Question
Create a very simple farm simulation in Java. The simulation will run in a loop and call a method that indicates the passage of time.
Create a very simple farm simulation in Java. The simulation will run in a loop and call a method that indicates the passage of time.
MUST USE A GENERIC CONTAINER such as ArrayList
The Generic it has must be some form of the base class ANIMAL such as: ArrayList farm = new ArrayList () ;
1. Create an Animal Class that will be the base class for all your animals: All animals have some level of hunger from 0-24.
2. When an animal is instantiated, it should randomly pick some number of hunger units between 0 and 24. (in the constructor)
3. Since it is a small farm, all animals have their own name.
4. All animals must be able to be fed (decrease their hunger units by 5 when they are fed).
5. Animals must increase their hunger as time goes on: create a method that increases their hunger by 1 unit of hunger.
6. In the loop example below tick() is used since the method relates to the virtual passing of time. While in this case all that happens is the hunger level increases other simulators might have much more complex effects.
7. When printed an animal should indicate. The animals name, the type of the animal and the animals hunger level: Spot the dog is very hungry.
8. If we are never going to instantiate an Animal directly what type of class could we make it? Explain? Put your answer in the comments at the top of the class.
Note: It is possible that when you run the simulation it will loop infinitely. Can you explain why? For larger sets of animals this would almost always happen. You could put this in the constructor: hungerUnits = hungerUnits - (hungerUnits%5); What does this do? Why does this stop infinite loops?
9. Create 5 subclasses of farmyard animals:
a. Each animal should also speak based on what type of animal it is and how much they speak should change with their hunger level. A cow might moo.
b. The calculation for the times speaking will be different for each subclass
c. The calculation for the first five animal subclasses should be:
i. First Animal Class: (Hunger Level + 1) * 2
ii. Second Animal Class: (Hunger Level + 1)
iii. Third Animal Class: ((Hunger Level / 2) + 1) * 2
iv. Fourth Animal Class: (Hunger Level * 3)
v. Fifth Animal Class: Hunger Level + 2
10.Create a class with a main method
a. That instantiates 2 of each or your animals and put them in an ArrayList. Sort the animals by their hunger level (most hungry to least hungry).
b. Allow each animal to speak, print it out and then feed it if it is not full. Continue this process until all of them are full.
11.Implement a sleeping feature.
Sleeping
All animals have a 10 percent chance of falling asleep when they are fed.
Side Effects of sleeping
1. Animals that are asleep do not decrease their hunger units when fed.
2. Animals that are asleep Snore when then speak instead of their normal vocalization.
3. Animals wake up if their Hunger Level changes
The Output from the simulator might look something like this for one feeding (Continue to feed until all animals are full):
bark bark bark bark
Spot the Dog is Very Hungry
cluck cluck cluck
Julie the Chicken is Hungry
moo moo
Jane the Cow is Peckish
cluck cluck
Mary the Chicken is Peckish
moo
Sarah the Cow is Full
woof
Bolt the Dog is Full
public static void main (String[] args) ArrayListStep by Step Solution
There are 3 Steps involved in it
Step: 1
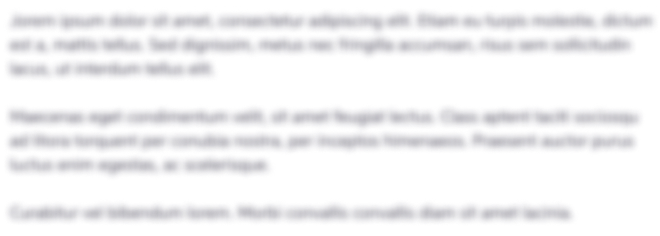
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started