Question
Create a UML DIAGRAM for the program below. The code is below. And I took a picture of the instructions. package homework4; import java.util.ArrayList; import
Create a UML DIAGRAM for the program below. The code is below. And I took a picture of the instructions.
package homework4;
import java.util.ArrayList;
import java.util.Scanner;
abstract class Appointment{
private String description;
private String lastName;
private int hours=0;
private int minutes=0;
public void setDescription(String description){
this.description = description;
}
public void setLastName(String lastName){
this.lastName = lastName;
}
public void setHours(int hours){
this.hours = hours;
}
public void setMinutes(int minutes){
this.minutes = minutes;
}
public String getDescription(){
return this.description;
}
public String getLastName(){
return this.lastName;
}
public int getHours(){
return this.hours;
}
public int getMinutes(){
return this.minutes;
}
abstract public boolean occursOn(int year,int month,int day);
}
class Onetime extends Appointment{
private int year;
private int month;
private int day;
public void setYear(int year) {
this.year=year;
}
public void setMonth(int month) {
this.month=month;
}
public void setDay(int day) {
this.day=day;
}
public int getYear() {
return this.year;
}
public int getMonth() {
return this.month;
}
public int getDay() {
return this.day;
}
@Override
public boolean occursOn(int year, int month, int day) {
if(this.year==year && this.month==month && this.day==day)
return true;
return false;
}
}
class Daily extends Appointment{
@Override
public boolean occursOn(int year, int month, int day) {
// TODO Auto-generated method stub
return true;
}
}
class Monthly extends Appointment{
private int day;
public void setDay(int day) {
this.day=day;
}
public int getDay() {
return this.day;
}
@Override
public boolean occursOn(int year, int month, int day) {
if(this.day==day)
return true;
return false;
}
}
public class TestAppointmentss {
static Scanner sc= new Scanner(System.in);
public static void main(String[] args) {
ArrayList
System.out.println("How many appointments do you with to make?");
int num = sc.nextInt();
for(int i = 1;i
System.out.println("Please make a selection:");
System.out.println("1. One Time Appointment");
System.out.println("2. Daily Appointment");
System.out.println("3. Monthly Appointment");
int selection = sc.nextInt();
al.add(makeAppointment(selection));
}
System.out.println("*******!!!Thank you for making all your appointments!!!*********");
char choice='y';
while(choice=='y') {
System.out.println("What is the date you wish to look up in your Appointment's Calendar");
System.out.print("Enter the month: ");
int month=sc.nextInt();
System.out.print("Enter the day: ");
int day=sc.nextInt();
System.out.print("Enter the year: ");
int year=sc.nextInt();
System.out.println("On "+month+"/"+day+"/"+year+" you have the following appointments:");
for(Appointment a:al) {
boolean isPresent=checkAppointment(a,year,month,day);
if(isPresent) {
System.out.println(a.getDescription()+" with "+a.getLastName()+" at "+a.getHours()+":"+a.getMinutes());
}
}
System.out.println("Do you wish to look up another date?");
choice=sc.next().charAt(0);
}
System.out.println("Thank you for using Appointment Calendar.");
}
public static Appointment makeAppointment(int selection) {
Appointment app=null;
String description="";
String lastName="";
int hours=0;
int minutes = 0;
int day=0;
int year=0;
int month=0;
sc.nextLine();
switch (selection) {
case 1:
Onetime onetime=new Onetime();
System.out.println("What is the description of your appointment?");
description = sc.nextLine();
System.out.println("What is your person's name?");
lastName = sc.nextLine();
System.out.println("What is the year of your appointment?(2017-2018)");
year = sc.nextInt();
System.out.println("What is the month of your appointment?(1-12)");
month = sc.nextInt();
System.out.println("What is the day of your appointment?(1-31)");
day = sc.nextInt();
System.out.println("What is the hour of your appointment?(1-31)");
hours = sc.nextInt();
System.out.println("What is the minutes of your appointment?(1-31)");
minutes = sc.nextInt();
onetime.setDescription(description);
onetime.setHours(hours);
onetime.setLastName(lastName);
onetime.setMonth(month);
onetime.setMinutes(minutes);
onetime.setYear(year);
onetime.setDay(day);
System.out.println("Appointment added with "+lastName+" on "+month+"/"+day+"/"+year+" at "+hours+":"+minutes);
System.out.println();
return onetime;
case 2:
Daily daily = new Daily();
System.out.println("What is the description of your appointment?");
description = sc.nextLine();
System.out.println("What is your person's name?");
lastName = sc.nextLine();
System.out.println("What is the hour of your appointment?(1-31)");
hours = sc.nextInt();
System.out.println("What is the minutes of your appointment?(1-31)");
minutes = sc.nextInt();
daily.setDescription(description);
daily.setHours(hours);
daily.setLastName(lastName);
daily.setMinutes(minutes);
System.out.println("Appointment added with "+lastName+" Daily at "+hours+":"+minutes);
System.out.println();
return daily;
case 3:
Monthly monthly = new Monthly();
System.out.println("What is the description of your appointment?");
description = sc.nextLine();
System.out.println("What is your person's name?");
lastName = sc.nextLine();
System.out.println("What day of the month is your appointment?(1-12)");
day = sc.nextInt();
System.out.println("What is the hour of your appointment?(1-31)");
hours = sc.nextInt();
System.out.println("What is the minutes of your appointment?(1-31)");
minutes = sc.nextInt();
monthly.setDescription(description);
monthly.setHours(hours);
monthly.setLastName(lastName);
monthly.setMinutes(minutes);
monthly.setDay(day);
System.out.println("Appointment added with "+lastName+" Monthly on day "+day+" at "+hours+":"+minutes);
System.out.println();
return monthly;
default:
System.out.println("Invalid Choice..!!");
return app;
}
}
public static boolean checkAppointment(Appointment a, int year, int month, int day) {
return a.occursOn(year, month, day);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
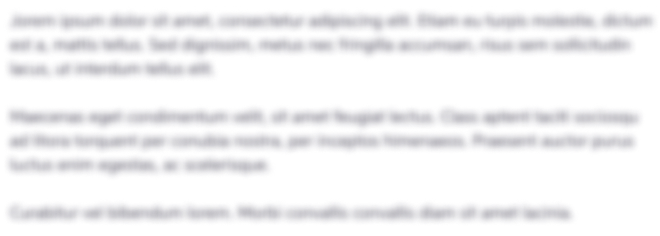
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started