Question
Create a new Driver class with a main method. In this method, do the following: 1. Create an instance of an ArrayStack and a LinkedStack
Create a new Driver class with a main method. In this method, do the following:
1. Create an instance of an ArrayStack and a LinkedStack class.
2. Push the following int's onto the two stacks: (1,7,3,4,9,2)
3. Pop off all the elements from the stacks, displaying each int as it's removed
I have the code for this (see below), but I need assistance in creating the println method to print the results to the screen. I have three separate classes, one for ArrayStack, LinkedStack, and Driver
public class Driver {
public static void main(String[] args) {
ArrayStack stack = new ArrayStack(10);
stack.push(1);
stack.push(7);
stack.push(3);
stack.push(4);
stack.push(9);
stack.push(2);
LinkedStack stack1 = new LinkedStack();
stack1.push(1);
stack1.push(7);
stack1.push(3);
stack1.push(4);
stack1.push(9);
stack1.push(2);
}
}
public class ArrayStack {
private int[] arr;
private int n;
private int capacity;
public ArrayStack(int cap) {
capacity = cap;
n = 0;
arr = new int[cap];
}
public int size() {
return n;
}
public void push(int item) {
if(n == capacity)
System.out.println("Stack is full");
else {
arr[n] = item;
n++;
}
}
public int pop() {
int data = -999;
if(n == 0)
System.out.println("Stack is full");
else {
data = arr[n-1];
n--;
}
return data;
}
}
public class LinkedStack {
class Node {
int data;
Node next;
Node(int item) {
data = item;
}
}
private Node top;
private int n;
public LinkedStack() {
top = null;
n = 0;
}
public void push(int item) {
Node newNode = new Node(item);
newNode.next = top;
top = newNode;
n++;
}
public int size() {
return n;
}
public int pop() {
if(n == 0) {
return -999;
}
int data = top.data;
top = top.next;
n--;
return data;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
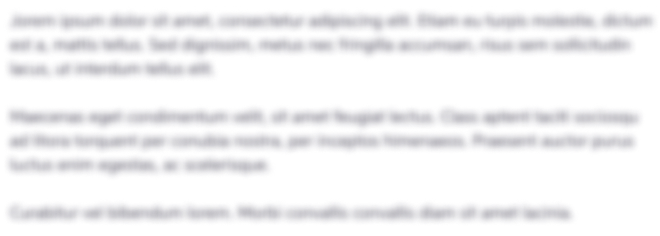
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started