Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Create a new project in BlueJ for Lab 8. If your Song class from Lab 4 is fully functional, copy it into the project. (You
- Create a new project in BlueJ for Lab 8.
- If your Song class from Lab 4 is fully functional, copy it into the project. (You can drag a file from File Explorer onto the BlueJ project window.) Otherwise, use the instructor's Song class:
- Create a new class using the "New Class..." button and name it Song.
- Open the Song class to edit the source code. Select and delete all the source code so that the file is empty.
- Copy in the starting source code found here: Lab 8: Song Starting Source Code. (This link will not work for you until you have received feedback on Lab 4. Email the instructor if you think you should be able to access it but can't.)
- Create a Playlist class and copy in the starting source code found here: Lab 8: Playlist Starting Source Code.
- For this lab, you will be editing the Playlist class. You should not change the Song class for this lab.
- In Playlist, update the header comment block and document your changes as you work. Use the javadoc tags @param and @return in method header comments as appropriate.
- As usual, adhere to Java style guidelines as described in Appendix J.
- Complete the TODOs in the Playlist code, modifying and implementing methods as described. Be sure to follow the specifications as given in the TODO comments.
- Before submitting your project, delete the TODO comments. TODOs represent a task that still needs to be done, and when you complete a TODO it should be removed. There should not be any TODOs in your submitted project (unless you didn't complete the project).
- As usual, you should be testing as you go to make sure everything works!
- The SongTest class given in Lab 4 should still work on this project - you can use it to verify that you didn't break any of the previous functionality.
- To test your functionality for this lab, use the PlaylistTest class: PlaylistTest.java.
- When you have completed the assignment, create a jar file of your project.
- While the project is open in BlueJ, choose Project->Create Jar File...
- Be sure the "Include source" box is checked.
Song Class Starting Source Code:
/** * A song, such as one in a music store which can be rated * * * @author Cara Tang * @version 2020.09.06 */ public class Song { private String title; private String artist; private int lengthInSeconds; private int rating; // valid ratings are 1-4 or 0 meaning not rated /** * Create a song with the given title, artist, length, and rating * @param songTitle the song title * @param songArtist the song artist * @param songLength the length of the song in seconds * @param songRating the song's rating */ public Song(String songTitle, String songArtist, int songLength, int songRating) { title = songTitle; artist = songArtist; lengthInSeconds = songLength; setRating(songRating); } /** * Create an unrated song with the given title, artist, and length * @param songTitle the song title * @param songArtist the song artist * @param songLength the length of the song in seconds */ public Song(String songTitle, String songArtist, int songLength) { title = songTitle; artist = songArtist; lengthInSeconds = songLength; rating = 0; } /** * @return the song title */ public String getTitle() { return title; } /** * @return the song artist */ public String getArtist() { return artist; } /** * @return the song length (in seconds) */ public int getLengthInSeconds() { return lengthInSeconds; } /** * @return a string giving the song length in minutes (m) and seconds (s). * Example: If lengthInSeconds is 150, the string returned is "2m 30s" */ public String getLengthAsString() { int minutes = lengthInSeconds / 60; int seconds = lengthInSeconds % 60; return minutes + "m " + seconds + "s"; } /** * Set the length of this song to the value given. * The length must be greater than 0. * @param newLength new length (in seconds) for this song */ public void setLengthInSeconds(int newLength) { if (newLength > 0) { lengthInSeconds = newLength; } else { System.out.println("Error: Length must be greater than 0"); } } /** * @return true if this song has not been rated, false otherwise */ public boolean isUnrated() { return rating == 0; } /** * @return the song rating */ public int getRating() { return rating; } /** * Update this song's rating to the one given * @param newRating new rating for this song */ public void setRating(int newRating) { if (newRating >= 1 && newRating <= 4) { rating = newRating; } else { System.out.println("Error: Rating must be in the range 1 to 4"); } } /** * Reset the rating of this song to not rated */ public void resetRating() { rating = 0; } /** * Increase the rating of this song by 1 */ public void increaseRating() { if (rating < 4) { rating = rating + 1; } } /** * Decrease the rating of this song by 1 */ public void decreaseRating() { if (rating > 1) { rating = rating - 1; } } /** * Print information on this song */ public void printSongInfo() { System.out.println("---------------------------------"); System.out.println("Song Title: " + title); System.out.println("Artist: " + artist); System.out.println("Length: " + getLengthAsString()); if (isUnrated()) { System.out.println("Rating: (not rated)"); } else { System.out.println("Rating: " + rating); } System.out.println("---------------------------------"); } }
Playlist Class Starting Source Code
import java.util.ArrayList; /** * A playlist of songs * * Modifications: * CT: Create Playlist class with a list of songs and basic methods to add, clear, and print songs * * @author Cara Tang * @version 2020.09.07 */ public class Playlist { private String playlistName; private ArrayListplaylist; /** * Create an empty playlist with the given name * @param name the name for this playlist */ public Playlist(String name) { playlistName = name; playlist = new ArrayList (); } /** * Populate the playlist with a few songs. * Use this method to make testing easier. After creating a Playlist object, * call this method to populate some songs, and then test your methods. */ public void populateSongs() { addSong("The Twist", "Chubby Checker", 154); addSong(new Song("Bohemian Rhapsody", "Queen", 355, 3)); // TODO: ------------------------ 1 -------------------------- // TODO: Add a few more songs. } /** * @return the playlist name */ public String getPlaylistName() { return playlistName; } /** * Add the given song to the end of this playlist * @param aSong a song to add */ public void addSong(Song aSong) { playlist.add(aSong); } /** * Create an unrated song with the given title, artist, and length, and * add it to the end of this playlist. * @param title title of the song * @param artist the song's artiest * @param length length of the song in seconds */ public void addSong(String title, String artist, int length) { playlist.add(new Song(title, artist, length)); } /** * @return the number of songs in this playlist */ public int getNumberOfSongs() { return playlist.size(); } /** * Remove all the songs from this playlist */ public void clearPlaylist() { playlist.clear(); } /** * Print the playlist */ public void printPlaylist() { System.out.println("============= Playlist: " + playlistName + " ============="); if (getNumberOfSongs() == 0) { System.out.println("No songs in this playlist"); } else { for (Song currentSong : playlist) { currentSong.printSongInfo(); } } System.out.println("============================================="); } /** * Find a song based on its title * @param title the title of the song to search for * @return the first song with the given title in this playlist * or null if there is no song with that title in this playlist */ public Song findSong(String title) { // TODO: ------------------------ 2 -------------------------- // TODO: Implement according to the header comment. // TODO: Do not change the method signature that is given. // TODO: Hint: One approach is to use a for-each to loop over each song in the list. // TODO: Inside the loop check if the song title matches and if so, return the song. // TODO: If the loop completes without returning, the song is not in the list and // TODO: you can return null. return null; } // TODO: ------------------------ 3 -------------------------- // TODO: Write a method called removeSong that takes a String parameter, // TODO: and removes the first song with that title in this playlist. // TODO: Hint: ArrayList has a remove method that takes an object that is the element // TOOD: to remove. You can call findSong to find the song to remove, and pass it to remove. // TODO: ------------------------ 4 -------------------------- // TODO: Write a method called getSongsByArtist that takes a String parameter // TODO: and returns a list of songs by the given artist. // TODO: If there are no songs by that artist, return an empty list (not null). // TODO: Do not print anything in this method. // TODO: Hint: The structure of this method will be the same as the getUnsold method // TODO: from the Auction project. // TODO: ------------------------ 5 -------------------------- // TODO: Write a method called getNumSongsWithRating that takes an int parameter // TODO: representing a rating, and returns the number of songs in the playlist // TODO: that have the given rating. // TODO: Example: If two songs have rating 3, then the call // TODO: getNumSongsWithRating(3) will return 2. // OPTIONAL TODO: ------------------------ 6 -------------------------- // OPTIONAL TODO: This TODO is optional. // OPTIONAL TODO: Write a method called getMaxRatingOfASong (no parameters) that // OPTIONAL TODO: returns the maximum rating of a song in the playlist. // OPTIONAL TODO: Example: If the playlist has 3 songs, one with rating 2 and two unrated, // OPTIONAL TODO: then the max rating is 2. // OPTIONAL TODO: If there are no songs in the playlist, return 0 // TODO: ------------------------ 7 -------------------------- // TODO: Write a method called printPlaylistSummaryStats (no parameters, no return) // TODO: that prints the name of the playlist, the number of songs in the playlist, // TODO: the number of songs with each rating, and the number of unrated songs. // TODO: If you implemented getMaxRatingOfASong, print the max rating as well. // TODO: Sample output: // ======== SUMMARY STATS for Playlist: Rock ======== // # of songs: 3 // # rated 4: 0 // # rated 3: 0 // # rated 2: 1 // # rated 1: 0 // # unrated: 2 // Max rating: 2 // ================================================== }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
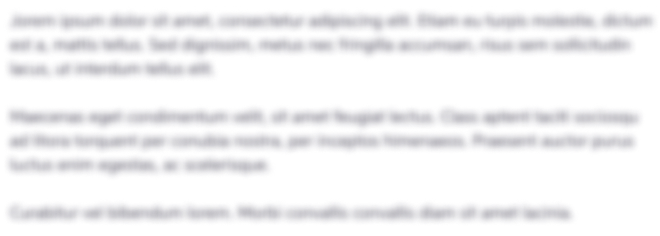
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started