Question
Creating An Additional Class for a Monopoly Game Section 4 of chapter 9 described the BoardSquare class, a class representing a collection of objects for
Creating An Additional Class for a Monopoly Game
Section 4 of chapter 9 described the BoardSquare class, a class representing a collection of objects for squares on a monopoly board. The NetBeans project Monopoly included with the files for this chapter, has that class and a public class named Monopoly with an executable main method.
Your task is to complete the programming exercise at the end of chapter 9 by adding a player class object to the program.
The class discussion for this week is about this project. You may work with other students and communicate about the project through the class discussion, through email, or by meeting in person, The Conference Feature in Canvas, to discuss the design and programming for this project, but each person should do his or her own work, writing his or her own code. You must participate in the class discussion. If you choose to work on this assignment with another person in the class as a team, your lab report should indicate who you worked with.
Remember, this is the first part in building a Monopoly game in Java so we are only testing the player class object moving around the board interacting with the BoardSquare class. Things like picking a card if the player lands on Chance, buying and selling properties,collecting $200 for passing Go, and so on, are not part of this project. In a real development situation, those things would be added in another phase of the project once the BoardSquare and Player objects work properly. Graphics (and sound) would also be added later. Dont get carried away with fancy features at this point and make the assignment harder than it needs to be. You only need to advance the player around the monopoly board one time
package monopolyassignment;
/** * @author Mike Hayden */ import java.util.*;
public class MonopolyAssignment {
/** * @param args the command line arguments * @throws java.lang.Exception */ public static void main(String[] args) throws Exception { BoardSquare[] square = new BoardSquare[40]; // array of 40 monopoly squares int i; // a loop counter // call the method to load the array loadArray(square); // test the code by printing the data for each square System.out.println("Data from the array of Monopoly board squares. Each line has: "); System.out.println("name of the square, type, rent, price, color "); for( i=0; i<40; i++) System.out.println( square[i].toString() ); } // main() //*********************************************************************** // method to load the BoardSquare array from a data file public static void loadArray(BoardSquare[] square) throws Exception { int i; // a loop counter // declare temporary variables to hold BoardSquare properties read from a file String inName; String inType; int inPrice; int inRent; String inColor; // Create a File class object linked to the name of the file to be read java.io.File squareFile = new java.io.File("squares.txt");
// Create a Scanner named infile to read the input stream from the file Scanner infile = new Scanner(squareFile);
/* This loop reads data into the square array. * Each item of data is a separate line in the file. * There are 40 sets of data for the 40 squares. */ for( i=0; i<40; i++) { // read data from the file into temporary variables // read Strings directly; parse integers inName = infile.nextLine(); inType = infile.nextLine(); inPrice = Integer.parseInt( infile.nextLine() ); inRent = Integer.parseInt( infile.nextLine() );; inColor = infile.nextLine(); // intialze each square with the BoardSquare constructor square[i] = new BoardSquare(inName, inType, inPrice, inRent, inColor); } // end for infile.close();
} // endLoadArray //***********************************************************************
} // end class Monopoly
class BoardSquare {
private String name; // the name of the square private String type; // property, railroad, utility, plain, tax, or toJail private int price; // cost to buy the square; zero means not for sale private int rent; // rent paid by a player who lands on the square private String color; // many are null; this is not the Java Color class
// constructors public BoardSquare() { name = ""; type = ""; price = 0; rent = 0; color = ""; } // end Square()
public BoardSquare(String name, String type, int price, int rent, String color) { this.name = name; this.type = type; this.price = price; this.rent = rent; this.color = color; } // end Square((String name, String type, int price, int rent, String color)
// accesors for each property public String getName() { return name; } //end getName()
public String getType() { return type; } //end getType()
public int getPrice() { return price; } //end getPrice()
public int getRent() { return rent; } //end getRent()
public String getColor() { return color; } //end getColor() // a method to return the BoardSquare's data as a String public String toString() { String info; info = (name +", "+type+", "+price + ", "+ rent+ ", "+color); return info; } //end toString() } // end class BoardSquare
PLEASE DO NOT COPY AND PASTE ANSWERS FROM OTHER CHEGG Q&A'S BECAUSE THEY ARE WRONG!!!!! GOD HELP ME IF YOU JUST COPY AND PASTE THE SAME ANSWER AS THE LAST TWO QUESTIONS LIKE THIS, BOTH ARE WRONG SO DONT WASTE MY TIME OR I WILL PERSURE THAT REFUND.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
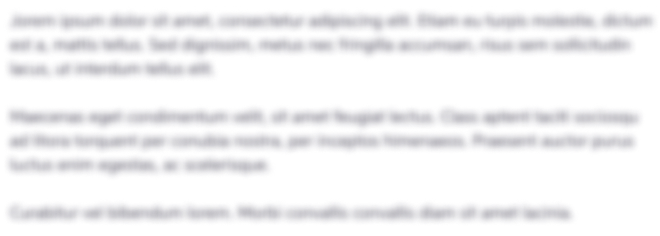
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started