Answered step by step
Verified Expert Solution
Question
1 Approved Answer
. Create two files: linkedlist.py and test_linkedlist.py. Implement unittests and functionality for a Node class as described below. Node item: Any link: Node | None
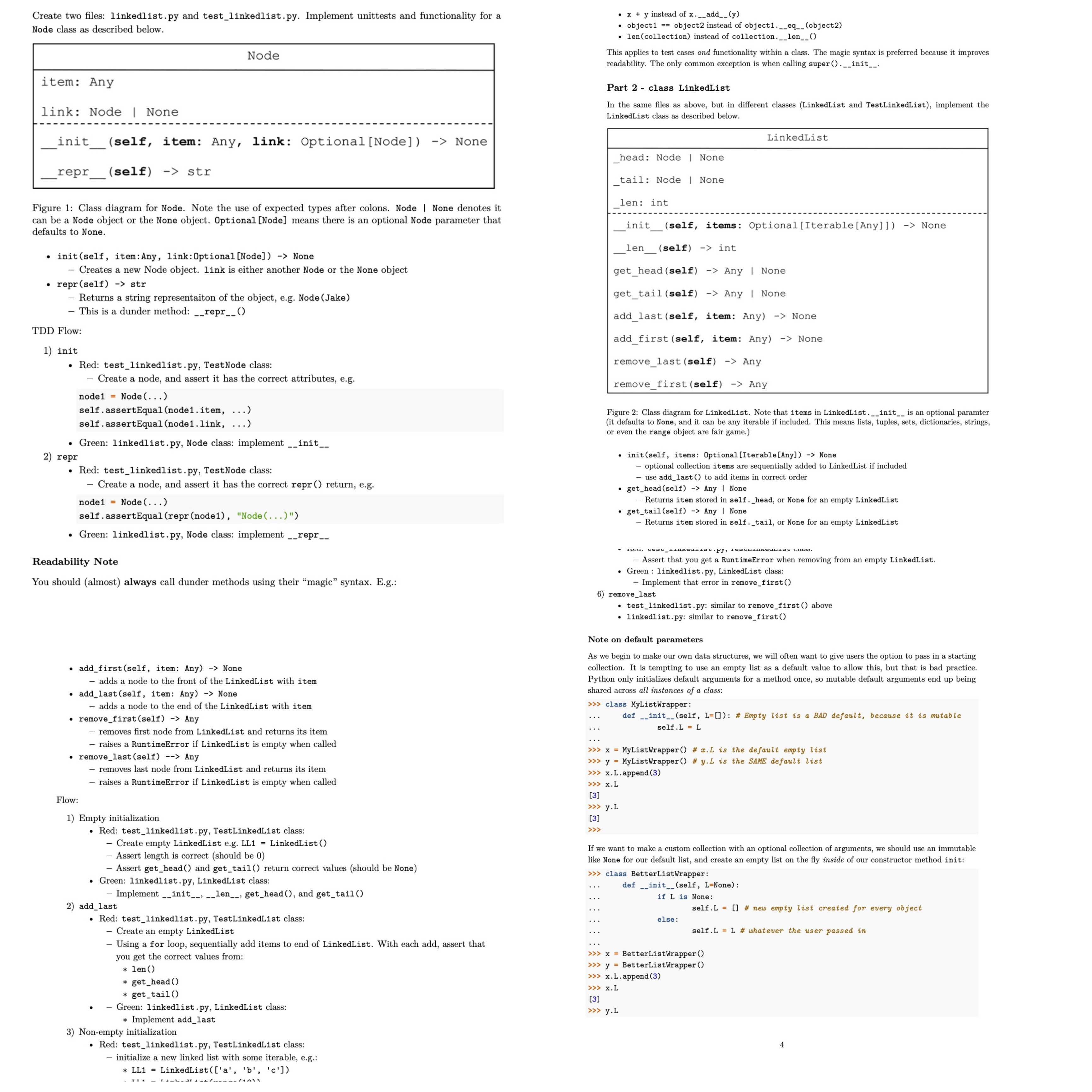
Create two files: linkedlist.py and test_linkedlist.py. Implement unittests and functionality for a Node class as described below. Node item: Any link: Node | None init _ (self, item: Any, link: Optional [Node]) -> None repr (self) -> str Figure 1: Class diagram for Node. Note the use of expected types after colons. Node | None denotes it can be a Node object or the None object. Optional [Node] means there is an optional Node parameter that defaults to None. init(self, item: Any, link: Optional [Node]) -> None Creates a new Node object. link is either another Node or the None object repr(self) -> str TDD Flow: 1) init Returns a string representaiton of the object, e.g. Node (Jake) This is a dunder method: _repr__() Red: test linkedlist.py, TestNode class: Create a node, and assert it has the correct attributes, e.g. node1 = Node(...) self.assertEqual (node1.item, ...) self.assertEqual (node1.link, ...) Green: linkedlist.py, Node class: implement __init__ 2) repr Red: test linkedlist.py, TestNode class: Create a node, and assert it has the correct repr() return, e.g. node1 = Node (...) self.assertEqual (repr (node1), "Node(...)") Green: linkedlist.py, Node class: implement _ repr_ Readability Note You should (almost) always call dunder methods using their "magic" syntax. E.g.: - add first (self, item: Any) -> None adds a node to the front of the LinkedList with item add_last (self, item: Any) -> None adds a node to the end of the LinkedList with item remove_first (self) -> Any - removes first node from LinkedList and returns its item raises a RuntimeError if LinkedList is empty when called remove_last (self) --> Any - removes last node from LinkedList and returns its item raises a RuntimeError if LinkedList is empty when called xy instead of x. _ add __ (y) object1 == object2 instead of object1. _ eq__ (object2) len(collection) instead of collection. _ len __ () This applies to test cases and functionality within a class. The magic syntax is preferred because it improves readability. The only common exception is when calling super().__init__. Part 2 class LinkedList - In the same files as above, but in different classes (LinkedList and TestLinkedList), implement the LinkedList class as described below. head: Node | None _tail: Node | None len: int LinkedList _init__ (self, items: Optional [Iterable [Any]]) -> None len (self) -> int get_head (self) -> Any | None get_tail (self) -> Any | None add_last (self, item: Any) -> None add first (self, item: Any) -> None remove_last (self) -> Any remove first (self) -> Any Figure 2: Class diagram for LinkedList. Note that items in LinkedList. __init__ is an optional paramter (it defaults to None, and it can be any iterable if included. This means lists, tuples, sets, dictionaries, strings, or even the range object are fair game.) init(self, items: Optional [Iterable [Any]) -> None - - optional collection items are sequentially added to LinkedList if included -use add_last () to add items in correct order get_head (self) -> Any | None Returns item stored in self. _ head, or None for an empty LinkedList get_tail (self) -> Any | None Returns item stored in self._tail, or None for an empty LinkedList Assert that you get a RuntimeError when removing from an empty LinkedList. linkedlist.py, LinkedList class: Green - Implement that error in remove_first() 6) remove_last test_linkedlist.py: similar to remove_first () above linkedlist.py: similar to remove_first() Note on default parameters As we begin to make our own data structures, we will often want to give users the option to pass in a starting collection. It is tempting to use an empty list as a default value to allow this, but that is bad practice. Python only initializes default arguments for a method once, so mutable default arguments end up being shared across all instances of a class: >>> class MyListWrapper: ... >>> x = >>> y = def __init__(self, L=[]): # Empty list is a BAD default, because it is mutable self.L = L MyListWrapper() #23 x.L is the default empty list MyListWrapper() #3 y.L is the SAME default list >>> x.L.append(3) >>> x.L Flow: 1) Empty initialization Red: test linkedlist.py, TestLinkedList class: - Create empty LinkedList e.g. LL1 = LinkedList() - Assert length is correct (should be 0) Assert get_head() and get_tail () return correct values (should be None) Green: linkedlist.py, LinkedList class: - Implement __init__, _len__, get_head(), and get_tail () 2) add_last Red: test_linkedlist.py, TestLinkedList class: - Create an empty LinkedList Using a for loop, sequentially add items to end of LinkedList. With each add, assert that you get the correct values from: * len() * get_head() * get_tail () Green: linkedlist.py, LinkedList class: * Implement add_last 3) Non-empty initialization Red: test_linkedlist.py, TestLinkedList class: - initialize a new linked list with some iterable, e.g.: * LL1 = LinkedList(['a', 'b', 'c']) TTA TAT [3] >>> y.L [3] >>> If we want to make a custom collection with an optional collection of arguments, we should use an immutable like None for our default list, and create an empty list on the fly inside of our constructor method init: >>> class BetterListWrapper: def _ init__(self, L=None): if L is None: self.L else: self.L >>> X >>> y = BetterListWrapper() BetterListWrapper () >>> x.L.append(3) >>> x. L [3] >>> y.L [] # new empty list created for every object = L # whatever the user passed in
Step by Step Solution
There are 3 Steps involved in it
Step: 1
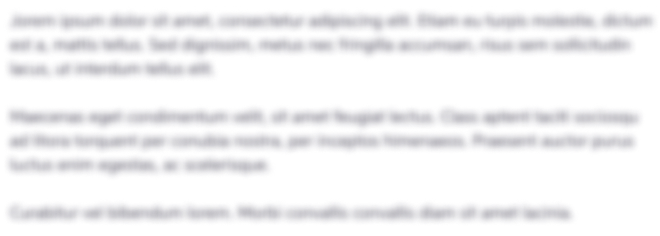
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started