Question
Create a procedure named FindThrees that returns 1 if an array has three consecutive values of 3 somewhere in the array. Otherwise, return 0. The
Create a procedure named FindThrees that returns 1 if an array has three consecutive values of 3 somewhere in the array. Otherwise, return 0. The procedures input parameter list contains a pointer to the array and the arrays size. Use the PROC directive with a parameter list when declaring the procedure. Preserve all registers (except EAX) that are modified by the procedure. Write a test program that calls FindThrees several times with different arrays.
Here is what I have so far,
;Create procedure name FindThrees INCLUDE Irvine32.inc ;FindThrees PROTO, ;pointerArr: PTR DWORD, ;ArrLeng:DWORD
.data ;strContains BYTE "The array has three consecutive 3.",0 ;strNotContain BYTE "The array does not contain three consecutive 3.",0 ;arrOne BYTE 1, 2, 3, 2 ;arrTwo BYTE 3, 3, 5, 7, 3 ;arrThree BYTE 4, 3, 3, 3, 1, 8
.code main PROC
; calls the procedures call Clrscr ; clears the screen ; find if the array arrOne contains three consecutive 3 INVOKE FindThrees, ADDR arrOne, LENGTHOF arrOne .IF eax == 1 mov edx,OFFSET strContains call WriteString ; writes strContains call Crlf .ELSE mov edx,OFFSET strNotContain call WriteString ; writes strNotContain call Crlf .ENDIF
; find if the array arrTwo contains three consecutive 3 INVOKE FindThrees, ADDR arrTwo, LENGTHOF arrTwo .IF eax == 1 mov edx,OFFSET strContains call WriteString ; writes strContains call Crlf .ELSE mov edx,OFFSET strNotContain call WriteString ; writes strNotContain call Crlf .ENDIF
; find if the array arrThree contains three consecutive 3 INVOKE FindThrees, ADDR arrThree, LENGTHOF arrThree .IF eax == 1 mov edx,OFFSET strContains call WriteString ; writes strContains call Crlf .ELSE mov edx,OFFSET strNotContain call WriteString ; writes strNotContain call Crlf .ENDIF exit main ENDP FindThrees PROC USES esi ecx, pointerArr: PTR DWORD, ; points the array ArrLeng: DWORD ; the length of array ; finds if the array contains three consecutive 3 as its values ; Receives: pointer to array and the length of array ; Returns: EAX = 1 (true) or 0 (false) mov eax,0 ; initialize EAX = 0 mov esi,pointerArr ; ESI is the pointer to array mov ecx,ArrLeng sub ecx,2 ; as next 2 elements were observed L1: .IF [esi] == 3 .IF [esi+1] == 3 .IF [esi+2] == 3 mov eax,1 ; set EAX = 1 jmp L2 .ENDIF .ENDIF .ENDIF inc esi ;Increment ESI loop L1 L2: ret ; returns EAX FindThrees ENDP
END main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
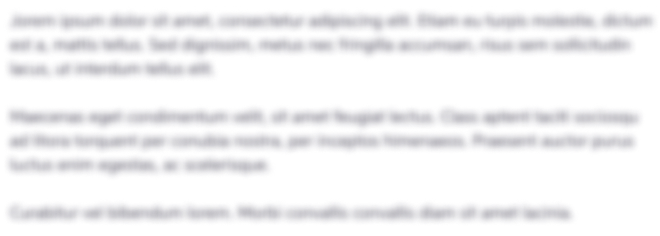
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started