Question
Create full database SQL code for the following classes. And also edit all the classes code to be able to save the database after entering
Create full database SQL code for the following classes. And also edit all the classes code to be able to save the database after entering input.
Spam and fake or incomplete answers will be reported to the advanced advocates!! be respectful
Hospital Management Sys
class Hospital
{
private string name;
private string address;
private string phoneNumber;
private List
private List
public Hospital(string name, string address, string phoneNumber)
{
this.name = name;
this.address = address;
this.phoneNumber = phoneNumber;
doctors = new List
patients = new List
}
public void addDoctor(Doctor doctor)
{
doctors.Add(doctor);
}
public void removeDoctor(Doctor doctor)
{
doctors.Remove(doctor);
}
public void addPatient(Patient patient)
{
patients.Add(patient);
}
public void removePatient(Patient patient)
{
patients.Remove(patient);
}
public Doctor getDoctor(string name)
{
return doctors.Find(x => x.name == name);
}
public Patient getPatient(string name)
{
return patients.Find(x => x.name == name);
}
public List
{
return doctors;
}
public List
{
return patients;
}
}
class Doctor
{
public string name;
public string specialty;
private List
public Doctor(string name, string specialty)
{
this.name = name;
this.specialty = specialty;
appointments = new List
}
public void addAppointment(Appointment appointment)
{
appointments.Add(appointment);
}
public void removeAppointment(Appointment appointment)
{
appointments.Remove(appointment);
}
public Appointment getAppointment(string date)
{
return appointments.Find(x => x.date == date);
}
public List
{
return appointments;
}
public void diagnose(Patient patient)
{
// diagnose patient
}
public void prescribe(Patient patient, Medicine medicine)
{
// prescribe medicine to patient
}
}
class Accountant
{
public string name;
private double salary;
private List
public Accountant(string name, double salary)
{
this.name = name;
this.salary = salary;
expenses = new List
}
public void addExpense(Expense expense)
{
expenses.Add(expense);
}
public void removeExpense(Expense expense)
{
expenses.Remove(expense);
}
public Expense getExpense(string description)
{
return expenses.Find(x => x.description == description);
}
public List
{
return expenses;
}
public double calculateProfit()
{
// calculate profit
}
}
class Receptionist
{
public string name;
private double salary;
private List
public Receptionist(string name, double salary)
{
this.name = name;
this.salary = salary;
appointments = new List
}
public void addAppointment(Appointment appointment)
{
appointments.Add(appointment);
}
public void removeAppointment(Appointment appointment)
{
appointments.Remove(appointment);
}
public Appointment getAppointment(string date)
{
return appointments.Find(x => x.date == date);
}
public List
{
return appointments;
}
public void checkIn(Patient patient)
{
// check in patient
}
public void checkOut(Patient patient)
{
// check out patient
}
}
class Patient
{
public string name;
public int age;
public string gender;
private List
private List
public Patient(string name, int age, string gender)
{
this.name = name;
this.age = age;
this.gender = gender;
appointments = new List
prescriptions = new List
}
public void addAppointment(Appointment appointment)
{
appointments.Add(appointment);
}
public void removeAppointment(Appointment appointment)
{
appointments.Remove(appointment);
}
public Appointment getAppointment(string date)
{
return appointments.Find(x => x.date == date);
}
public List
{
return appointments;
}
public void addPrescription(Prescription prescription)
{
prescriptions.Add(prescription);
}
public void removePrescription(Prescription prescription)
{
prescriptions.Remove(prescription);
}
public Prescription getPrescription(string medicine)
{
return prescriptions.Find(x => x.medicine == medicine);
}
public List
{
return prescriptions;
}
}
class Appointment
{
public string date;
public string time;
public Doctor doctor;
public Patient patient;
public Appointment(string date, string time, Doctor doctor, Patient patient)
{
this.date = date;
this.time = time;
this.doctor = doctor;
this.patient = patient;
}
public string getDate()
{
return date;
}
public string getTime()
{
return time;
}
public Doctor getDoctor()
{
return doctor;
}
public Patient getPatient()
{
return patient;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
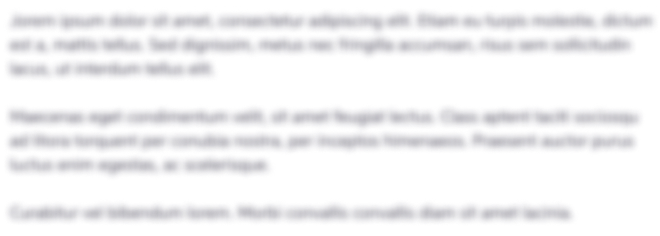
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started