Question
- Create an array of structure which will be used to store student information. - The size of array of structure should be 15, but
- Create an array of structure which will be used to store student information.
- The size of array of structure should be 15, but will only be partially filled.
- Structure to be used
struct StudentInfo
{
string firstName;
int id;
unsigned long int phoneNum;
string homeState;
int birthYear;
double gpa;
};
- Read in student information from an input file (file name: lab2_input.txt) and store the information in the array of structure.
- Input file format:
Bob 12345 4193215984 OH 1988 3.60 Will 32158 8052584455 FL 1998 3.66
- Assume that we don't know the number of students shown in the file. (But it will be less than 15.)
- After reading in all the information, create and call a function (function name: showStudentInfo( )) that displays the student information as shown below.
(Hint: function prototype to be used
void showStudentInfo(const StudentInfo [], int numEle); ) NAME ID PHONE STATE Birth Year GPA Bob 12345 4193215984 OH 1988 3.60 Will 32158 8052584455 FL 1998 3.66 - Create and call a function (function name: findOldestStudent( )) that returns the oldest student information. The function should return a structure. The return structure information should be displayed on the screen.
(Hint: function prototype to be used StudentInfo findOldestStudent(const StudentInfo [], int numEle); )
#include
#include
#include
#include
using namespace std;
struct StudentInfo
{
string firstName;
int id;
unsigned long int phoneNum;
string homeState;
int birthYear;
double gpa;
};
void showStudentInfo(const StudentInfo []);
StudentInfo findOldestStudent(const StudentInfo []);
int main();
{
const int STUDENT_SIZE = 15;
StudentInfo stuList[STUDENT_SIZE];
int num_students = 0;
ifstream File;
File.open("lab02_input.txt");
while (!File.eof())
{
File >> stuList[num_students].firstName;
File >> stuList[num_students].id;
File >> stuList[num_students].phoneNum;
File >> stuList[num_students].homeState;
File >> stuList[num_students].birthYear;
File >> stuList[num_students].gpa;
num_students++;
}
showStudentInfo(StudentInfo, num_students);
}
void showStudentInfo(const StudentInfo[], int num_students)
{
int num = 0;
cout << "----" << "Name" << "----" << "ID" << "----" << "Phone State" << "----" << "Birth Year" << "----" << "GPA" << endl;
for (num = 0; num < num_students; num++);
{
cout << "----" << stuList[STUDENT_SIZE].firstName << "----" << stuList[STUDENT_SIZE].id << "----" << stuList[STUDENT_SIZE].phoneNum << "----" << stuList[STUDENT_SIZE].homeState << "----" << stuList[STUDENT_SIZE].birthYear << "----"
<< stuList[STUDENT_SIZE].gpa << "----" << endl;
}
}
struct findOldestStudent(const StudentInfo[], const int STUDENT_SIZE, int num_students)
{
Please tell me how should I go futher that this.
And then:-
* After the oldest student information is displayed on the screen, create and call a function (function name: sortStudentID( )) that sort the student information by the student ID (i.e., in increasing order).
* Hint: function prototype to be used --> void sortStudentID(StudentInfo [ ], int);
* Then, display the contents of the student information using showStudentInfo( ) (which was a part of the Lab 02 assignment).
THE ABOVE ONE IS LAB 2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
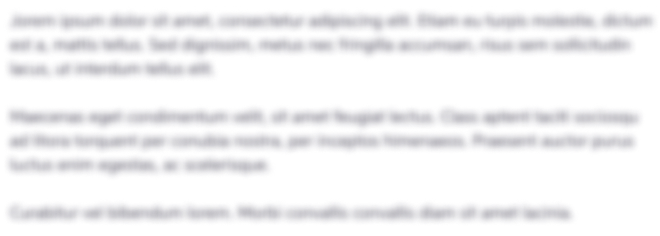
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started