Question
Create an application that can apply set operations to a set of values. Generics will be used to specify the type of values on which
Create an application that can apply set operations to a set of values. Generics will be used to specify the type of values on which the application should apply the set operations.
The applicaton will follow the S.O.L.I.D. design principles.
Interfaces
The ISet interface represents a set of non-duplicating values. Specify a generic type for the interface (
Methods of the ISet interface include Add(T) to add an element of type T to the set, AddAll(IEnumerable
The ISetFactory represents a ISet instantiator that creates ISet objects. There are two methods supported in this interface: CreateSet with no parameters that creates and returns an ISet object with no elements and CreateSet that takes an IEnumerable parameter and returns an ISet object initialized with elements from that parameter. The CreateSet methods must include a type parameter (
Classes
The Set class implements (supports) the ISet interface. A generic type (
The Set class must implement the Add, AddAll, SetUnion, SetIntersect, and SetDifference methods as described in the ISet interface. Each of the five methods should be overridable to allow for future extensions. The Set must also support the IEnumerable
The Set class should not use the IEnumerable
Finally, the Set class must override the ToString method to return a string that contains a comma-separated list for the elements of the set in square brackets (e.g. [element1, element2, element3, ..., elementn]).
The SetFactory class implements (supports) the ISetFactory interface. It implements the two CreateSet methods by calling the appropriate Set class constructors and returning the newly instantiated object.
The Application
z
Program.cs
The Program.cs file must create the ConSet object, the ISetFactory object and then call the ConSet.Run method specifying the type of data for the set. E.g.
ConSet conset = new ConSet();
ISetFactory setfactory = new SetFactory();
conset.Run
conset.Run
Sample Execution of the Application
Please enter the first set of Int32 values:
1
2
3
Please enter the second set of Int32 values:
3
5
7
[1, 2, 3] UNION [3, 5, 7] = [1, 2, 3, 5, 7]
[1, 2, 3] INTERSECT [3, 5, 7] = [3]
[1, 2, 3] DIFFERENCE [3, 5, 7] = [1, 2]
Please enter the first set of Double values:
1.1
2.2
3.3
Please enter the second set of Double values:
3.3
5.5
7.7
[1.1, 2.2, 3.3] UNION [3.3, 5.5, 7.7] = [1.1, 2.2, 3.3, 5.5, 7.7]
[1.1, 2.2, 3.3] INTERSECT [3.3, 5.5, 7.7] = [3.3]
[1.1, 2.2, 3.3] DIFFERENCE [3.3, 5.5, 7.7] = [1.1, 2.2]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
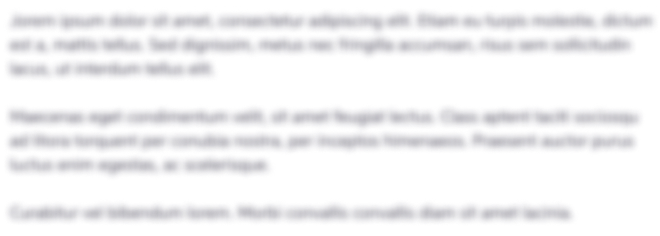
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started