Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Create the following simplified Customer class : public class Customer { String name ; int age ; char gender ; float money ; // A
Create the following simplified Customer class: public class Customer { String name; int age; char gender; float money; // A simple constructor public Customer(String n, int a, char g, float m) { name = n; age = a; gender = g; money = m; } // Return a String representation of the object public String toString() { String result; result = "Customer " + name + ": a " + age + " year old "; if (gender == 'F') result += "fe"; return result + "male with $" + money; } }
public class Store { public static final int MAX_CUSTOMERS = 500; String name; Customer[] customers; int customerCount; public Store(String n) { name = n; customers = new Customer[MAX_CUSTOMERS]; customerCount = 0; } public void addCustomer(Customer c) { if (customerCount < MAX_CUSTOMERS) customers[customerCount++] = c; } public void listCustomers() { for (int i=0; i<customerCount; i++) System.out.println(customers[i]); } }
public class StoreTestProgram { public static void main(String args[]) { Customer[] result; Store walmart; walmart = new Store("Walmart off Innes"); walmart.addCustomer(new Customer("Amie", 14, 'F', 100)); walmart.addCustomer(new Customer("Brad", 15, 'M', 0)); walmart.addCustomer(new Customer("Cory", 10, 'M', 100)); walmart.addCustomer(new Customer("Dave", 5, 'M', 48)); walmart.addCustomer(new Customer("Earl", 21, 'M', 500)); walmart.addCustomer(new Customer("Flem", 18, 'M', 1)); walmart.addCustomer(new Customer("Gary", 8, 'M', 20)); walmart.addCustomer(new Customer("Hugh", 65, 'M', 30)); walmart.addCustomer(new Customer("Iggy", 43, 'M', 74)); walmart.addCustomer(new Customer("Joan", 55, 'F', 32)); walmart.addCustomer(new Customer("Kyle", 16, 'M', 88)); walmart.addCustomer(new Customer("Lore", 12, 'F', 1000)); walmart.addCustomer(new Customer("Mary", 17, 'F', 6)); walmart.addCustomer(new Customer("Nick", 13, 'M', 2)); walmart.addCustomer(new Customer("Omar", 18, 'M', 24)); walmart.addCustomer(new Customer("Patt", 24, 'F', 45)); walmart.addCustomer(new Customer("Quin", 42, 'M', 355)); walmart.addCustomer(new Customer("Ruth", 45, 'F', 119)); walmart.addCustomer(new Customer("Snow", 74, 'F', 20)); walmart.addCustomer(new Customer("Tamy", 88, 'F', 25)); walmart.addCustomer(new Customer("Ulsa", 2, 'F', 75)); walmart.addCustomer(new Customer("Vern", 9, 'M', 90)); walmart.addCustomer(new Customer("Will", 11, 'M', 220)); walmart.addCustomer(new Customer("Xeon", 17, 'F', 453)); walmart.addCustomer(new Customer("Ying", 19, 'F', 76)); walmart.addCustomer(new Customer("Zack", 22, 'M', 35)); 3 System.out.println("Here are the customers: "); walmart.listCustomers(); } }
---Write a method in the Store class called averageCustomerAge() which should return an int representing the average age of all Customer objects in the store. Test your code by appending the following line to the end of the StoreTestProgram:. You should get a result of 26. // Find average Customer age System.out.println(" Average age of customers: " + walmart.averageCustomerAge()); --- Write a method in the Store class called mostPopularGender() which should return a char representing the most popular gender of all Customer objects in the store (i.e., 'M' or 'F'). Test your code by appending the following lines to the end of the StoreTestProgram. The answer should be M. // Find most popular gender System.out.println(" Most popular gender is: " + walmart.mostPopularGender()); ---Write a method in the Store class called richestCustomer() which should return a Customer object representing the Customer in the store who has the most money. Make sure to return the whole Customer object, not just the name. Test your code by appending the following lines to the end of the StoreTestProgram. The result should be Lore who has $1000: // Find richest customer System.out.println(" Richest customer is: " + walmart.richestCustomer());
Write the following two methods in the Store class. public void changeGender(Customer c) { if (c.gender == 'M') c.gender = 'F'; else c.gender = 'M'; } public void rob(Customer c) { c.money = 0; } Then test your code by appending the following lines to the end of the StoreTestProgram: // Change Zack's gender while he is shopping at the Store walmart.changeGender(walmart.customers[25]); System.out.println(" Zack is now: " + walmart.customers[25].gender); // Continue to make Zack's walmart experience quite miserable walmart.rob(walmart.customers[25]); System.out.println(" Zack is now: " + walmart.customers[25]);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
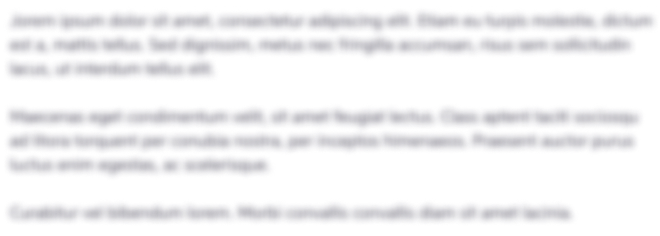
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started