Question
Create a stack ADT to be used on a simple calculator The calculator should be capable of evaluating the following simple expression: 2.3 * 1.2
Create a stack ADT to be used on a simple calculator
The calculator should be capable of evaluating the following simple expression:
2.3 * 1.2 + 14.0
The operators for our simple calculator are
Addition +
Subtraction -
Multiplication *
Division /
The excercise involves one class: Calculator. The Calculator class is incomplete. You must fill in the details to make the class complete by focusing on the "insert your code here" phrase in the class.
For the Calculator class, you will need to complete:
doOp()
repeatOps()
evalExp()
What code do I need to put in between the bolded areas to make the methods complete?
Leave a comment if there are any questions.
public class Calculator { private StackvalStk; // the operand stack private Stack opStk; // the operator stack private int prec[] = new int[128]; // used for comparing precedent of operators // Constructor public Calculator() { valStk = new Stack (10); opStk = new Stack (10); prec['$'] = 0; // Used to signal end of input condition prec['+'] = 1; // + and - have lower precedence than * and / prec['-'] = 1; prec['*'] = 2; prec['/'] = 2; } /** * Take two operands from the valStack along with an operator from opStack and perform the * operation, pushing the result back on the valstack. * * @param obj * the object to be pushed */ void doOp() { // Begin: Insert your code here to complete the method // End: Of your code } /** * Repeat processing the operation while the operator on the top of the opStack * has a higher precedence than the current operator *
* @param refOp * the current operator */ void repeatOps(Character refOp) { // Begin: Insert your code here to complete the method // End: Of your code } /** * Evaluate a stream of tokens representing an arithmetic expression (with Doubles) *
* @param opstr * the string containing the expression, e.g., "3.0 * 2.0 + 1.0" *
* @return * true if the stack is empty, otherwise false * */ public Double evalExp(String opstr) { String[] tokens = opstr.split(" "); for (int i = 0; i < tokens.length; i++) { Double val; try { // Managing the exception val = Double.parseDouble(tokens[i]); } catch (NumberFormatException e) { val = null; } // Begin: Insert your code here to complete the method // End: Of your code } // Make sure all the operands have been processed by processing an operator // signaling the end // Begin: Insert your code here to complete the method // End: Of your code } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
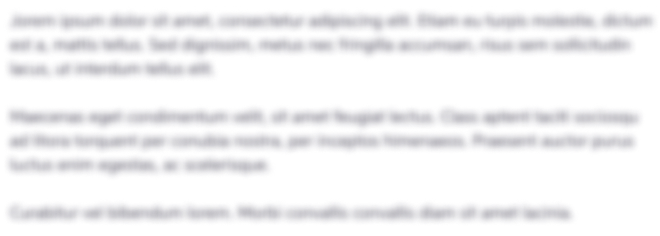
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started