Question
CS210 Introduction to Programming Topic 11: Classes and Objects Requirements for chapter 11 part B zyLab Full Program: Classes & Objects This program will extend
CS210 Introduction to Programming Topic 11: Classes and Objects
Requirements for chapter 11 part B zyLab Full Program: Classes & Objects
This program will extend the warm-up program from Part A.
The program will create two objects, and compute monthly loan payments for each. Then it will compute and compare total interest paid over the life of each loan.
The Loan class from part A will be renamed ModLoan and will contain these additional methods (highlighted in blue below in the URL class diagram):
- a second constructor, to be used to create ModLoan objects using argument values
- three instance methods to compute a monthly payment, to compute total interest paid on a loan, and to display the data fields.
Loan |
-loanNumber: int -loanAmount: double -interestRate: double -termMonths: int |
+Loan() +Loan(loanNumber: int, loanAmount: double, interestRate: double, termMonths: int) +setLoanNumber (loanNumber: int) +setLoanAmount (loanAmount: double) +setInterestRate(interestRate: double) +setTermMonths(termMonths: int) +getLoanNumber(): int +getLoanAmount(): double +getInterestRate(): double +getTermMonths(): int +computeMonthlyPayment(): double +computeTotalInterest(): double +displayLoanTerms() |
Preparing your Project
The program will be written in NetBeans.
Start with a copy of your warm-up project (so you wont lose your warm-up work)
- From file explorer, copy the project folder: Topic11partA
to a new project folder named: Topic11partB
After copying the folder, you will need to open the project and rename the project and file.
- Open the project in the Topic11partB folder in NetBeans.
- Click on the Projects tab
- Right-click the project name (Topic11partA) at the top of the projects window.
- Select Rename, and type Topic11partB for the Project Name, and click the Rename button
- Click on the Files tab, and then click the + next to Topic11partB to open the folder.
- Click the + next to src to open the folder.
- Right-click the file name Loan.java, and select Refactor, then Rename
- Change Loan to ModLoan and then click the Refactor button
- Right-click the file name LoanManager.java, and select Refactor, then Rename
- Change LoanManager to CompareLoans
and then click the Refactor button
Note that all Loan objects in your code will now be ModLoan objects.
Program Requirements
Within the ModLoan class (used to create ModLoan type objects):
- Include JavaDoc top of file comments with a class description and @author/@version tags
- Add an overloaded second constructor that:
- Has parameters for each data field/member of the class (loan number, loan amount, interest rate, and number of months in term, in that order, in that order)
- Uses the parameter values to initialize the data fields.
NOTE: Do not add any additional data fields to the ModLoan class.
- Add the following additional methods to the ModLoan class after the setters and getters (must use these exact method names):
- computeMonthlyPayment()- public method to compute monthly loan payment
- Divide the annual interest rate by 100 to get a value that can be used in computations (e.g. converts 5.5 to 0.055). Then divide that value by 12 to get the Monthly Interest Rate to be used in the formulas below.
- Use the following formula to compute the loans monthly payment.
- computeMonthlyPayment()- public method to compute monthly loan payment
Monthly Payment = (Factor * Monthly Interest Rate * Loan Amount) / (Factor - 1);
- The previous formula requires that you first calculate a factor and then use it in the formula. The factor formula is:
Factor = exp( Number of Months in Loan * log(1 + Monthly Interest Rate))
NOTE: exp and log are predefined math functions, located in the Math class.
-
- This method will have no parameters, and will return the monthly payment.
- computeTotalInterest() - public method to compute the total interest paid over the life of the loan.
- This method will have one parameter, the monthly loan payment,
- Total interest will be computed by determining the total amount paid over the life of the loan, and deducting the initial loan amount from that value.
- After computing the total interest, round it to two decimal places using the formula:
totalInterest = Math.round(totalInterest * 100) / 100.00;
-
- This method will return the rounded total interest for the loan.
- displayLoanTerms() - public method to all display the data field values,
exactly as they were formatted as in Topic11partA
Loan 112233 is for $200000.00 at 6.50% APR, to be paid over 360 month(s)
-
- Note that the getters will no longer be needed to access the data fields for this display.
- This method will have no parameters and will not return anything.
- Add JavaDoc method comments above each method,
with method descriptions, @return tags, and @param tags.
Within the CompareLoans class:
- Include JavaDoc top of file comments with a program descriptions and @author/@version tags
- Add JavaDoc method comments above each method, with method descriptions and any necessary @return tags and @param tags.
- Add another public static method as shown in blue in the URL diagram below:
LoanManager |
+main(args[]: String) +readLoanTerms(keyboard: Scanner, oneLoan: Loan) +createLoanObject(keyboard: Scanner): Loan |
- This createLoanObject() method will:
- Have one parameter:
- a Scanner object (to read data from the keyboard)
- Similar to the readLoanTerms() method, and using the same prompts, this method will read the loan terms (loan number, loan amount, interest rate, and number of months in term, in that order) from the user.
- Declare a ModLoan type variable, and instantiate a ModLoan object using the overloaded second constructor, with the values read from the user as the arguments for the constructor parameters.
- Return the ModLoan object created.
Within the CompareLoans class main method:
- After the code that:
- Creates the first ModLoan object
- Calls the readLoanTerms() method, from Topic 11 part A
- Displays a blank line.
DELETE the code that uses the getters to display the values of each data field of the ModLoan object.
- Add code to call the createLoanObject () method to create a second Loan object.
Note that you will have to capture the object that is returned from the method, and store it into a
ModLoan object variable.
Sample declaration and call:
Loan LoanObjectName = createLoanObject(scannerObjectName);
- Display a blank line.
- For each ModLoan object (i.e. TWICE):
- Call the computeMonthlyPayment() method with the ModLoan object and store the returned value.
- Call the computeTotalInterest() method with the ModLoan object and save the returned value.
- Call the displayLoanTerms() method with the ModLoan object.
- Display the monthly payment and total interest paid that were computed for the
ModLoan object.
-
- Display a blank line.
- Finally, add code to determine which loan requires that less interest be paid, and display the results in one of the following formats,
using the getter methods to access the ModLoan objects loan numbers.
Loan 111111 requires less total interest than loan 222222
or or
Sample run:
Loan 222222 requires less total interest than loan 111111 Both loans require the same amount of total interest
Enter loan number:
112233
Enter loan amount:
200000
Enter annual interest rate (e.g. 5.5):
6.5
Enter loan term (in months):
360
Enter loan number:
445566
Enter loan amount:
200000
Enter annual interest rate (e.g. 5.5):
7
Enter loan term (in months):
240
Loan 112233 is for $200000.00 at 6.50% APR, to be paid over 360 month(s) The monthly payment is $1264.14
The total interest on the loan is $255088.98
Loan 445566 is for $200000.00 at 7.00% APR, to be paid over 240 month(s) The monthly payment is $1550.60
The total interest on the loan is $172143.49
Loan 445566 requires less total interest than loan 112233
Your program code must follow the CS210 Coding Standards, and include file and method JavaDoc comments.
Submission
After you finish coding your solution, drag and drop the CompareLoans.java AND the
ModLoan.java source code files from NetBeans project src folder into the zyLab for testing. There will again be both Unit tests and Output tests for this program
Step by Step Solution
There are 3 Steps involved in it
Step: 1
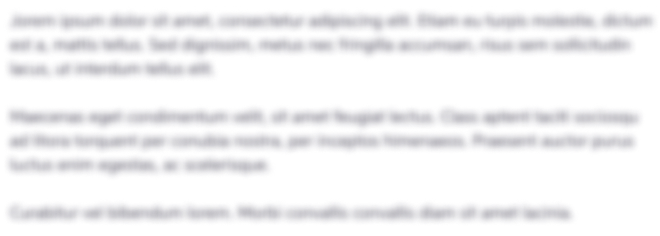
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started