Question
Credit Card Number Check Write a program to check whether a credit card is valid or not. The following algorithm is used to verify actual
Credit Card Number Check
Write a program to check whether a credit card is valid or not.
The following algorithm is used to verify actual credit card numbers:
The last digit of a credit card number is the check digit, which protects against transcription errors such as a single digit or switching two digits. For simplicity, we will describe it for numbers with 8 digits instead of 16:
Starting from the rightmost digit, form the sum of every other digit. For example, if the credit card number is 4358 9795, then you form the sum 5 + 7 + 8 + 3 = 23.
Double each of the digits that were not included in the preceding step. Add all digits of the resulting numbers. For example, with the number given above, doubling the digits, starting with the next-to-last one, yields 18 18 10 8. Adding all digits in these values yields 1 + 8 + 1 + 8 +1 + 0 + 8 = 27.
Add the sums of the two preceding steps. If the last digit of the result is 0, the number is valid. In our case, 23 + 27 = 50, so the number is valid.
As a programmer, your tasks are to design the program in a class diagram, and then implement the design in Java program.
Create a class diagram for the service class ONLY
The service class, CreditCard should include:
A data member card number, which can be an integer or a String
A default constructor
A constructor with a parameter for card number
Mutator and accessor for the data member
At least one method, validateNumber(), which returns a boolean value (optional: you can have more methods to complete this task.)
A method to display result
Implement the design in Java code
Service class:
In the validateNumber() method, to get an individual digit from a whole number, you can use EITHER of the following (Of course, it is good to try both) in any type of loop structures:
If you store a number as an int, use the operators, / and % . For example:
To get the last digit from the number, 43589795, you use 43589795 % 10, the result (reminder) is 5.
To move 5 from the number, you use 43589795/10, the result is 4358979. Remember, this is an integer division!
Then you continue using % to get the last digit in 4358979, and then using / to remove 9, and so on.
In the loop, you repeat the operation above, until only one digit left.
If you treat a number as a String, you can use the method, such as the substring() (P575) in the String class, to get a single digit.
The substring() method returns a String, and cannot be used in a math expressions directly. You can use the Integer.parseInt() method to convert a String to an int. The argument for the method is a String (digit), such as 8.
In the display method, display whether the number is valid or not. The following must be done in the method:If it is not valid, you should print out the value of the check digit that would make the number valid.
To fix the last digit, use the formula:
(cardNumber - finalSum + 10) % 10
Application/client class:
Use either the Scanner or the JOptionPane to get the input from the keyboard.
Make sure the user enters 8 digits. If not, force the user to re-enter a value until a value is correct. You can use the length() method in the String class to check the number of characters, and use methods (P560) in the Character class to check if each character is a digit.
Once the program works, Add a loop structure to allow the user validate multiple numbers and terminate the program using an appropriate sentinel value.
GRASP Wedge2 Hit any key to start Enter 8 digit credit card numbe: 43589794 The credit card number is not valid. The last digit should be 5 ontinue(y>? Enter 8 digit credit card numbe: 43589795 The credit card number is valid. ontinue(y>? Enter 8 digit credit card number: 67034014 The last digit should be? he credit card number is not valid. ontinue (y>? Hit any key to continue
Step by Step Solution
There are 3 Steps involved in it
Step: 1
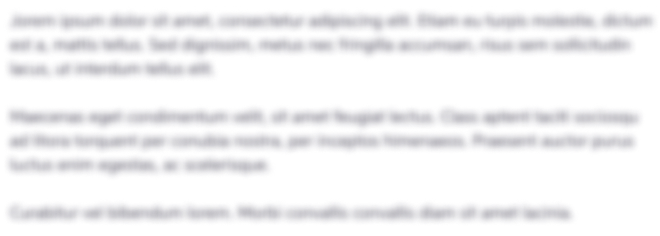
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started