Question
create a test program for the Pet class: Use the class Pet Write a program to read data for three Pet s and display the
create a test program for the Pet class:
Use the class Pet
Write a program to read data for three Pets and display the following information, using the Pet instance methods:
The names of the smallest and largest Pets (by weight).
The name of the youngest and oldest Pets.
The average weight of the three Pets.
The average age of the three Pets.
Hints: You can keep track of the smallest/largest and youngest/oldest Pets as you are reading them in once the first one has been initialized you can assume it is the smallest/largest and youngest/oldest to start, and then compare later Pets against those. You can also accumulate their weights and ages as they are being read in, starting from 0 for both. Be sure to calculate the average age as a double, not by using integer division.
And here is the Pet source code. Thank you!
/** Class for basic pet data: name, age, and weight. */ public class Pet { private String name; private int age; //in years private double weight;//in pounds public Pet(String initialName, int initialAge, double initialWeight) { name = initialName; if ((initialAge < 0) || (initialWeight < 0)) { System.out.println("Error: Negative age or weight."); System.exit(0); } else { age = initialAge; weight = initialWeight; } } public void setPet(String newName, int newAge, double newWeight) { name = newName; if ((newAge < 0) || (newWeight < 0)) { System.out.println("Error: Negative age or weight."); System.exit(0); } else { age = newAge; weight = newWeight; } } public Pet(String initialName) { name = initialName; age = 0; weight = 0; } public void setName(String newName) { name = newName; //age and weight are unchanged. } public Pet(int initialAge) { name = "No name yet."; weight = 0; if (initialAge < 0) { System.out.println("Error: Negative age."); System.exit(0); } else age = initialAge; } public void setAge(int newAge) { if (newAge < 0) { System.out.println("Error: Negative age."); System.exit(0); } else age = newAge; //name and weight are unchanged. } public Pet(double initialWeight) { name = "No name yet"; age = 0; if (initialWeight < 0) { System.out.println("Error: Negative weight."); System.exit(0); } else weight = initialWeight; } public void setWeight(double newWeight) { if (newWeight < 0) { System.out.println("Error: Negative weight."); System.exit(0); } else weight = newWeight; //name and age are unchanged. } public Pet( ) { name = "No name yet."; age = 0; weight = 0; } public String getName( ) { return name; } public int getAge( ) { return age; } public double getWeight( ) { return weight; } public void writeOutput( ) { System.out.println("Name: " + name); System.out.println("Age: " + age + " years"); System.out.println("Weight: " + weight + " pounds"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
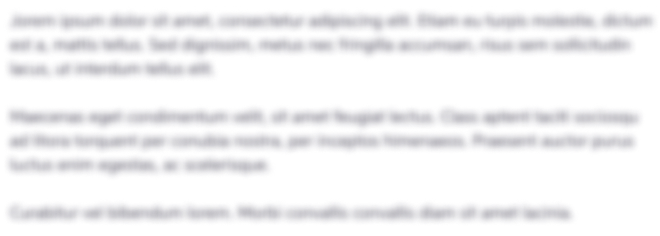
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started