Question
Create the method public void draw(Graphic2D g2) in circle.java and octagon.java . This method should draw the shape at the location given by the instance
Create the method public void draw(Graphic2D g2) in circle.java and octagon.java. This method should draw the shape at the location given by the instance data x and y. The shape should be drawn in the color specified by the color instance data, and if the shape should be filled with the color, the draw method should fill the shape.
Create a class called ShowShapes with a main method. In the main method create a Frame that is 500 by 500. Set the usual properties of the frame.
Create a ShapeComponent class that extends JComponent. Create the paintComponent method and make it draw a row of Octagons that alternate between being filled and being empty. Then draw a row of colored circles that alternate between being filled and being empty. Add a shapeComponent to the frame created in the previous exercise.
Create a new subclass of GeometricShape called Star. Implement the usual members for the class. Create a draw method that draws a 5 sided star at the location given by the x and y instance data. The star should be drawn in the color specified by the color instance data, and if the star should be filled with the color, the draw method should fill the star. Then add a row of stars to the ShapeComponent. The stars should be randomly colored and alternate between being filled and unfilled.
--------------------------------------------------------------------------------------
abstract class GeometricShape implements Comparable
private Color color; private boolean isFilled; private int x, y;
public GeometricShape() { this.color = Color.WHITE; this.isFilled = true;
}
public GeometricShape(Color color, int shape, int x, int y) { this.color = color; this.isFilled = true; this.x = x; this.y = y; }
public static GeometricShape max(GeometricShape s1, GeometricShape s2) {
if (s1.getArea() == s2.getArea()) return s1;
else if (s1.getArea() > s2.getArea()) return s1;
else return s2; }
public static double sumArea(GeometricShape[] shapes) {
double sum = 0;
for (int i = 0; i < shapes.length; i++) sum += shapes[i].getArea();
return sum; }
public int compareTo(GeometricShape a) {
if (this.getArea() <= a.getArea()) return -1;
return 1; }
public Color getColor() { return color; }
public void setColor(Color color) { this.color = color; }
public boolean isFilled() { return isFilled; }
public int getX() { return x; }
public void setX(int x) { this.x = x; }
public int getY() { return y; }
public void setY(int y) { this.y = y; }
@Override public String toString() { return ("color= " + color + ", isFilled=" + isFilled + ", Area: " + getArea() + "Perimeter: " + getPerimeter() + " "); }
public abstract double getArea();
public abstract double getPerimeter();
} --------------------------------------------------------------------------------------------
public abstract class Circle extends GeometricShape {
private double radius; private Color color; private int x, y;
public Circle(double radius, Color color, int x, int y) {
this.radius = radius; this.color = color; this.x = x; this.y = y;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public Color getColor() { return color; }
public void setColor(Color color) { this.color = color; }
public int getX() { return x; }
public void setX(int x) { this.x = x; }
public int getY() { return y; }
public void setY(int y) { this.y = y; }
@Override public double getArea() {
return Math.PI * radius * radius;
}
@Override public double getPerimeter() {
return 2 * Math.PI * radius;
}
public String toString() {
return "Shape: Circle" + "Radius: " + radius + "Area: " + getArea() + "Perimeter: " + getPerimeter() + " ";
} }
------------------------------------------------------------------------------------------
public abstract class Octagon extends GeometricShape {
private int side; private Color color; private int x, y;
public Octagon(int side, Color color, int x, int y) {
this.side = side; this.color = color; this.x = x; this.y = y;
}
public double getSide() {
return side;
}
public void setSide(int side) {
this.side = side;
}
public Color getColor() { return color; }
public void setColor(Color color) { this.color = color; }
public int getX() { return x; } public void setX(int x) { this.x = x; }
public int getY() { return y; }
public void setY(int y) { this.y = y; }
@Override public double getArea() {
return 2 * (1 + Math.sqrt(2)) * side * side;
}
@Override public double getPerimeter() {
return 8 * side;
}
public String toString() {
return "Shape: Octagon" + "Side: " + side + "Area: " + getArea() + "Perimeter: " + getPerimeter() + " ";
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
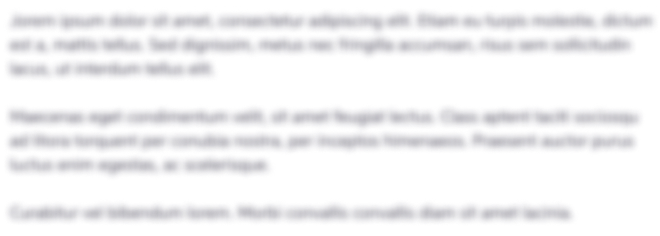
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started