Question
CSc 210 Project 5 Locating peaks in data (with extra credit) due 11:55 pm Monday 4/9/2018 (standard 48 hour grace period for 75% of credit)
CSc 210 Project 5
Locating peaks in data (with extra credit)
due 11:55 pm Monday 4/9/2018
(standard 48 hour grace period for 75% of credit)
(This document is available from the CSc 210 iLearn site.)
You may work by yourself, or in groups of two. Both partners in each group are expected to contribute equally to each part, and will get equal scores.
When working with a sequence of data with a lot of variation, such as the average temperature over a number of days, it is often useful to find the well-defined peaks in the data that stand out from the background variation. These correspond to the days that are hotter than usual.
There are many approaches to locating peaks. Consider an array of data x[]. For our project, we define a peak as an element of the array that is a local maximum, greater than each of its neighbors by a factor of 2 or more. The first and last elements in an array have only one neighbor each, and by definition cannot be peaks. Hence, given this array x[] with 20 terms:
0.2000 0.5000 0.1000 0.1500 0.2000 0.1300 0.3000 0.2500 0.3000 0.3000
0.7000 0.2000 0.4500 0.1500 0.2000 0.8500 0.3000 0.6500 0.2000 0.1000
The peaks are at x[1] = 0.5, x[10] = 0.7, x[12] = 0.45, x[15] = 0.85, x[17] = 0.65.
For this project, you will write a program that scans through an array of data, finds the peaks based on the criterion defined above, and sorts them into non-descending order. Heres what you program should do:
Declare and initialize an array x[] of 20 floats.
float [] x = {0.2f, 0.5f, 0.1f, 0.15f, 0.2f, 0.13f, 0.3f, 0.25f, 0.3f, 0.3f,
0.7f, 0.2f, 0.45f, 0.15f, 0.2f, 0.85f, 0.3f, 0.65f, 0.2f, 0.1f};
Print the elements of x[], 10 on each line.
Then locate the peaks in x[], place them in an array peaks[]
Print the index and data for each peak, in the sorted order
A sample run:
libra% java FindPeaks
Data array:
0.2000 0.5000 0.1000 0.1500 0.2000 0.1300 0.3000 0.2500 0.3000 0.3000
0.7000 0.2000 0.4500 0.1500 0.2000 0.8500 0.3000 0.6500 0.2000 0.1000
5 peaks found:
1 0.5
10 0.7
12 0.45
15 0.85
17 0.65
libra%
After printing the elements of x[] , your program will first step through the elements of x[] , find each peak, and add each peak to the array peaks[] . This is the very common operation of building a list that contains elements with interesting properties; well discuss how to do this in class.
Extra credit (20 out of 100 points):
It is also useful to have the peaks sorted in order of their magnitudes. Hence, in non-decreasing order, the peaks would be listed as x[12], x[1], x[17], x[10], x[15].
Once weve built the array containing the peaks, there are several ways to sort the peaks. You can obviously use java.util.Arrays.sort(); see Chapter 6 slides. This is easy, but you wont be able to report the index of each peak.
Another way is to just copy the selection sort code we saw in class. You do have to make some small changes so you remember what indices the peaks came from. Displaying the indices as well as the data in the peaks will get you extra credit of 20 points (out of 100) for this project.
A sample run:
Data array:
0.2000 0.5000 0.1000 0.1500 0.2000 0.1300 0.3000 0.2500 0.3000 0.3000
0.7000 0.2000 0.4500 0.1500 0.2000 0.8500 0.3000 0.6500 0.2000 0.1000
5 peaks found:
1 0.5
10 0.7
12 0.45
15 0.85
17 0.65
Sorted peaks:
12 0.45
1 0.5
17 0.65
10 0.7
15 0.85
libra%
Note: do not work on the extra credit part until you have the basic project finished! If you cannot print out the peaks in the original order, we will not even consider your extra credit work.
Programming style and documentation: You must follow the programming style and documentation guidelines specified in previous projects.
Submission: Submit your code (the .java file only) with the name Assignment5.java using the iLearn submission link.
Grading deductions:
-20 points for not following guidelines for variables (eg using a double when an int is asked for)
-10 points for not following guidelines for output
-50 points if code does not compile
-25 points if results do not match with the base case.
15 0.85 17 0.65 libra You may work by yourself, or in groups of two Both partners in ach group are experted to contibute equally to each part, and will get equal scoes. After printing the elements of x, your program will first step through the elements of xl. find each peak, and add each peak to the array peaks.This is the very common operation of building a list that contains elements with "interesting" properties; well discuss how to do this in class. When working with a sequence of data with a lot of variation, such as the average teauperature over a number of days, i is often useful to firad the well-defined peaks in the data that stand out from the background variation These corespond to the days that are hotter than usual Extra credit (20 out of 100 points): There are many approaches to locating peaks. Consider a aay of data x. For ou project, we define a peak as an element of the array that is a local maximum, greater than each of its neighbors by a facto of 2 oa more. The fust arnd last elements in an aray have only one ncighbor cach, and by definition cannot be peaks. Hence, given this array xl with 20 terms It is also useful to have the peaks sorted in order of their magnitudes. Hence, in non- decreasing order, the peaks would be listed asx12, x1 x17], x[10), x[15]. Once we've built the array containing the peaks, there are several ways to sort the peaks You can obviously use java.utilArrays.sort0; see Chapter 6 slides. This is easy, but you won't be able to report the index of each peak 0.2000 0.5000 0.1000 0.1500 0.2000 0.1300 0.3000 0.2500 0.3000 0.3000 0.7000 0.200004500 01500 0.20000.8500 0 3000 0,6500 02000 0.1000 The peaks are at xl] 0.5, x[10]0.7, x[12] 0.45, x[15] 0.85, x10.65 For tiis project, you will write a program that scans through an array of data, finds the peaks based on the criterion defined above, and sorts them into non-descending order Here's what vou proram shld do: Another way is to just copy the selection sort code we saw in class. You do have to make some small changes so you remember what indices the peaks came from. Displaying the indices as well as the data in the peaks will get you extra credit of 20 points (out of 100) for this project. Declare and initialize an array x[] of 20 floars. floatJx-0.2f, 0.5f, 0.1f, 0.15f, 0.2f, 0.13f, 0.3f, 0.25f, 0.3f, 0.3f, A sample run: 0.7f, 0.2f, 045, 0.151 02f, 0.85f, 0.3f, 0.65f, 02f. 0.1fj Print the clements ofx[l. 10 oa cach line. Then locate the peaks inxl. place them in an array peaks I Print the index and data for each peak. in the sarted order Data array: 0.2000 0.5000 0.1000 0.1500 0.2000 0.1300 0.3000 0.2500 0.3000 0.3000 0.7000 0.2000 0.4500 0.1500 0.2000 0.8500 0.3000 0.6500 0.2000 0.1000 5 peaks found: 1 0.5 10 0.7 12 0.45 15 0.85 17 0.65 A s arpe Data array: 0.2000 ?.5000 0.1000 0"1500 o. 2000 0.1300 0.3000 c.2500 0-3000 o.3c00 ?.7000 ?.2000 0,4500 ?.1500 ?. 2000 0.850? ?. 3000 ?,6500 0.2000 0.1000 pe found : Sorted peaks: 12 0.4S 1 0.5 17 0.65 10 0.7 15 0.85 libra % 15 0.85 17 0.65 libra You may work by yourself, or in groups of two Both partners in ach group are experted to contibute equally to each part, and will get equal scoes. After printing the elements of x, your program will first step through the elements of xl. find each peak, and add each peak to the array peaks.This is the very common operation of building a list that contains elements with "interesting" properties; well discuss how to do this in class. When working with a sequence of data with a lot of variation, such as the average teauperature over a number of days, i is often useful to firad the well-defined peaks in the data that stand out from the background variation These corespond to the days that are hotter than usual Extra credit (20 out of 100 points): There are many approaches to locating peaks. Consider a aay of data x. For ou project, we define a peak as an element of the array that is a local maximum, greater than each of its neighbors by a facto of 2 oa more. The fust arnd last elements in an aray have only one ncighbor cach, and by definition cannot be peaks. Hence, given this array xl with 20 terms It is also useful to have the peaks sorted in order of their magnitudes. Hence, in non- decreasing order, the peaks would be listed asx12, x1 x17], x[10), x[15]. Once we've built the array containing the peaks, there are several ways to sort the peaks You can obviously use java.utilArrays.sort0; see Chapter 6 slides. This is easy, but you won't be able to report the index of each peak 0.2000 0.5000 0.1000 0.1500 0.2000 0.1300 0.3000 0.2500 0.3000 0.3000 0.7000 0.200004500 01500 0.20000.8500 0 3000 0,6500 02000 0.1000 The peaks are at xl] 0.5, x[10]0.7, x[12] 0.45, x[15] 0.85, x10.65 For tiis project, you will write a program that scans through an array of data, finds the peaks based on the criterion defined above, and sorts them into non-descending order Here's what vou proram shld do: Another way is to just copy the selection sort code we saw in class. You do have to make some small changes so you remember what indices the peaks came from. Displaying the indices as well as the data in the peaks will get you extra credit of 20 points (out of 100) for this project. Declare and initialize an array x[] of 20 floars. floatJx-0.2f, 0.5f, 0.1f, 0.15f, 0.2f, 0.13f, 0.3f, 0.25f, 0.3f, 0.3f, A sample run: 0.7f, 0.2f, 045, 0.151 02f, 0.85f, 0.3f, 0.65f, 02f. 0.1fj Print the clements ofx[l. 10 oa cach line. Then locate the peaks inxl. place them in an array peaks I Print the index and data for each peak. in the sarted order Data array: 0.2000 0.5000 0.1000 0.1500 0.2000 0.1300 0.3000 0.2500 0.3000 0.3000 0.7000 0.2000 0.4500 0.1500 0.2000 0.8500 0.3000 0.6500 0.2000 0.1000 5 peaks found: 1 0.5 10 0.7 12 0.45 15 0.85 17 0.65 A s arpe Data array: 0.2000 ?.5000 0.1000 0"1500 o. 2000 0.1300 0.3000 c.2500 0-3000 o.3c00 ?.7000 ?.2000 0,4500 ?.1500 ?. 2000 0.850? ?. 3000 ?,6500 0.2000 0.1000 pe found : Sorted peaks: 12 0.4S 1 0.5 17 0.65 10 0.7 15 0.85 libra %
Step by Step Solution
There are 3 Steps involved in it
Step: 1
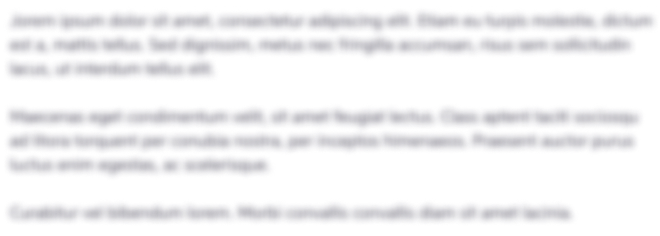
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started