Question
Create this assignment below using Java. Follow the rules below and create the required classes for a good rate. Contents class BookStore Sample BookStore Run
Create this assignment below using Java.
Follow the rules below and create the required classes for a good rate.
Contents
class BookStore Sample BookStore Run enum BookType class ShoeStore Sample ShoeStore Run enum ShoeType class Store class Address class Book class Author class Date class Name class Shoe enum Material class Item
-----------------------------------------------------------------------------
The purpose of this assignment is to create two Stores: a book store (class BookStore) and a shoe store (class ShoeStore).
class BookStore
This is a subclass of Store. It also has an instance variable for specialty, which is an enum of the following possible values: FICTION, NONFICTION, SCIENCEFICTION, REFERENCE
Note that we can map these enums to Strings (and vice versa) as follows in the BookType enum (see below):
import java.util.Map; import java.util.HashMap;
public enum BookType { FICTION("fiction"), NONFICTION("nonfiction"), SCIENCEFICTION("sciencefiction"), REFERENCE("reference");
private String theBookType;
private static Map
static { for (BookType b : BookType.values()) { lookup.put(b.getTheBookType(), b); } }
private BookType(String theBookType) { this.theBookType = theBookType; }
public String getTheBookType() { return theBookType; }
// the following method allows me to create a BookType enum from a String! // e.g. in the Book class, I could set the BookType instance variable to: // bookType = BookType.get("fiction"); public static BookType get(String theBookType) { return lookup.get(theBookType); } }
Provide an overloaded constructor: one accepts Address, String, and BookType parameters for address, name, and specialty, respectively; the other accepts Address, String, and String parameters for address, name, and specialty, respectively example: this.specialty = BookType.get(specialty).
Both constructors then call an addBooks() method which creates anonymous objects and adds the following books to its (parents) HashMap. The key is the Book Items uniqueID String; and the value is the Book.
The BookStore must provide the following methods. One of the methods has been filled in for an example.
import java.util.Collection; import java.util.Iterator;
class BookStore extends Store { private BookType specialty;
public BookStore(Address address, String name, BookType specialty) { super(address, name); this.specialty = specialty; addBooks(); }
public BookStore(Address address, String name, String specialty) { super(address, name); this.specialty = BookType.get(specialty); addBooks(); }
private void addBooks() { Date birthDate = new Date(1919, 1, 1); Name name = new Name("Jerome", "David", "Salinger"); BookType genre = BookType.get("fiction"); Author author = new Author(birthDate, name, genre, "JD"); Date datePublished = new Date(1951, 5, 14); String title = "The Catcher in the Rye"; Book b = new Book(0.4, 2.0, 4.0, "1234", author, datePublished, title, genre); addItem(b);
datePublished = new Date(1948, 1, 31); title = "A Perfect Day for Bananafish"; genre = BookType.get("fiction"); b = new Book(1, 11, 12, "2345", author, datePublished, title, genre); addItem(b);
datePublished = new Date(1945, 12, 12); title = "A Boy in France"; genre = BookType.get("fiction"); b = new Book(2, 33, 35, "3456", author, datePublished, title, genre); addItem(b);
birthDate = new Date(1963, 9, 3); name = new Name("Malcolm", "Gladwell"); genre = BookType.get("nonfiction"); author = new Author(birthDate, name, genre); datePublished = new Date(2008, 11, 18); title = "Outliers"; b = new Book(2.1, 2, 6, "4567", author, datePublished, title, genre); addItem(b);
datePublished = new Date(2000, 3, 1); title = "The Tipping Point"; genre = BookType.get("nonfiction"); b = new Book(0.5, 3, 5, "5678", author, datePublished, title, genre); addItem(b);
birthDate = new Date(1919, 11, 26); name = new Name("Frederik", "Pohl"); genre = BookType.get("sciencefiction"); author = new Author(birthDate, name, genre, "Paul Dennis Lavond"); datePublished = new Date(1977, 7, 4); title = "Gateway"; b = new Book(0.01, 4, 4, "6789", author, datePublished, title, genre); addItem(b);
datePublished = new Date(1937, 10, 6); title = "Elegy to a Dead Planet: Luna"; genre = BookType.get("sciencefiction"); b = new Book(0.1, 5, 11, "abcd", author, datePublished, title, genre); addItem(b);
birthDate = new Date(1918, 5, 11); name = new Name("Richard", "Phillips", "Feynman"); genre = BookType.get("reference"); author = new Author(birthDate, name, genre); datePublished = new Date(1942, 5, 20); title = "Principle of Least Action in Quantum Mechanics"; b = new Book(0.8, 15, 30, "efgh", author, datePublished, title, genre); addItem(b);
datePublished = new Date(1964, 6, 30); title = "The Messenger Lectures"; genre = BookType.get("reference"); b = new Book(0.6, 44, 45.5, "ijkl", author, datePublished, title, genre); addItem(b);
datePublished = new Date(1985, 11, 1); title = "Surely You're Joking Mr. Feynman"; genre = BookType.get("nonfiction"); b = new Book(1.0, 3, 13, "mnop", author, datePublished, title, genre); addItem(b); }
public void displayAllBooksWrittenByAuthorsOverThisAge(int ageInYears) { Collection
all other methods go here too... }
Sample BookStore Run
Test a BookStore named Chapters, with an address of 1234 Main Street, Vancouver, A3A A4A, and a specialty of fiction.
The following methods needs to be provided for the Bookstore class:
a) displayAllBooksByEveryAuthor() b) displayAllBooksByAuthor(String lastName) c) displayAllBooksWrittenBefore(int year) d) displayTitlesOfBooksWrittenBy(String pseudonym) e) displayAllBooksForGenre(String genre) f) displayTotalWeightKgOfAllBooks() g) displayAllBooksWrittenByAuthorsOverThisAge(int ageInYears) h) displayAllBooksWrittenByAuthorsBornOn(String dayOfTheWeek) i) displayAllBooksPublishedOn(String dayOfTheWeek) j) displayAllBooksWrittenByAuthorsWithAPseudonym() k) displayBookWithBiggestPercentageMarkup() l) displayAllBooksWrittenOutsideSpecialty()
enum BookType see above
class ShoeStore This is a subclass of Store. It also has an instance variable for department, which is an enum of the following values: WOMEN, MEN, CHILDREN, SPORTS, DRESS (created similarly to BookType, above).
The ShoeStore class must have similar functionality as the BookStore (above). Its instance variables are:
Department (see above).
The inventory that will be created in the addShoes() method is as follows:
Kg | Manuf Price $ | Retail Price $ | ID | Designer | Size | Material | Color | Department |
1 | 58.5 | 90 | Diameter | Skechers | 10 | LEATHER | DARK_GRAY | men |
1.15 | 104 | 160 | Wave | Robert Cobbler | 12 | LEATHER | BLACK | dress |
1 | 110.5 | 170 | Monet | Geox | 7 | CLOTH | BLUE | men |
0.85 | 84.5 | 130 | Camya Multi Glitter | Nine West | 8 | PLASTIC | BLACK | women |
0.9 | 97.5 | 150 | Marieclaire | Geox | 10 | PLASTIC | GRAY | women |
0.6 | 45.5 | 70 | Balance Of The Force | Stride Rite | 9 | RUBBER | GRAY | children |
0.7 | 39 | 60 | Top-Sider Unisex Bluefish H&L | Sperry | 9 | CLOTH | ORANGE | children |
0.85 | 32.5 | 50 | Lite Kicks Rainbow Sprite | Skechers | 10 | PLASTIC | PINK | children |
0.5 | 39 | 60 | Toachi | Robert Cobbler | 5 | CLOTH | BLUE | children |
1.2 | 117 | 180 | Jordan Ace 23 II | Nike | 13 | RUBBER | WHITE | sports |
This classs methods must have the same sort of output as the BookStore methods.
displayAllShoesAndDesigners() displayAllShoesByDesigner(String designerName) displayAllShoesMadeOf(Material material) displayAllShoesMadeOf(String material) displayNumberOfShoesDesignedBy(Name designer) displayNumberOfShoesDesignedBy(String designerLastName) displaySmallestShoeSize () displayTotalWeightKgOfAllShoes() displayAllShoesOfThisMaterialMadeByThisDesigner(Material m, Name designer) displayAllShoesNotInMatchingStore() // e.g. For a shoe store with department WOMEN, show all the shoes of type MEN, CHILDREN, SPORTS, and DRESS
Sample ShoeStore Run Test a ShoeStore named My Shoes, with an address of 789 East 1st Street, West Vancouver, V3A 7A4, which specializes in selling childrens shoes.
enum ShoeType see ShoeStore, above, for the enums
class Store This is the parent class of all other Stores.
It has the following instance variables:
A streetAddress, of class Address. A name, of class String.
The store contains a HashMap of Items. The key of each item is its uniqueID String. For example, an Item may have a uniqueID of abc123. The value is the item itself.
This class offers very useful, generic methods such as:
public void addItem(Item item){ itemsForSale.put(item.getUniqueID(), item); } public Item getItemByKey(String key){ return itemsForSale.get(key); } public Collection getCollectionOfItems(){ return itemsForSale.values(); }
class Address Contains instance variables for street number (String), street name (String), city (String), postal code (String).
class Book Has instance variables for author (class Author), datePublished (class Date), title (String), genre (bookType enum). Note that a Book also has an ISBN (a String). In our class we will NOT have an ISBN instance variable. Our Book class in fact uses its parent classs uniqueID variable and methods: e.g. class Book has methods called setISBN() and getISBN(), but in turn it just calls setUniqueID() and getUniqueID() from the Item class. This class also has a getYearPublished() convenience function, as well as getAuthorFullName(), and getGenreString() which returns a String such as fiction when its BookType is BookType.FICTION (return genre.getTheBookType();). Book is a subclass of class Item.
class Author Has instance variables for birthDate (class Date), name (class Name), genre (enum BookType), and pseudonym (String).
class Date Has instance variables for year (int), month (int), dayOfTheMonth (int). Has accessor methods for each, plus getMonthName( ) (e.g. returns January), getSuffix(e.g returns st for 1, nd for 2, rd for 3, th for 4, etc), and getDayOfWeek() (e.g. returns Friday). Here is an algorithm to get the day of the week from a date:
Example date: May 17th, 1989
Step 1: determine how many twelves fit in the last two digits of the year e.g. there are 7 twelves in 89 (12 * 7 = 84) Sum is 7
Step 2: determine the remainder from step 1 e.g. 89 84 = 5 remainder Sum is 7 + 5
Step 3: determine how many fours fit into the remainder from step 2
e.g. 1 four fits into five Sum is 7 + 5 + 1
Step 4: Add the date of the month e.g. 17 for the 17th Sum is 7 + 5 + 1 + 17
Step 5: Add the month code (see below) e.g. 2 for May Sum is 7 + 5 + 1 + 17 + 2
Step 6: Mod the sum by 7 7 + 5 + 1 + 17 + 2 = 32; 32 % 7 = 4 = WEDNESDAY
Match this number with the day of the week:
Saturday | Sunday | Monday | Tuesday | Wednesday | Thursday | Friday |
0 | 1 | 2 | 3 | 4 | 5 | 6 |
Month codes:
January: 1 | February: 4 | March: 4 |
April: 0 | May: 2 | June: 5 |
July: 0 | August: 3 | September: 6 |
October: 1 | November: 4 | December: 6 |
For dates in the 1700s, add 4. For dates in the 1800s, add 2. For dates in the 1900s, add nothing. For dates in the 2000s, add 6. For any other years, simply return Dunno.
Add 6 for January or February of a leap year.
The mutators do not permit invalid dates such as February 30th.
class Name Has instance variables for first name (String), middle name (String), last name (String). Overload the constructor to allow for people that have one (e.g. Madonna or Cher), or two (e.g. Tiger Woods), or three names (e.g. Andrew Lloyd Webber).
In addition to all regular accessor and mutator methods, include a method getFullName() which returns the full name (such as Madonna, or Tiger Woods, or Andrew Lloyd Webber).
class Shoe Has instance variables for material (enum Material), size (int), designer (Name class; e.g. Nike or Manolo Blahnik), shoe type (enum, and color (class java.awt.Color; for example, java.awt.Color.gray). Shoe is a subclass of class Item. Note that a Shoe also has a description (a String). In our class we will NOT have an description instance variable. Our Shoe class in fact uses its parent classs uniqueID variable and methods: e.g. class Shoe has methods called setDescription() and getDescription(), but in turn it just calls setUniqueID() and getUniqueID() from the Item class.
enum Material Has values for PLASTIC, LEATHER, RUBBER, CLOTH
class Item Has instance variables for weightKg (double), manufacturingPriceDollars (double), suggestedRetailPriceDollars (double), uniqueID (String).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
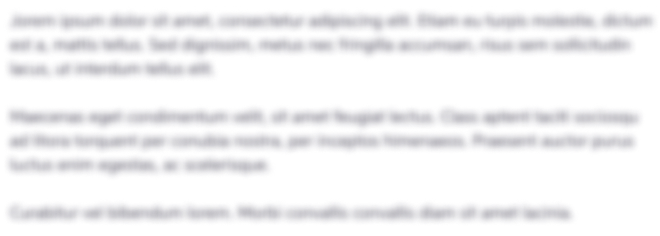
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started