Question
Create a simple database with three fields : ID, LastName, FirstName all as Strings. You should define a class called DataBaseRecord with three private String
Create a simple database with three fields : ID, LastName, FirstName all as Strings. You should define a class called DataBaseRecord with three private String elements (the three fields), along with the appropriate methods. Here's what mine looked like: public class DataBaseRecord { private String ID; private String first; private String second; DataBaseRecord(String a,String b,String c) { ID=new String(a); first=new String(b); second=new String(c); } public String toString() { return ID+" "+first+" "+second; } } You should also declare an object called DataBaseArray which is an array of DatabaseRecords. Records of type DataBaseRecord should be added at the end of the DataBaseArray. Create an IndexRecord class: public class IndexRecord { private String key; private int where; //other stuff here } Now create an IndexArray. This is an array of IndexRecord and is to be implemented as an OrderedArray class like the one we developed in class. That is, insertions must maintain the order in the array, where order is maintained by the key value. Note that this means you need to define a compareTo method in the IndexRecord object. Iterators Your IndexArray must implement an interator. An iterator is simply a variable that maintains a current reference into the data structure. In this instance, since IndexArray is a static array, the iterator is just an integer; a pointer into the array. You should implement the following methods: void iteratorInitFront - set the iterator to zero void iteratorInitBack - set the iterator to the last element in the array boolean hasNext - returns true if iterator<= current last index in the array, false otherwise. boolean hasPrevious - returns true if iterator>0 , false otherwise int getNext - returns the where component of the IndexRecord referenced by iterator and then increments the iterator int getPrevious - returns the where component of the IndexRecord referenced by iterator and then decrements the iterator Finally, create an class called DataBase. Here's what mine looked like: public class DataBase { private DataBaseArray myDB; private IndexArray ID , First , Last; public DataBase() { myDB=new DataBaseArray(100); ID = new IndexArray(100); First=new IndexArray(100); Last=new IndexArray(100); } //other stuff here } To get an idea of how the iterators work, here's the code I have for printing out the database in ascending order by first name: public void ListByFirstAscending() { First.iteratorInitFront(); while(First.hasNext()) { int temp=First.getNext(); System.out.println(myDB.retrieve(temp)); } } Here's the output from the following for my sample data set: 1234 alice jones 9988 dave bing 2244 ed smiley 6633 ellen nance 3234 mac edwards 6655 mary rogers 9999 mike adams 4234 roger morris 2233 sue charles 1235 zelda smith
has to read in a text file with this inside
Dunn Sean 31111 Duong Geoffrey 29922 Fazekas Nicholas 31100 Prezioso Stefano 22223 Puvvada Mohana 11224 Ravikumar Rakhi 11226 Salyers Matthew 11227 Gillespie William 49587 Hess Caleb 29282 Armatis Jared 34512 Beckman Allan 35176 Wang Zhen 22113 Wingett Jordan 12345 Belt Keith 34987 Bixler Tyler 22234 Chambers Quentin 22567 Chinni Adithya 28456 Donheiser Michael 28456 Kondrashov Mikhail 33331 Kraus Laura 33332 Krupp Phillip 49888 Maass John 44112 McCarty Amanda 44223 Moldovan Gregory 44335 Oshiyoye Adekunle 44556 Pagalos Frank 33112 Perski Zackery 33221 Saunders Jordan 77556 Simpson Ashlynne 77665 Szalai Kyle 33112 Witting Robert 21354
use this driver program:
import java.util.*; public class Driver { public static void main(String[] args) { DataBase d=new DataBase(); int response; Scanner keyboard=new Scanner(System.in); /* Read the data into the database from the external disk file here * IMPORTANT: duplicate ID numbers should not be added. Disregard * the entire record for duplicate IDs */ do { System.out.println(" 1 Add a new student"); System.out.println(" 2 Delete a student"); System.out.println(" 3 Find a student by ID"); System.out.println(" 4 List students by ID increasing"); System.out.println(" 5 List students by first name increasing"); System.out.println(" 6 List students by last name increasing"); System.out.println(" 7 List students by ID decreasing"); System.out.println(" 8 List students by first name decreasing"); System.out.println(" 9 List students by last name decreasing"); System.out.println(" "); System.out.println(" 0 End"); response=keyboard.nextInt(); switch (response) { case 1: d.addIt(); //Note: if the user enters an ID already in use, issue a warning and return to the menu break; case 2: d.deleteIt(); //Note: output either "Deleted" or "ID not Found" and return to menu break; case 3: d.findIt(); //Note: output the entire record or the message "ID not Found" and return to menu break; case 4: d.ListByIDAscending(); break; case 5: d.ListByFirstAscending(); break; case 6: d.ListByLastAscending(); break; case 7: d.ListByIDDescending(); break; case 8: d.ListByFirstDescending(); break; case 9: d.ListByLastDescending(); break; default: } } while (response!=0); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
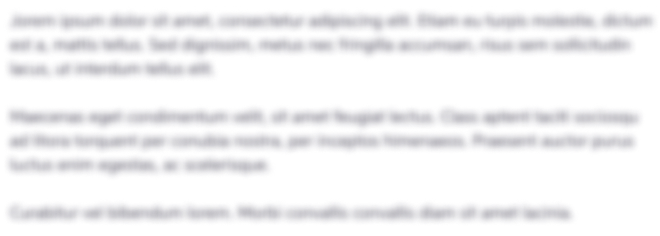
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started