Question
Creating a java program that has the following: Objectives: Using input and output text files Using the Scanner class Using ArrayList Using the String split,
Creating a java program that has the following:
Objectives:
Using input and output text files
Using the Scanner class
Using ArrayList
Using the String split, matches, and other methods
Using some of the Character class methods
Using regular Expressions
Using Exceptions
Using Command-line arguments
Write a program that reads data from a text file and counts the following:
Number of non-empty lines
Number of tokens
Number of alphabetic tokens (i.e. letters only)
Number of integer tokens
Number of double tokens
Number of other than numeric and alphabetic tokens.
Number of non-white space characters.
Number of digits
Number of letters
Number of other than digits and letters characters
Longest token.
Largest numeric value
Input text file and output text file should be entered as command-line arguments (input file name followed by output file name).
Program should send output to an output text file as well as displaying them on the screen.
Each team submits a single copy of project zipped into a single file, additionally, each team member submits her/his project report. Each team should submit the following items:
MyCounts5.java (driver)
ProcessTokens.java. this file should process the input file and compute all counts above.
MySampleRun5
Note: Make sure that your program is well documented, readable, and user friendly.
The output is well labeled and aligned
Sample input
CS 1302 A
6 / 22 / 2017
An Exception can be anything which interrupts the normal flow of the program.
When an exception occurs program processing gets terminated and doesnt continue further
In such cases we get a system generated error message The good thing about exceptions
is that they can be handled
Test1 89.5
Test2 100
Test3 55.75
Final 100.50.759
Quiz 25.
When are we going to have a test?
Are the PPT slides helpful?
You have done a good job!!!
Sample output
1. All tokens
1 CS
2 1302
3 B
4 6
5 /
6 22
7 /
8 2017
9 Fares
10 An
11 Exception
12 can
13 be
14 anything
15 which
16 interrupts
17 the
18 normal
19 flow
20 of
21 the
22 program.
23 When
24 an
25 exception
26 occurs
27 program
28 processing
29 gets
30 terminated
31 and
32 doesnt
33 continue
34 further
35 In
36 such
37 cases
38 we
39 get
40 a
41 system
42 generated
43 error
44 message
45 The
46 good
47 thing
48 about
49 exceptions
50 is
51 that
52 they
53 can
54 be
55 handled
56 Test1
57 89.5
58 Test2
59 100
60 Test3
61 55.75
62 Final
63 100.50.759
64 Quiz
65 25.
66 When
67 are
68 we
69 going
70 to
71 have
72 a
73 test?
74 Are
75 the
76 PPT
77 slides
78 helpful?
79 You
80 have
81 done
82 a
83 good
84 job!!!
2. All Alphabet tokens
1 CS
2 B
3 Fares
4 An
5 Exception
6 can
7 be
8 anything
9 which
10 interrupts
11 the
12 normal
13 flow
14 of
15 the
16 When
17 an
18 exception
19 occurs
20 program
21 processing
22 gets
23 terminated
24 and
25 continue
26 further
27 In
28 such
29 cases
30 we
31 get
32 a
33 system
34 generated
35 error
36 message
37 The
38 good
39 thing
40 about
41 exceptions
42 is
43 that
44 they
45 can
46 be
47 handled
48 Final
49 Quiz
50 When
51 are
52 we
53 going
54 to
55 have
56 a
57 Are
58 the
59 PPT
60 slides
61 You
62 have
63 done
64 a
65 good
3. Double tokens
1 89.5
2 55.75
4. Integer tokens
1 1302
2 6
3 22
4 2017
5 100
5. Other tokens
1 /
2 /
3 program.
4 doesnt
5 Test1
6 Test2
7 Test3
8 100.50.759
9 25.
10 test?
11 helpful?
12 job!!!
6 Counts and, largests, and longest
Count of non-empty lines: 19
Count of all tokens: 84
Count of alphabet tokens: 65
Count of Integer tokens: 5
Count of Double tokens: 2
Count of Other tokens: 12
Count of numberOfCharacters: 376
Count of digits: 34
Count of letters: 328
Count of non-digit & letters: 14
Longest alphabet token: interrupts
Largest numeric value: 2017.00
Regular Expression Syntax
Here is the table listing down helpful regular expression metacharacter syntax available in Java
Subexpression Matches
^ Matches the beginning of the line.
$ Matches the end of the line.
. Matches any single character except newline. Using m option allows it to match the newline as well.
[...] Matches any single character in brackets.
[^...] Matches any single character not in brackets.
\A Beginning of the entire string.
\z End of the entire string.
\Z End of the entire string except allowable final line terminator.
re* Matches 0 or more occurrences of the preceding expression.
re+ Matches 1 or more of the previous thing.
re? Matches 0 or 1 occurrence of the preceding expression.
re{ n} Matches exactly n number of occurrences of the preceding expression.
re{ n,} Matches n or more occurrences of the preceding expression.
re{ n, m} Matches at least n and at most m occurrences of the preceding expression.
a| b Matches either a or b.
(re) Groups regular expressions and remembers the matched text.
(?: re) Groups regular expressions without remembering the matched text.
(?> re) Matches the independent pattern without backtracking.
\w Matches the word characters.
\W Matches the nonword characters.
\s Matches the whitespace. Equivalent to [\t \f].
\S Matches the nonwhitespace.
\d Matches the digits. Equivalent to [0-9].
\D Matches the nondigits.
\A Matches the beginning of the string.
\Z Matches the end of the string. If a newline exists, it matches just before newline.
\z Matches the end of the string.
\G Matches the point where the last match finished.
Back-reference to capture group number "n".
\b Matches the word boundaries when outside the brackets. Matches the backspace (0x08) when inside the brackets.
\B Matches the nonword boundaries.
, \t, etc. Matches newlines, carriage returns, tabs, etc.
\Q Escape (quote) all characters up to \E.
\E Ends quoting begun with \Q.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
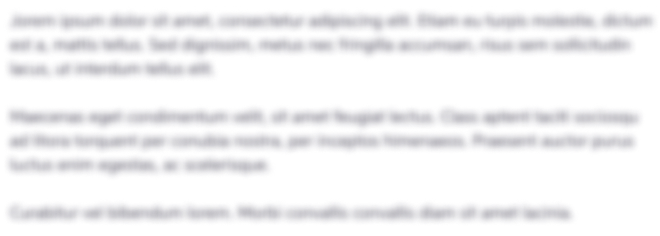
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started