Question
Create two classes that implement a Game interface: There is a Game interface defined in the Week 11 Source Code folder, and also a PlayGames
Create two classes that implement a Game interface:
There is a Game interface defined in the Week 11 Source Code folder, and also a PlayGames class that uses objects from classes that implement that interface. The Game interface declares a single abstract method play that returns an int, the score from playing the game, where bigger is better.
PlayGames creates and stores Game objects in a Game[] and uses a popRandom method to randomly choose one of the games to play next by running that objects play method.
There is an example of one such game, AdditionGame, that implements the Game interface. PlayGames creates an AdditionGame object and stores it in the Game[] array.
You must write at least 2 more classes that implement Game, store objects from those classes in the Game[] array in PlayGames, and run PlayGames to test that they work. You will test them manually by interacting with PlayGames, not by writing specific tests in a main method.
IN JAVA:
/// Starting point for Game Interface Lab.
import java.util.*;
public class PlayGames
{
public static final Scanner170 in = new Scanner170(System.in);
private static Random rand = new Random(1); // so Random always generates the same numbers
private static int gameCount = 0;
public static Game popRandom(Game[] g)
{
int n = gameCount;
int i = rand.nextInt(n);
Game ret = g[i];
g[i] = g[n-1];
gameCount--;
return ret;
}
public static void main(String[] args)
{
Game[] games = new Game[10]; // Note Game as a type
games[gameCount] = new AdditionGame(rand, 100);
gameCount++; // next index to put a Game at
// write at least 2 more different types of Game classes
// and add a new one of each type to games
// ...
/* for this exercise, write a SubtractionGame and a
MultiplicationGame by copying and modifying the
AdditionGame below - the SubtractionGame should be
constructed with 100 as the maximum number, like
AdditionGame, and the MultiplicationGame should be
constructed with 20 as the maximum number */
/* add those two games to the games array here, in that order */
int totScore = 0;
do {
Game g = popRandom(games);
totScore += g.play(); // use numerical result from the game
} while (gameCount > 0 && agree("Want a game? "));
System.out.println("Thanks for Playing!");
System.out.println("Your total score is " + totScore);
}
public static boolean agree(String prompt)
{
System.out.print(prompt);
String input = in.next();
if (input.equalsIgnoreCase("y")) return true;
return false;
}
}
/* DO NOT MARK THIS INTERFACE PUBLIC */
interface Game // Note *interface* in place of *class*
{
/// play the game and return the final score
/// where a higher score should be better,
/// and a negative score is allowed.
int play(); // Note semicolon in place of a body
// You can have multiple method headings declared
}
/* DO NOT MARK THIS CLASS PUBLIC */
class AdditionGame implements Game // note implements!!
{
private Random rand;
private int n;
// Constructor for objects of class AdditionGame
public AdditionGame(Random r, int big)
{
rand = r;
n = big;
}
// play all games and keep score.
public int play() // exactly matches heading in Game interface
{
final int numGames = 3;
Scanner in = PlayGames.in;
int score = 0;
System.out.println("Welcome to the addition game! We'll now play " + numGames + " rounds.");
for (int i = 0; i < numGames; i++) {
int x = rand.nextInt(n), y = rand.nextInt(n), ans = x+y;
System.out.print(String.format("Enter the sum: %d + %d = ", x, y));
int val = in.nextInt();
if (ans == val) {
System.out.println("Correct!");
score++;
}
else
System.out.println("Wrong! Right answer is " + ans);
}
System.out.println("Thanks for playing the addition game. Your score is " + score + ".");
System.out.println();
return score;
}
}
/* DO NOT MARK THIS CLASS PUBLIC */
class SubtractionGame implements Game // note implements!!
{
/* copy the contents of AdditionGame here and modify it so that
it becomes the SubtractionGame - it should also play 3 rounds */
}
/* DO NOT MARK THIS CLASS PUBLIC */
class MultiplicationGame implements Game // note implements!!
{
/* copy the contents of AdditionGame here and modify it so that
it becomes the MultiplicationGame - it should also play 3 rounds */
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
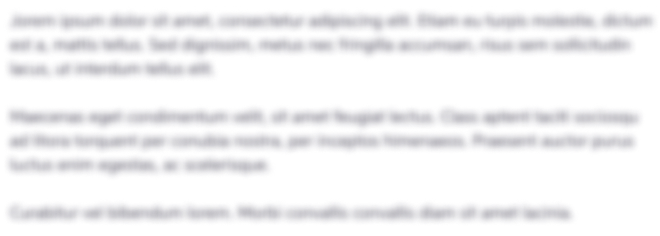
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started