Question
Create a program that will report gross salary amounts and other compensation. This program will take the classes detailed below and build upon them to
Create a program that will report gross salary amounts and other compensation. This program will take the classes detailed below and build upon them to run the output.
There are three types of employees in the firm: Programmers, Lawyers, Accountants
Here's the output of the program:
Employee List Programmer: Mr. Ima Nerd Programmer: Mrs. Ima Nerd Accountant: Mr. Bean Counter Accountant: Mrs. Bean Counter Lawyer: Mr. Lawyer Lawyer: Mrs. Lawyer Employee Report I am a Programmer. I get 60000.0, and I get a bus pass. I am a Programmer. I get 65000.0, and I do not get a bus pass. I am an Accountant. I make 100000.0 plus a parking allowance of 50.0 I am an Accountant. I make 75000.0 plus a parking allowance of 150.0 I am a Lawyer. I get 180000.0, and I have 25 shares of stock. I am a Lawyer. I get 190000.0, and I have 125 shares of stock. Employee List: Natural Order Accountant - Mrs. Bean Counter - 75000.0 Accountant - Mr. Bean Counter - 100000.0 Lawyer - Mr. Lawyer - 180000.0 Lawyer - Mrs. Lawyer - 190000.0 Programmer - Mr. Ima Nerd - 60000.0 Programmer - Mrs. Ima Nerd - 65000.0 Employee List by Salary Programmer - Mr. Ima Nerd - 60000.0 Programmer - Mrs. Ima Nerd - 65000.0 Accountant - Mrs. Bean Counter - 75000.0 Accountant - Mr. Bean Counter - 100000.0 Lawyer - Mr. Lawyer - 180000.0 Lawyer - Mrs. Lawyer - 190000.0 Employee List by Name Accountant - Mr. Bean Counter - 100000.0 Programmer - Mr. Ima Nerd - 60000.0 Lawyer - Mr. Lawyer - 180000.0 Accountant - Mrs. Bean Counter - 75000.0 Programmer - Mrs. Ima Nerd - 65000.0 Lawyer - Mrs. Lawyer - 190000.0 ----- Here's the classes that need to be built out
import java.io.*; import java.util.*; import lab7.comparators.*; import lab7.inheritance.*; public class Main { public static void main(String [] args) { ArrayListmyList = new ArrayList(); myList.add(new Programmer("Mr. Ima Nerd", 40000, 20000, true)); myList.add(new Programmer("Mrs. Ima Nerd", 45000, 20000, false)); myList.add(new Accountant("Mr. Bean Counter", 100000, 0, 50.00)); myList.add(new Accountant("Mrs. Bean Counter", 75000, 0, 150.00)); myList.add(new Lawyer("Mr. Lawyer", 150000, 30000, 25)); myList.add(new Lawyer("Mrs. Lawyer", 170000, 20000, 125)); System.out.println("Employee List"); for(Employee e : myList) System.out.println(e); System.out.println(); System.out.println("Employee Report"); for(Employee e : myList) e.report(); System.out.println(); Collections.sort(myList); System.out.println("Employee List: Natural Order"); for(Employee e : myList) System.out.println(e.getType() + " - " + e.getName() + " - " + e.getSalary()); System.out.println(); Collections.sort(myList, new SalaryComparator()); System.out.println("Employee List by Salary"); for(Employee e : myList) System.out.println(e.getType() + " - " + e.getName() + " - " + e.getSalary()); System.out.println(); Collections.sort(myList, new NameComparator()); System.out.println("Employee List by Name"); for(Employee e : myList) System.out.println(e.getType() + " - " + e.getName() + " - " + e.getSalary()); System.out.println(); }//end main }// end class --- Accountant class that needs to be build per details listed public class Accountant | extends Employee The Accountant class derived from employee. Accountants get a parking stipend Field Summary: private double - parkingStipend (The parkingStipend acccountants receive) Fields inherited from class lab7.inheritance.Employee: salary Salary: BASE- private final double BASE - This is a constant representing the BASE pay and can't be changed. It is set as the first line of the constructor - private String name, protected double salary (The salary is the BASE + the additional salary) Constructor Detail: public Employee(String name,double basePayrate,double additionalPayrate) Throws:IllegalArgumentException - if any of the strings are empty or the doubles are less than 0, IllegalArgumentException - if any of the strings are null Method Detail: getParkingStipend: public double getParkingStipend(), public void report() report prints to the screen I am an accountant. I make "the salary from the base class" plus a parking stipend of "the parking stipend value" Specified by: report in class Employee, toString - public String toString(), returns Accountant: the parents toString, Overrides: toString in class Employee Returns: String --- Employee class that needs to be build per details listed public abstract class Employee | extends Object implements Comparable The Generic Employee Base class. There will never just be an employee NOTE: All parameters will be passed as final and all precondtions will be meField Summary: private double BASE: This is a constant representing the BASE pay and can't be changed. private String name, protected double salary (The salary is the BASE + the additional salary) Constructor Summary Employee(String name, double basePayrate, double additionalPayrate) - EVC All parameters are for the private class level variables. Method Summary int compareTo(Employee another) - compareTo first by type if the types are the same then by salary double getBaseSalary(), String getName(), double getSalary(), String getType() This methods first gets the class via getClass and then the simple name abstract void report() - The abstract method that will be overridden in the children String toString()- Simply returns the name - Methods inherited from class java.lang.Object - clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait Field Detail BASE private final double BASE- This is a constant representing the BASE pay and can't be changed. It is set as the first line of the constructor name private String name, protected double salary: The salary is the BASE + the additional salary Constructor Detail public Employee(String name,double basePayrate,double additionalPayrate) Parameters:name - The name,basePayrate - The base pay,additionalPayrate - The additonal pay Throws:IllegalArgumentException - if any of the strings are empty or the doubles are less than 0,IllegalArgumentException - if any of the strings are null Method Detail: public double getSalary(),public double getBaseSalary(), public String getName(),public String getType()- This methods first gets the class via getClass and then the simple name Returns: String the String of the simpleName- public String toString(),Overrides: toString in class Object Returns: String The name compareTo: public int compareTo(Employee another), compareTo first by type if the types are the same then by salary, Specified by: compareTo in interface Comparable, Parameters: another - - Representing the BCR to the DCO Returns: int The order Throws:IllegalArgumentException - if another is null report: public abstract void report(),The abstract method that will be overridden in the children ----- Lawyer class that needs to be build per details listed public class Lawyer | extends Employee: The lawyer class derived from employee. Lawyers get stock options NOTE: All parameters will be passed as final and all precondtions will be met Field Summary private int stockOptions Fields inherited from class lab7.Employee: salary Constructor Summary: Lawyer(String name, double basePayrate, double additionalPayrate, int stockOptions) Method Summary All MethodsInstance MethodsConcrete Methods Modifier and Type Method Description int getStockOptions() void report(): report prints to the screen I am an lawyer. String toString()- returns Lawyer: the parents toString Methods inherited from class lab7.cscd211inheritance.Employee:compareTo, getBaseSalary, getName, getSalary, getType Methods inherited from class java.lang.Object: clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait Field Detail stockOptions - private int stockOptions Constructor Detail Lawyer - public Lawyer(String name,double basePayrate,double additionalPayrate,int stockOptions) Parameters:name - The name basePayrate - The base pay additionalPayrate - The additonal pay stockOptions - The stock options Throws: IllegalArgumentException - if stock options is less than 0 Method Detail: public int getStockOptions(),p ublic void report(): report prints to the screen I am an lawyer. I get "the salary from the base class" and I have "stock options value" shares of stock. Specified by: report in class Employee - toString, public String toString()- returns Lawyer: the parents toString, Overrides: toString in class Employee Returns: String --- Programmer class that needs to be build per details listed public class Programmer|extends Employee The Programmer class derived from employee. Accountants get a bus pass maybe NOTE: All parameters will be passed as final and all precondtions will be met Field Summary private boolean busPass:A programmer is given a bus pass Fields inherited from class lab7.inheritance.Employee: salary Constructor Summary Constructors Constructor Description Programmer(String name, double basePayrate, double additionalPayrate, boolean busPass) EVC additional parameter is a bus pass. Method Summary All MethodsInstance MethodsConcrete Methods Modifier and Type Method Description boolean getBusPass(),void report(),report prints to the screen I am a programmeString toString() returns Programmer: the parents toString Methods inherited from class lab7.inheritance.Employee:compareTo, getBaseSalary, getName, getSalary, getType Methods inherited from class java.lang.Object:clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait Field Detail busPass: private boolean busPass- A programmer is given a bus pass Constructor Detail public Programmer(String name,double basePayrate,double additionalPayrate,boolean busPass)-EVC additional parameter is a bus pass. Parameters:name - The name,basePayrate - The base pay,additionalPayrate - The additonal pay,busPass - The bus pass Method Detail public boolean getBusPass(),public void report()-report prints to the screen I am a programmer. I get "the salary from the base class" and I get a bus pass or and I do not get a bus pass, Specified by: report in class Employee, public String toString()- returns Programmer: the parents toString Overrides: toString in class Employee, Returns:String
Step by Step Solution
There are 3 Steps involved in it
Step: 1
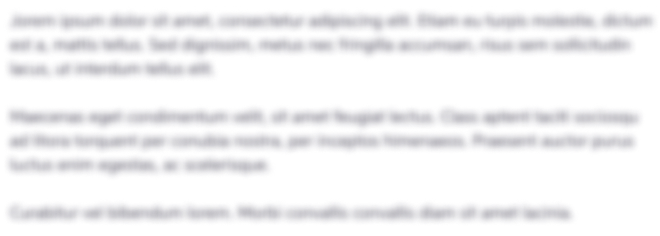
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started