Question
/* CS 125 - Intro to Computer Science * File Name: CS125_Lab5.java * Java Programming * Lab 5 - Due X/XX/XXXX * Instructor: Dr. Dan
/* CS 125 - Intro to Computer Science * File Name: CS125_Lab5.java * Java Programming * Lab 5 - Due X/XX/XXXX * Instructor: Dr. Dan Grissom * * Name 1: * Description: This file contains the source code for Lab 5. */ import java.io.EOFException; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.util.ArrayList; import java.util.Scanner;
public class CS125_Lab5 { public static void main(String[] args) { // Your program should always output your name and the lab/problem // number. DO NOT DELETE OR COMMENT OUT. Replace with relevant info. System.out.println("Zack Owens"); System.out.println("Lab 5"); System.out.println("");
// Declare new employee list ArrayList employees = new ArrayList(); //Instantiate employee list
// Init scanner Scanner scan = new Scanner(System.in);
// Read in choice until user picks valid choice int choice = -1; do { // Prompt user for choice System.out.print("Please select from the following options (type number): " + "\t1. See the list of all employees " + "\t2. Add new employees ");
try { choice = scan.nextInt(); // ...keep asking them for an int. scan.nextLine(); // Clear extra whitespace left by nextInt() } catch(Exception e) { scan.next(); // If they don't enter an int, advance past the bad input }
// Inform user that their choice was not valid if(choice != 1 && choice != 2) System.out.println("Invalid choice: Please try again... ");
} while(choice != 1 && choice != 2);
// Choice 1: List employees if(choice == 1) { // TODO 4: Your code here which calls readEmployeesFromFile() method } else // Choice 2: Add new employees { // TODO 1: Your code here which prompts the user to create and add new employees in a loop..... // Pseudocode: // While user wants to add more employees // Give user prompts to create new employee // firstName, lastName, hourlyRate, years // Create new employee with info just read in // Add new employee to employees ArrayList // Call updateEmployeeFile () method } // TODO 3: Your code here which prints out all employees (Your code here.....) }
//////////////////////////////////////////////////////////////////////// // Reads Employees from a given file and stores them into the employees // list. Returns true upon success, false upon failure. private static boolean readEmployeesFromFile(String fileName, ArrayList employees) { // Initialize input streams FileInputStream fis = null; ObjectInputStream ois = null; boolean readFail = false;
// TODO 5: Your code here which reads Employees from file and adds them to employees ArrayList.....
// Return whether there was success or not if (readFail) return false; else return true; //If we made it here, then the file was read successfully. Return true. }
//////////////////////////////////////////////////////////////////////// // Reads employee list from file and updates the file with any new // employees. Returns true upon success; false upon failure. Do NOT // place any prompts in this method asking the user to input data (that // should all be done in main(). private static boolean updateEmployeeFile(String fileName, ArrayList employees) { // Add employees from file into employees ArrayList readEmployeesFromFile(fileName, employees);
// Initialize output streams FileOutputStream fos = null; ObjectOutputStream oos = null; boolean readFail = false;
// TODO 2: Your code here which writes all the employees in the employees ArrayList out to file..... // Return whether there was success or not if (readFail) return false; else return true; //If we made it here, then the file was read successfully. Return true. } }
import java.text.DecimalFormat;
public class Employee extends UniqueObject { ///////////////////////////////////////////////////// //Fields private String firstName; private String lastName; protected double hourlyRate; protected int years; ///////////////////////////////////////////////////// //Constructors public Employee() { firstName = "John"; lastName = "Doe"; hourlyRate = 9.0; years = 0; } public Employee(String fn, String ln, double hr, int y) { firstName = fn; lastName = ln; hourlyRate = hr; years = y; } ///////////////////////////////////////////////////// //Getters/Setters protected String getFirstName() { return firstName; } protected String getLastName() { return lastName; } protected double getHourlyRate() { return hourlyRate; } protected double getYears() { return years; } protected double getYearlySalary() { return hourlyRate * 40D * 52D; } ///////////////////////////////////////////////////// //Other Methods protected void promote() { years++; hourlyRate *= 1.03; } public String toString() { DecimalFormat money = new DecimalFormat("$0.00"); return firstName + " " + lastName + " (#" + this.getUniqueIdString() + ")" + " has been with the company for " + years + " years" + " and makes " + money.format(hourlyRate) + "/hr."; } }
import java.text.DecimalFormat;
public class UniqueObject { ///////////////////////////////////////////////////// //Fields private static long nextUniqueId = 0; private long uniqueId; ///////////////////////////////////////////////////// //Constructor public UniqueObject() { uniqueId = nextUniqueId++; } ///////////////////////////////////////////////////// // Getters protected long getUniqueId() { return uniqueId; } protected String getUniqueIdString() { DecimalFormat df = new DecimalFormat("0000"); return df.format(uniqueId); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
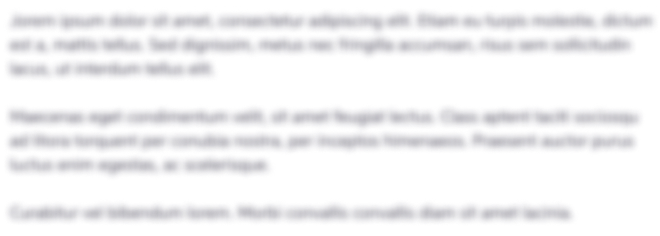
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started