Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Create a tool to count how many of each letter there are, and print both a table and a graph of the counts. Read in
Create a tool to count how many of each letter there are, and print both a table and a graph of the counts. Read in a stream of text UNTIL YOU ENCOUNTER END OF FILE (EOF). DO NOT READ A SINGLE LINE, READ THE ENTIRE FILE. Read one character at a time. Keep a count of how many of each letter you see in an array of 26 ints called: int count[26] ; Print the text totals of the count[] table, then create a horizontal graph of the data and print it on screen as shown below: Sample Run: a.out < histo.data count[ 0]: 320 count[ 1]: 160 count[ 2]: 80 count[ 3]: 40 count[ 4]: 20 count[ 5]: 10 count[ 6]: 5 count[ 7]: 2 count[ 8]: 1 count[ 9]: 2 count[10]: 17 count[11]: 41 count[12]: 67 count[13]: 97 count[14]: 127 count[15]: 157 count[16]: 191 count[17]: 227 count[18]: 257 count[19]: 283 count[20]: 331 count[21]: 367 count[22]: 373 count[23]: 379 count[24]: 383 count[25]: 389 320 a ================================================== 160 b ========================= 80 c ============ 40 d ====== 20 e === 10 f = 5 g 2 h 1 i 2 j 17 k == 41 l ====== 67 m ========== 97 n =============== 127 o =================== 157 p ======================== 191 q ============================= 227 r =================================== 257 s ======================================== 283 t ============================================ 331 u =================================================== 367 v ========================================================= 373 w ========================================================== 379 x =========================================================== 383 y ============================================================ 389 z ============================================================= Remember that characters are integer values. So when you are reading in characters, you can USE THEM AS NUMBERS AS WELL AS DISPLAYING THEM AS CHARACTERS. The principle is: 'c' - 'a' == 2 'z' - 'a' == 25 'a' - 'a' == 0 SO: c = tolower(cin.get()) ; if (isalpha(c)) count[c - 'a']++ ; // this saves you 26 lines of code Do NOT create a huge switch() or 56 if() statements, that'd be inefficient. There are files of sample data you can run against, just understand they're just samples, and your program must accurately count ANY input streams. They are: http://209.129.16.61/~hhaller/data/cisc192/asst5/histo.data http://209.129.16.61/~hhaller/data/cisc192/asst5/h.dat. http://209.129.16.61/~hhaller/data/cisc192/asst5/karla.txt h.dat and histo.data have the same distribution. One is 10 times larger. ================================================================= How to write the program. Ask the system how many columns the user's termainal has. (Windows is too stupid to provide this information, but Buffy will if you use int cols = atoi(getenv("COLUMNS") ) ; The same can be done with any Environment Variable. To see the Environment Variables, from Buffy's command line run: set | less Figure out how to use each line of your display by running my demo: count char graph bar 97 n: ========================= 127 o: ================================= 157 p: ========================================= 191 q: ================================================== Make an INTELLIGENT ESTIMATE of how big your numbers could be, and design your screen accordingly. Don't ask anybody to help, think for yourself. You may err. Keep fixing your errors until they go away. Once you know how many columns you will use just for your graph bars, call it COLS. COLS will be filled by the largest graph (the largest number) in count[]. Remember this. (Obviously, this means you must find the largest number in the array right after you finish filling it. Call that MAX.) For each element in the array: printf the count, flush-right, the character whose count it is, then calculate a "LENGTH" for that letter's graph bar: You want a bar whose "LENGTH" is to COLS as count[x] is to MAX. LENGTH count[x] ------- = ------- COLS MAX (Use the "ratio and proportion" calculation you learned in 6th grade.) Set up a nested for() which prints that many '=' chars, end the line with a ' ', and start the next line until all letters are done. GRADING CRITERIA: 0. The program must be able to process streams of any length, character counts from 0 to the maximum value an integer variable may contain. 1. The bars must be shorter than the screen is wide, they must NOT "wrap". (Regardless of input, no output line may extend past the right side of the screen.) 2. The lines must all start in the same column, so control the width of the printed numbers. 3. Your program must be able to handle files where character frequencies are quite large and/or quite small without wrapping, and while always printing at least some graphic bar(s). 4. Store the 'count' data in an array. Remember that you can record the counts in the array thusly: (after you read in a character into c with c = tolower(cin.get()) ; and confirmed that if(isalpha(c)) { you can increment the count for whichever character c contains with: count[c - 'a']++ ; (Do NOT create a 56-line switch() or multiple if() statements, that kind of thing gets programmers mocked by their peers. Study the line above until you can use it.) ====================================== Professional Note: You can have your program ask the Linux system for the number of lines and columns the user has in hir window with: int lines = atoi(getenv("LINES") ; int cols = atoi(getenv("COLUMNS") ; Like so many real tools, this won't work on Windows. This document is copyright (C) 2000 T. E. Harrisburg, M.S., All Rights Reserved. It may not be stored on any information storage and retrieval device, web page, etc., without the written consent of the owner. Part B: Character count with Vertical Histogram Display. 50 points. 10 extra for GENERALIZED vertical number routine. (Not tied to a given range of values.) Read in a stream of text UNTIL YOU ENCOUNTER END OF FILE (EOF). Read one character at a time. Keep a count of how many of each letter you see in an array of 26 ints called: int count[26] ; Example run: a.out < histo.data Counts: count[ a]: 320 count[ b]: 160 count[ c]: 80 count[ d]: 40 count[ e]: 20 count[ f]: 10 count[ g]: 5 count[ h]: 2 count[ i]: 1 count[ j]: 2 count[ k]: 17 count[ l]: 41 count[ m]: 67 count[ n]: 97 count[ o]: 127 count[ p]: 157 count[ q]: 191 count[ r]: 227 count[ s]: 257 count[ t]: 283 count[ u]: 331 count[ v]: 367 count[ w]: 373 count[ x]: 379 count[ y]: 383 count[ z]: 389 Graph: * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * a b c d e f g h i j k l m n o p q r s t u v w x y z ==================================== How to design the program: Use the instructions in the previous Horizontal Histogram Assignment we just completed. Do not deviate from it regarding input and storage of counts. 0. Decide how many lines you want to use for your display, call it LINES. Number the lines highest to lowest, top to bottom of the screen. 1. Find the biggest number in the array, call it MAX 2. For each LINE on your display, calculate a minimum threshold value required for an element of count[] to get a '*' insread of a ' '. Call it THRESHOLD. LINE THRESHOLD ---- = ---------- LINES MAX So: THRESHOLD = (MAX * LINE) / LINES ; This also should be obvious to you, since it's 6th grade arithmetic. If you don't understand it easily and completely, you need to seek remediation. The screen will be processed by a for() loop which runs from LINES to 1, (a descending for() loop.) Run a for() loop which steps through the count[] array, and decides to print either a '*' or ' ' for each column by comparing count[LINE] with THRESHOLD. (Each column represents a letter in count[]). -------------------------- Discussion: For each line in, say, a 60-line screen, you start with the top line, (line 60). To "get a star" on the TOP LINE, the number in the count[] element must be EQUAL TO MAX, that is, the top line represents the largest count we've seen in the input. The next line down represents 59/60 ths of a 60 line screen. The bottom line represents 1/60 th of a 60 line screen. So for each line, you check each element in count, and if it holds that fraction of MAX or more, it gets a star, otherwise it gets a space. (Once a column gets a star, it will get stars for all the lines below that one, obviously.) Obviously, the "outer" loop will be a downward-counting loop, something like: for (line = LINES ; line > 0 ; line--) ====================================== Professional Note: Vertical numbers. To print out the numeric totals vertically beneath the columns they correspond to, come up with a general purpose function for formatting numbers of arbitrary size vertically using several lines. a.out < histo.data * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * ---------------------------------------------------- a b c d e f g h i j k l m n o p q r s t u v w x y z 3 1 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 2 2 2 3 3 3 3 3 3 2 6 8 4 2 1 0 0 0 0 1 4 6 9 2 5 9 2 5 8 3 6 7 7 8 8 0 0 0 0 0 0 5 2 1 2 7 1 7 7 7 7 1 7 7 3 1 7 3 9 3 9 a.out < h.dat * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * ---------------------------------------------------- a b c d e f g h i j k l m n o p q r s t u v w x y z 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 2 2 2 2 2 8 9 4 2 1 0 0 0 0 0 0 2 3 5 7 8 0 2 4 5 8 0 1 1 1 1 0 0 5 2 1 5 2 1 0 1 9 3 7 4 1 8 7 8 4 9 6 6 0 3 6 9 4 2 1 5 2 6 8 1 5 1 5 1 7 7 6 5 7 0 9 6 6 9 3 7 0 3 8 4 2 6 8 4 2 2 6 2 8 2 8 0 2 4 2 2 4 1 8 8 7 5 1 9 0 0 0 0 0 0 0 8 4 8 8 4 8 8 8 8 4 8 8 2 4 8 2 6 2 6 UNIX NOTE: If you're writing this so it senses the size, in columns and rows, of the user's terminal, you may need to explicitly do this at the command line: $ export LINES $ export COLUMNS If you encounter "segfault" errors, this may be the problem. (I set your .bashrc files to do this, it ought to be OK.)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
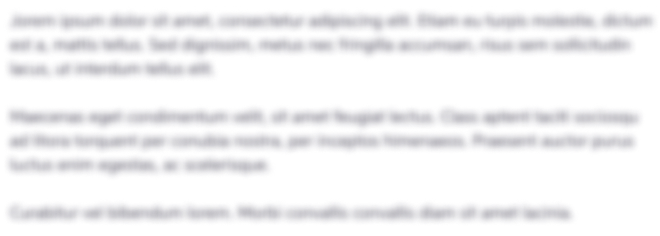
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started