Question
/* Create a program that prints a class roll sheet in alphabetical order. The user inputs the students' first name and last names (separately!), and
/* Create a program that prints a class roll sheet in alphabetical order. The user inputs the students' first name and last names (separately!), and presses enter on the first name when done. The program prints out the roster like this...
HATFIELD, HEIDI KAISER, RUSSELL LIPSHUTZ, HOWARD PENKERT, DAWN WRIGHT, ELIZABETH
Before starting, carefully study sort_str(), mod_str(), and format(). Make a copy of sort_str() and rename it roll(). Also, change stsrt(). Compile and make sure it works from main(). Change limit[] to represent first name. The input should work for 30 students and first name should be 15 characters. Change prompts as needed. Compile and test. Make changes to convert the first name to all upper case using a function from mod_str(). Add another array and get input for last name which will also be an array of 30 with 15 characters. Combine last and first into an third array. You may use sprintf() for this one. There are other ways.
*/
References:
################################################################
/* sort_str.c -- reads in strings and sorts them */
#include
#include
#define SIZE 81 /* string length limit, including \0 */
#define LIM 20 /* maximum number of lines to be read */
#define HALT "" /* null string to stop input */
void stsrt(char *strings[], int num);/* string-sort function */
char * s_gets(char * st, int n);
void sort_str(void)
{
char input[LIM][SIZE]; /* array to store input */
char *ptstr[LIM]; /* array of pointer variables */
int ct = 0; /* input count */
int k; /* output count */
printf("Input up to %d lines, and I will sort them. ",LIM);
printf("To stop, press the Enter key at a line's start. ");
while (ct < LIM && s_gets(input[ct], SIZE) != NULL
&& input[ct][0] != '\0')
{
ptstr[ct] = input[ct]; /* set ptrs to strings */
ct++;
}
stsrt(ptstr, ct); /* string sorter */
puts(" Here's the sorted list: ");
for (k = 0; k < ct; k++)
puts(ptstr[k]) ; /* sorted pointers */
}
/* string-pointer-sorting function */
void stsrt(char *strings[], int num)
{
char *temp;
int top, seek;
for (top = 0; top < num-1; top++)
for (seek = top + 1; seek < num; seek++)
if (strcmp(strings[top],strings[seek]) > 0)
{
temp = strings[top];
strings[top] = strings[seek];
strings[seek] = temp;
}
}
char * s_gets(char * st, int n)
{
char * ret_val;
int i = 0;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
while (st[i] != ' ' && st[i] != '\0')
i++;
if (st[i] == ' ')
st[i] = '\0';
else // must have words[i] == '\0'
while (getchar() != ' ')
continue;
}
return ret_val;
}
#####################################################################################
/* mod_str.c -- modifies a string */
#include
#include
#include
#define LIMIT 81
void ToUpper(char *);
int PunctCount(const char *);
void mod_str(void)
{
char line[LIMIT];
char * find;
puts("Please enter a line:");
fgets(line, LIMIT, stdin);
find = strchr(line, ' '); // look for newline
if (find) // if the address is not NULL,
*find = '\0'; // place a null character there
ToUpper(line);
puts(line);
printf("That line has %d punctuation characters. ",
PunctCount(line));
}
void ToUpper(char * str)
{
while (*str)
{
*str = toupper(*str);
str++;
}
}
int PunctCount(const char * str)
{
int ct = 0;
while (*str)
{
if (ispunct(*str))
ct++;
str++;
}
return ct;
}
#####################################################################################
/* format.c -- format a string */
#include
#define MAX 20
char * s_gets(char * st, int n);
void format(void)
{
char first[MAX];
char last[MAX];
char formal[2 * MAX + 10];
double prize;
puts("Enter your first name:");
s_gets(first, MAX);
puts("Enter your last name:");
s_gets(last, MAX);
puts("Enter your prize money:");
scanf("%lf", &prize);
sprintf(formal, "%s, %-19s: $%6.2f ", last, first, prize);
puts(formal);
}
char * s_gets(char * st, int n)
{
char * ret_val;
int i = 0;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
while (st[i] != ' ' && st[i] != '\0')
i++;
if (st[i] == ' ')
st[i] = '\0';
else // must have words[i] == '\0'
while (getchar() != ' ')
continue;
}
return ret_val;
}
#####################################################################################
/* sprintf example */
#include
int main ()
{
char buffer [50];
int n, a=5, b=3;
n=sprintf (buffer, "%d plus %d is %d", a, b, a+b);
printf ("[%s] is a string %d chars long ",buffer,n);
return 0;
}
#####################################################################################
Step by Step Solution
There are 3 Steps involved in it
Step: 1
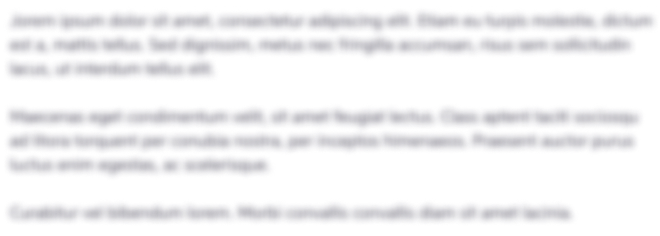
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started