Question
Create the following list methods (all indices are 0-based) - T get (in index), returns the value in the list at the specified index. throw
Create the following list methods (all indices are 0-based) - T get (in index), returns the value in the list at the specified index. throw a java.lang.RuntimeException if index is invalid. - void set(int index, T value), changes the list item at position index to value. throw a java.lang.RuntimeException if index is invalid - void add(T value), inserts value into the list at the end. - void add(int index, T value), inserts value at position index. throw a java.lang.RuntimeException if index is invalid - void remove(int index), remove the item in the list at position index. throw a java.lang.RuntimeException if index is invalid - boolean contains(T value), returns true if the list contains value. returns false otherwise
Here is the following code I have so far (there is a sample main at the end):
import java.util.Iterator;
public class SList
{
private class Node
{
T value;
Node next;
public T data;
}
private Node head;
public void addFirst(T v)
{
Node n = new Node();
n.value = v;
n.next = head;
head = n;
}
public void removeFirst()
{
head = head.next;
}
public int size()
{
int count=0;
Node curs = head;
while (curs != null)
{
count++;
curs=curs.next;
}
return count;
}
public T get (int index)
{
if (index < 0 || index >= size())
{
throw new RuntimeException("Index Is Out Of Bounds!");
}
else
{
Node temp = head;
for (int i = 0; i < index; i++)
{
temp = temp.next;
}
return temp.data;
}
}
private class SLIterator implements Iterator
{
private Node n;
public SLIterator()
{
n = null;
}
public boolean hasNext()
{
if (n == null)
{
return head != null;
}
else
{
return n.next != null;
}
}
public T next()
{
if (n == null)
{
n = head;
}
else
{
n = n.next;
}
return n.value;
}
}
public Iterator
{
return new SLIterator();
}
}
/* Expected output of this program: 0 [(adding)size=1;0] 1 0 [(adding)size=2;1] 2 1 0 [(adding)size=3;2] 3 2 1 0 [(adding)size=4;3] 4 3 2 1 0 [(adding)size=5;4] 5 4 3 2 1 0 [(adding)size=6;5] 6 5 4 3 2 1 0 [(adding)size=7;6] 7 6 5 4 3 2 1 0 [(adding)size=8;7] 8 7 6 5 4 3 2 1 0 [(adding)size=9;8] 9 8 7 6 5 4 3 2 1 0 [(adding)size=10;9] size: 10 8 7 6 5 4 3 2 1 0 [(removing)size=9;8] 7 6 5 4 3 2 1 0 [(removing)size=8;7] 6 5 4 3 2 1 0 [(removing)size=7;6] 5 4 3 2 1 0 [(removing)size=6;5] 4 3 2 1 0 [(removing)size=5;4] 3 2 1 0 [(removing)size=4;3] 2 1 0 [(removing)size=3;2] 1 0 [(removing)size=2;1] 0 [(removing)size=1;0] */ public class SampleMain { public static void main(String[] args) { SListlst = new SList<>(); for (int i=0; i<10; i++) { lst.addFirst(i); for (Integer val : lst) { System.out.print(val + " "); } System.out.println("[(adding)size="+lst.size()+";"+lst.get(0)+"]"); } System.out.println("size: " + lst.size()); while (lst.size() > 1) { lst.removeFirst(); for (Integer val : lst) { System.out.print(val + " "); } System.out.println("[(removing)size="+lst.size()+";"+lst.get(0)+"]"); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
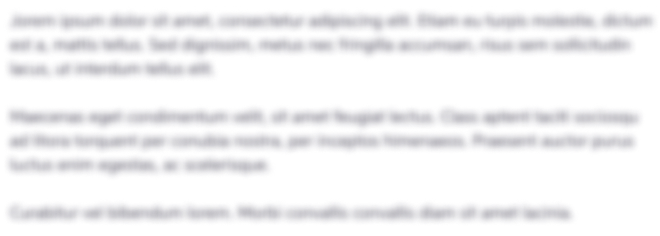
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started