Question
Create a program fraction.cpp that add and subracts two fractions and write the answer in reduced form. The program should contain header file fraction.h and
Create a program "fraction.cpp" that add and subracts two fractions and write the answer in reduced form. The program should contain header file "fraction.h" and the constructor "Fraction::Fraction(int a, int b)". ALso it should work with the provided main programs "calculator.cpp" and "useFraction.cpp" which should not be modified. The >> and << operators must be overloaded and used to read and write fractions. Member functions getNum() and getDen () should be implemented, returning the (reduced) numerator and denominator of the fraction. Examples of input/output are 1/2+1/3 and 4/6 + 4/6 = 5/6 and 4/3.
Calculator.cpp
//
// calculator.cpp
//
// DO NOT MODIFY THIS FILE
//
#include "Fraction.h"
#include
#include
using namespace std;
int main()
{
Fraction x,y;
char op;
try
{
cin >> x;
cin >> op;
while ( cin && ( op == '+' || op == '-' ) )
{
cin >> y;
if ( op == '+' )
x = x + y;
else
x = x - y;
cin >> op;
}
cout << x << endl;
}
catch ( invalid_argument& e )
{
cout << "Error: " << e.what() << endl;
}
}
useFraction.cpp
//
// useFraction.cpp
//
// DO NOT MODIFY THIS FILE
//
#include "Fraction.h"
#include
using namespace std;
void print_fraction(const Fraction& f)
{
cout << "print_fraction: " << f.getNum() << "/" << f.getDen() << endl;
}
int main()
{
Fraction x(2,3);
Fraction y(6,-2);
cout << x << endl;
cout << y << endl;
cin >> y;
cout << y << endl;
print_fraction(y);
Fraction z = x + y;
cout << x << " + " << y << " = " << z << endl;
}
just in case if its helpful here is the code in C to find the reduced_fraction. make necessary changes so that it can be used in C++
reduce_fraction.c
/* find gcd of two numbers */
int find_gcd (int n1, int n2)
{
int gcd, remainder;
/* Euclid's algorithm */
remainder = n1 % n2;
while ( remainder != 0 )
{
n1 = n2;
n2 = remainder;
remainder = n1 % n2;
}
gcd = n2;
return gcd;
}
/* reduce a fraction */
void reduce_fraction(int *nump, int *denomp)
{
int gcd;
gcd = find_gcd(*nump, *denomp);
*nump = *nump / gcd;
*denomp = *denomp / gcd;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
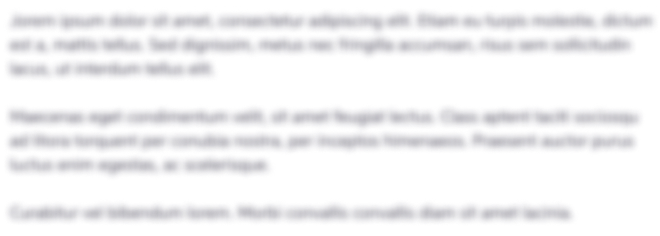
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started