(String initialName, String initialTitle){ super(initialName); title=initialTitle; } public String getTitle() { return title; }
public void setTitle(String title) { this.title = title; } public void Reset(String newName){ setName(newName); } public void WriteOutput(){ super.WriteOutput(); System.out.println("Title: "+title); } public boolean Equals(Faculty other){ return this.hasSameName(other)&&(this.title==other.title); } }
Staff.java
public class Staff extends Employee { int payGrade; public Staff(){ super(); payGrade=0; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
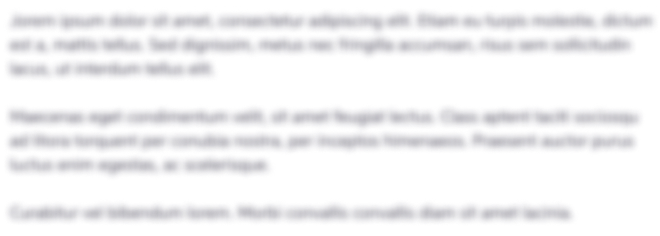
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started