Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Create a source file in c++ that impements the header file given by only using the libraries in the main file also given, copy and
Create a source file in c++ that impements the header file given by only using the libraries in the main file also given, copy and paste the files. Header and main files are not to be changed, just used to implement the source file. If compiled correctly, "Assignment Complete" will appear and all tests have passed. (trendtracker.h)-Header file #ifndef TRENDTRACKER_H #define TRENDTRACKER_H #include #include using namespace std; class Trendtracker { // For the mandatory running times below: // n is the number of hashtags in the Trendtracker. public: // Creates a new empty collection of hashtags. Trendtracker(); // Inserts a hashtag (tweeted 0 times) into the Trendtracker. // If the hashtag already is in Trendtracker, does nothing. // // Must run in O(n) time. void insert(string ht); // Return the number of hashtags in the Trendtracker. // // Must run in O(1) time. int size(); // Adds 1 to the total number times a hashtag has been tweeted. // If the hashtag does not exist in TrendTracker, does nothing. // // Must run in O(n) time. void tweeted(string ht); // Returns the number of times a hashtag has been tweeted. // If the hashtag does not exist in Trendtracker, returns -1. // // Must run in O(n) time. int popularity(string name); // Returns a most-tweeted hashtag. // If the Trendtracker has no hashtags, returns "". // // Must run in O(n) time. string top_trend(); // Fills the provided vector with the k most-tweeted hashtags, // in order of most-tweeted-to-least-tweeted. // // If there are fewer than k hashtags, then the vector is filled // with all hashtags (in most-tweeted-to-least-tweeted order). // // Must run in O(nk) time. void trending(int k, vector &T); private: // A simple class representing a hashtag and // the number of times it has been tweeted. class Entry { public: string hashtag; int pop; }; // Entries containing each hashtag and its popularity. vector E; }; #endif
(main.cpp)
#include
#include
#include#include #include #include #include "trendtracker.h" using namespace std; using namespace chrono; inline void _test(const char* expression, const char* file, int line) { cerr << "test(" << expression << ") failed in file " << file; cerr << ", line " << line << "." << endl; abort(); } #define test(EXPRESSION) ((EXPRESSION) ? (void)0 : _test(#EXPRESSION, __FILE__, __LINE__)) int main() { // Setup srand(2017); vector R; string s, line; system_clock::time_point start, end; float dur; // Test size() and insert(). Trendtracker T1; test(T1.size() == 0); test(T1.popularity("#algorithms") == -1); test(T1.popularity("#cs4all") == -1); test(T1.popularity("#programming") == -1); T1.insert("#cs4all"); test(T1.size() == 1); test(T1.popularity("#algorithms") == -1); test(T1.popularity("#cs4all") == 0); test(T1.popularity("#programming") == -1); T1.insert("#algorithms"); test(T1.size() == 2); test(T1.popularity("#algorithms") == 0); test(T1.popularity("#cs4all") == 0); test(T1.popularity("#programming") == -1); T1.insert("#programming"); test(T1.size() == 3); test(T1.popularity("#algorithms") == 0); test(T1.popularity("#cs4all") == 0); test(T1.popularity("#programming") == 0); T1.insert("#algorithms"); test(T1.size() == 3); test(T1.popularity("#algorithms") == 0); test(T1.popularity("#cs4all") == 0); test(T1.popularity("#programming") == 0); T1.tweeted("#programming"); test(T1.popularity("#algorithms") == 0); test(T1.popularity("#cs4all") == 0); test(T1.popularity("#programming") == 1); T1.tweeted("#programming"); test(T1.popularity("#algorithms") == 0); test(T1.popularity("#cs4all") == 0); test(T1.popularity("#programming") == 2); T1.tweeted("#programming"); test(T1.popularity("#algorithms") == 0); test(T1.popularity("#cs4all") == 0); test(T1.popularity("#programming") == 3); T1.tweeted("#cs4all"); test(T1.popularity("#algorithms") == 0); test(T1.popularity("#cs4all") == 1); test(T1.popularity("#programming") == 3); T1.tweeted("#algorithms"); test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 1); test(T1.popularity("#programming") == 3); T1.tweeted("#cs4all"); test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 2); test(T1.popularity("#programming") == 3); T1.tweeted("#datastructures"); test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 2); test(T1.popularity("#datastructures") == -1); test(T1.popularity("#programming") == 3); T1.insert("#datastructures"); test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 2); test(T1.popularity("#datastructures") == 0); test(T1.popularity("#programming") == 3); T1.tweeted("#datastructures"); test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 2); test(T1.popularity("#datastructures") == 1); test(T1.popularity("#programming") == 3); T1.tweeted("#datastructures"); test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 2); test(T1.popularity("#datastructures") == 2); test(T1.popularity("#programming") == 3); T1.tweeted("#datastructures"); test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 2); test(T1.popularity("#datastructures") == 3); test(T1.popularity("#programming") == 3); T1.tweeted("#datastructures"); test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 2); test(T1.popularity("#datastructures") == 4); test(T1.popularity("#programming") == 3); Trendtracker T2; T2.insert("#3333"); T2.insert("#programming"); T2.insert("#cs4all"); T2.insert("#C++"); T2.insert("#algorithms"); T2.tweeted("#programming"); T2.tweeted("#programming"); T2.tweeted("#programming"); T2.tweeted("#programming"); T2.tweeted("#programming"); T2.tweeted("#C++"); T2.tweeted("#C++"); T2.tweeted("#C++"); T2.tweeted("#C++"); T2.tweeted("#cs4all"); T2.tweeted("#cs4all"); T2.tweeted("#cs4all"); T2.tweeted("#algorithms"); T2.tweeted("#algorithms"); T2.tweeted("#3333"); test(T2.popularity("#programming") == 5); test(T2.popularity("#cs4all") == 3); test(T2.popularity("#algorithms") == 2); test(T2.popularity("#C++") == 4); test(T2.popularity("#3333") == 1); T2.insert("#3333"); T2.insert("#programming"); T2.insert("#cs4all"); T2.insert("#C++"); T2.insert("#algorithms"); test(T2.popularity("#programming") == 5); test(T2.popularity("#cs4all") == 3); test(T2.popularity("#algorithms") == 2); test(T2.popularity("#C++") == 4); test(T2.popularity("#3333") == 1); // Enforce no usage of global variables test(T1.popularity("#algorithms") == 1); test(T1.popularity("#cs4all") == 2); test(T1.popularity("#datastructures") == 4); test(T1.popularity("#programming") == 3); Trendtracker T3; test(T3.top_trend() == ""); T3.trending(1, R); test(R.size() == 0); T3.trending(2, R); test(R.size() == 0); T3.trending(3, R); test(R.size() == 0); T3.insert("#programming"); test(T3.top_trend() == "#programming"); T3.trending(1, R); test(R.size() == 1); test(R[0] == "#programming"); T3.trending(2, R); test(R.size() == 1); test(R[0] == "#programming"); T3.trending(3, R); test(R.size() == 1); test(R[0] == "#programming"); T3.tweeted("#programming"); test(T3.top_trend() == "#programming"); T3.trending(1, R); test(R.size() == 1); test(R[0] == "#programming"); T3.trending(2, R); test(R.size() == 1); test(R[0] == "#programming"); T3.trending(3, R); test(R.size() == 1); test(R[0] == "#programming"); T3.insert("#C++"); T3.tweeted("#C++"); T3.tweeted("#C++"); test(T3.top_trend() == "#C++"); T3.trending(1, R); test(R.size() == 1); test(R[0] == "#C++"); T3.trending(2, R); test(R.size() == 2); test(R[0] == "#C++"); test(R[1] == "#programming"); T3.trending(3, R); test(R.size() == 2); test(R[0] == "#C++"); test(R[1] == "#programming"); T3.insert("#3333"); T3.tweeted("#3333"); T3.tweeted("#3333"); T3.tweeted("#3333"); test(T3.top_trend() == "#3333"); T3.trending(1, R); test(R.size() == 1); test(R[0] == "#3333"); T3.trending(2, R); test(R.size() == 2); test(R[0] == "#3333"); test(R[1] == "#C++"); T3.trending(3, R); test(R.size() == 3); test(R[0] == "#3333"); test(R[1] == "#C++"); test(R[2] == "#programming"); T3.insert("#cs4all"); T3.tweeted("#cs4all"); T3.tweeted("#cs4all"); T3.tweeted("#cs4all"); T3.tweeted("#cs4all"); test(T3.top_trend() == "#cs4all"); T3.trending(1, R); test(R.size() == 1); test(R[0] == "#cs4all"); T3.trending(2, R); test(R.size() == 2); test(R[0] == "#cs4all"); test(R[1] == "#3333"); T3.trending(3, R); test(R.size() == 3); test(R[0] == "#cs4all"); test(R[1] == "#3333"); test(R[2] == "#C++"); // At this point: // cs4all: 4 // 3333: 3 // C++: 2 // programming: 1 T3.tweeted("#programming"); T3.tweeted("#programming"); T3.tweeted("#programming"); T3.tweeted("#programming"); test(T3.top_trend() == "#programming"); T3.trending(5, R); test(R.size() == 4); test(R[0] == "#programming"); test(R[1] == "#cs4all"); test(R[2] == "#3333"); test(R[3] == "#C++"); // At this point: // programming: 5 // cs4all: 4 // 3333: 3 // C++: 2 T3.tweeted("#cs4all"); T3.tweeted("#cs4all"); T3.tweeted("#3333"); test(T3.top_trend() == "#cs4all"); T3.trending(5, R); test(R.size() == 4); test(R[0] == "#cs4all"); test(R[1] == "#programming"); test(R[2] == "#3333"); test(R[3] == "#C++"); // At this point: // cs4all: 6 // programming: 5 // 3333: 4 // C++: 2 for (int i = 0; i < 10000; ++i) T3.tweeted("#C++"); test(T3.top_trend() == "#C++"); T3.trending(5, R); test(R.size() == 4); test(R[0] == "#C++"); test(R[1] == "#cs4all"); test(R[2] == "#programming"); test(R[3] == "#3333"); Trendtracker T4; // Test insert() performance ifstream f; f.open("common.txt"); assert(f.is_open()); // If this fails, you're missing common.txt start = system_clock::now(); while (getline(f, line)) T4.insert(line); end = system_clock::now(); f.close(); test(T4.size() == 3597); dur = duration (end - start).count(); test(dur < 2.0); // Test tweeted() performance f.open("common.txt"); start = system_clock::now(); while (getline(f, line)) T4.tweeted(line); end = system_clock::now(); f.close(); dur = duration (end - start).count(); test(dur < 2.5); // Test popularity() performance f.open("common.txt"); start = system_clock::now(); for (int i = 0; i < 1000; ++i) { getline(f, line); test(T4.popularity(line) > 0); } end = system_clock::now(); f.close(); dur = duration (end - start).count(); test(dur < 0.3); // Test top_trend() performance. start = system_clock::now(); for (int i = 0; i < 1000; ++i) test(T4.top_trend() == "#finishing"); end = system_clock::now(); dur = duration (end - start).count(); test(dur < 1.2); // Test trending() performance. start = system_clock::now(); for (int i = 0; i < 1000; ++i) T4.trending(10, R); end = system_clock::now(); dur = duration (end - start).count(); test(dur < 3.5); test(R[0] == "#finishing"); test(R[1] == "#discovering"); start = system_clock::now(); for (int i = 0; i < 100; ++i) T4.trending(1000, R); end = system_clock::now(); dur = duration (end - start).count(); test(dur < 0.5); test(R[0] == "#finishing"); test(R[1] == "#discovering"); cout << "Assignment complete." << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
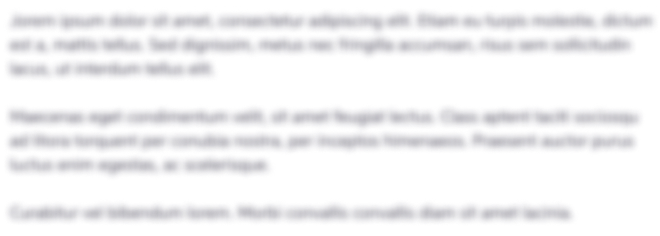
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started