Question
Create a TeaTime class that tests tea for taste and temperature. If the tea is too hot or tastes bad, an error message appears and
Create a TeaTime class that tests tea for taste and temperature. If the tea is too hot or tastes bad, an error message appears and an indication of the error location in the program. If the tea tastes good, a message indicating that the tea is just right is printed. In any case, the program always prints a request for another cup.
Create a project called TeaTime.
Create a TeaException class that extends Exception.
Create a TeaTime application - class with a main method.
Create a boolean field in the TeaTime class called
badTaste
.
The default value is false.
The field may be static, use getters and setters or be passed as a parameter to the tasteTea() method in the next step - Programmer's choice.
Create a tasteTea() method in the TeaTime class that throws a TeaException if badTaste is true.
Create a testTea() method that calls the tasteTea() method and then immediately prints a message that the tea is just right.
Surround the tasteTea() method and the just right message with a try block. Add an appropriate catch and finally block.
The catch method should print the message and the stack trace
Make sure that a message asking for another cup is always printed.
In the main method, include statements that
set the badTaste value to false and run testTea()
then true and run testTea().
Create a TeaTooHotException that extends TeaException.
In the TeaTime class, create getters and setters for an int field called temperature. The default temperature is 149.
Modify the tasteTea() method in TeaTime to throw a TeaTooHotException if the temperature is greater than 150 degrees. Insert this condition and throw statement before the bad taste exception.
Modify the testTea() method in TeaTime to catch the new Exception in the appropriate location. In the catch block print a message that the temperature will be corrected for the next cup and set the temperature to 149.
In the main method of TeaTime, include statements that
set the temperature value past 150 and run testTea()
Then set badTaste to true and run testTea(). Remember that the temperature was corrected in the catch block.
set the temperature to a value past 150 and the badTaste to true. See the answers for a sample sequence of events that tests all of the conditions. Note: The answers below and results may print in a slightly different order, since exceptions and System.out.println() print from different streams. This is acceptable, as long as the flow of the program makes sense. One solution is to use System.err.println(), as that is not buffered and uses the same output stream as Exception to print.
As required for all assignments from now on
validate the proper functionality using the example answers below and review the Concepts and Ideas checklist format the code
add a comment at the top of the file that reads @ author and your name
build the javadocs
Verify that the jar file contains source and class files and the MANIFEST.MF file contains a Main-Class. See the Resources section for Configuring Eclipse to view Jar files.
Run the application from the jar file, either from the command line using java -jar jarfilename or from Eclipse. See the Resources section for Configuring Eclipse to run Jar files.
submit the jar file in the Assignment section of BlackBoard under View/Complete Assignment for this assignment.
Example:
################################################
Getting a cup of tea to taste...
The tea is just right.
May I have another cup?
################################################
################################################
Getting a cup of tea to taste...
where the taste is bad
This tea tastes bad.
TeaException: This tea tastes bad.
at TeaTime.tasteTea(TeaTime.java:130)
at TeaTime.testTea(TeaTime.java:94)
at TeaTime.main(TeaTime.java:64)
May I have another cup?
################################################
################################################
Getting a cup of tea to taste...
where the taste is bad
where the tea is too hot
The tea is too hot to drink.
TeaTooHotException: The tea is too hot to drink.
at TeaTime.tasteTea(TeaTime.java:126)
at TeaTime.testTea(TeaTime.java:94)
at TeaTime.main(TeaTime.java:67)
I will cool the next cup.
May I have another cup?
################################################
################################################
Getting a cup of tea to taste...
where the taste is bad
This tea tastes bad.
TeaException: This tea tastes bad.
at TeaTime.tasteTea(TeaTime.java:130)
at TeaTime.testTea(TeaTime.java:94)
at TeaTime.main(TeaTime.java:69)
May I have another cup?
################################################
################################################
Getting a cup of tea to taste...
where the taste is bad
where the tea is too hot
The tea is too hot to drink.
TeaTooHotException: The tea is too hot to drink.
at TeaTime.tasteTea(TeaTime.java:126)
at TeaTime.testTea(TeaTime.java:94)
at TeaTime.main(TeaTime.java:72)
I will cool the next cup.
May I have another cup?
################################################
################################################
Getting a cup of tea to taste...
The tea is just right.
May I have another cup?
################################################
Step by Step Solution
There are 3 Steps involved in it
Step: 1
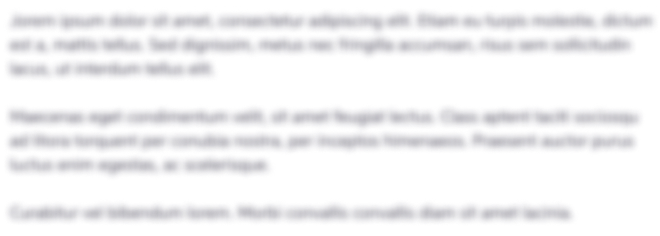
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started