Question
Create a method called countingsort() and add it to the following sorting.java program that reads 4 integers n, a, b and k from any input
Create a method called countingsort() and add it to the following sorting.java program that reads 4 integers n, a, b and k from any input file, generates n random integers and stores them in an arr[], prints arr[] formatted with k numbers per line, before and after count sorting.
import java.io.*;
import java.util.*;
// program reads 4 integers n, a, b and k from anyinputfile, generates n random
// integers and stores them in an arr[], prints arr[] formatted k numbers per line
public class sorting{
int freq[], arr[], a, b, n, k, range;
PrintStream prt = System.out
// This is the class constructor
sorting(){
prt.print("\tThis Java program reads 4 integers n, a, b and k" +
" \tfrom anyinputfile, generates n random integers and stores them in an arr[] and \t" +
"Prints arr[] formatted k numbers per line," +
"before and after count sorting." +
" \t\tTo compile: javac sorting.java " +
" \t\tTo execute: java sorting < anyinputfile");
try{
// open file inf
Scanner inf = new Scanner(System.in)
n = inf.nextInt(); //read n, no. of data
a = inf.nextInt(); //read a, lower range
b = inf.nextInt(); //read b, upper range
k = inf.nextInt(); //read k, per line
// close file inf
inf.close();
} catch (Exception e){
prt.printf(" Oops! Read Exception: %s" , e);
}
range = b - a + 1;
arr = new int[n];
freq = new int[range];
prt.printf(" \tINPUT: n = %3d, a = %4d, b = %4d, k = %4d", n, a, b, k);
}
private void generate(){
int i;
Random rand = new Random();
for (i = 0; i < n ; i ++)
arr[i] = rand.nextInt(range) + a;
}
//Print arr[] formatted, k elements per line
private void printarr(){
int j;
for (j = 0; j < n ; j ++){
prt.printf("%4d ", arr[j]);
if ((j+1) % k == 0)
prt.printf(" \t");
}
}
// main program
public static void main(String args[]) throws Exception{
//create an instance of a class
sorting h = new sorting();
//call generate method
h.generate();
System.out.printf(" \t arr[] contents before count sorting: \t");
//call printarr method
h.printarr();
//call countingsort method
g.countingsort();
System.out.printf(" \t arr[] contents after count sorting: \t");
//call printarr method
h.printarr();
System.out.printf(" \tAuthor: Date: " +
java.time.LocalDate.now());
}
}
//please help me I made a code but it doesn't sort properly
Step by Step Solution
There are 3 Steps involved in it
Step: 1
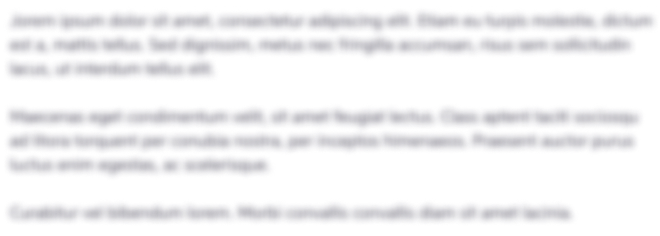
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started