Question
CSEBank has decided to offer a new collection of credit cards to its customers: a basic/traditional credit card, a rewards card, and a prepaid/stored-balance card.
CSEBank has decided to offer a new collection of credit cards to its customers: a basic/traditional credit card, a rewards card, and a prepaid/stored-balance card. For this assignment, you will develop a program that processes a data file containing a list of customer transactions and prints a monthly statement for each card. Follow the instructions below carefully; they are extensive, but they provide a highly-detailed description of how to complete each step of the development process. Part 1: Laying The Foundations 1. To begin with, we need to develop classes to represent the different card types. These classes share various properties, so inheritance is extremely useful here. For example, a RewardsCard is a specialized type of CreditCard. A PrepaidCard is similar to a CreditCard, but not identical, so it should be a sibling that shares a common BankCard parent class. We have provided a fully-implemented Transaction helper class that represents a single card transaction (e.g., a credit or debit). While you should inspect the source code for this class yourself to see exactly how it works, a quick summary of its public interface methods is given below: A single constructor: Transaction(String type, String merchant, double amount) Four (no-argument) accessor methods: type(), amount(), merchant(), and notes() Two mutator methods: setNotes() and addNotes(), each of which takes one String argument A toString() method We have also given you source code for three test driver programs to make sure that your BankCard subclasses work correctly before you proceed to Part 2 (the main program). If you plan to use these drivers to double-check your code (not strictly mandatory, but HIGHLY recommended!), be sure that each of your classes uses the exact method headers described below; otherwise, the test drivers won't work (at least, not without some significant modification). Every card issued by CSEBank has a 16-digit number whose first two digits indicate its type: a. Credit cards have numbers that begin with 84 or 85. b. Rewards cards have numbers that begin with 86 or 87. c. Prepaid cards have numbers that begin with 88 or 89.2. Start by defining an abstract BankCard class with the following elements: a. Instance variables: i. a private String variable that stores the cardholder's name ii. a protected long variable that holds the card number (a 16-digit integer) iii. a protected double variable that stores the card's current balance iv. a protected ArrayList that holds Transaction objects b. Constructors and instance methods (DO NOT modify the headers/signatures specified below): i. public BankCard(String cardholderName, long cardNumber). This constructor sets the initial card balance to 0. ii. public double balance(). This method returns the current balance of the card. iii. public long number(). This method returns the card number. iv. public String cardHolder(). This method returns the cardholder's name. v. public String toString(). This method returns a String containing (at minimum) the card number and the current balance, with appropriate labels, as in Card # 1234567890123456 Balance: $1234.56 vi. public abstract boolean addTransaction(Transaction t). When implemented, this method attempts to performs the specified Transaction on a card. Its return value indicates success or failure. vii. public abstract void printStatement(). When implemented, this method prints the current card's basic information (cardholder name, card number, balance, etc.), along with a list of transactions that have been completed successfully. 3. The CreditCard class extends BankCard. It contains the following elements: a. Instance variables: i. a private integer variable that stores the card's expiration date: a 3- or 4-digit integer in myy or mmyy form. Note that single-digit months CANNOT be preceded with a leading 0; if they are, Java will assume that the integer is written in octal (base 8) instead of decimal (base 10) as intended. ii. a protected double variable that stores the card's credit liimit (in dollars) b. Constructors and instance methods: i. public CreditCard(String cardHolder, long cardNumber, int expiration, double limit). For now, you may assume that the card number is always valid.ii. public CreditCard(String cardHolder, long cardNumber, int expiration). This constructor sets the credit limit to $500.00. For now, you may assume that the card number is always valid. iii. public double limit(). This method returns the credit limit for this CreditCard. iv. public double availableCredit(). This method returns the difference between the credit limit and the current balance (i.e., limit - balance). v. public int expiration(). This method returns the expiration date for this CreditCard. vi. public String toString(). This method overrides BankCard's version, returning a String with the card number, its expiration date, and its current balance. vii. public boolean addTransaction(Transaction t). This method implements the abstract BankCard method. It returns a boolean value according to the rules below: 1. If the Transaction type is "debit" and the transaction amount is less than or equal to this card's available credit, the transaction is accepted; its amount is added to the card balance, the Transaction is added to the card's ArrayList of transactions, and the method returns true. 2. If the Transaction type is "debit" and its amount is greater than this card's available credit, the transaction is declined; the ArrayList does not change, and the method returns false. 3. If the Transaction type is "credit", the transaction amount (which will be a negative number) is added to the card balance (lowering it), the Transaction is added to the card's ArrayList of transactions, and the method returns true. 4. If the Transaction has any other type (like "redemption"), the ArrayList does not change, and the method returns false. viii. public void printStatement(). This method implements the abstract method from BankCard. It prints out the card's basic information (cardholder name, card number, expiration date, and ending balance), along with a neatly-formatted list of card transactions. 4. The RewardsCard class extends CreditCard. It contains the following elements:a. Instance variables: i. a protected integer variable that stores the current number of available reward points b. Constructors and instance methods: i. public RewardsCard(String holder, long number, int expiration, double limit). This constructor initializes the reward points to 0. For now, you may assume that the card number is always valid. ii. public RewardsCard(String holder, long number, int expiration). This constructor sets the credit limit to $500.00 and initializes the reward points to 0. For now, you may assume that the card number is always valid. iii. public int rewardPoints(). This method returns the current number of reward points.v. public boolean redeemPoints(int points). This method operates as follows: 1. If the parameter is less than or equal to the current number of reward points: a. Reduce the card balance by an amount equal to the number of points redeemed divided by 100.00 (for example, 1255 reward points can be used to lower the card balance by $12.55)1 b. Subtract the number of points redeemed from the current number of reward points c. Create a new Transaction and add it to this card's list of transactions. The new Transaction has the type "redemption". Its amount is equal to the value of the balance reduction you just calculated (the Transaction class will automatically make this value negative to reflect that it is a form of credit). Use "CSEBank" as the merchant name. Leave the notes field empty. d. Return true to indicate success. 2. Otherwise, do not change the current balance, number of accumulated reward points, or the list of transactions. Return false to indicate failure. v. public String toString(). This method returns a String containing the same data as the CreditCard version, plus the current number of available reward points. vi. public void addTransaction(Transaction t). This method overrides the version inherited from CreditCard. Its behavior matches the inherited version, except successful "debit" transactions also generate reward points equal to 100 times the transaction amount (cast to an integer). For example, a successful debit of $12.34 earns 1234 reward points. vii. public void printStatement(). Like its superclass equivalent, this method prints out a neatly-formatted list of card transactions, along with the rest of the card's information (don't forget to include the reward points as well!). 5. The PrepaidCard class extends BankCard. It defines the instance methods below (it does not add any new instance variables). Note that several of these operations are somewhat different from their counterparts in CreditCard and RewardsCard! a. public PrepaidCard(String cardHolder, long cardNumber, double balance). For now, you may assume that the card number is always valid. b. public PrepaidCard(String cardHolder,long cardNumber). This constructor sets the starting balance to 0. For now, you may assume that the card number is always valid. c. public boolean addTransaction(Transaction t). This method works as follows: i. If the Transaction type is "debit" and the transaction amount is less than or equal to this card's balance, the transaction is accepted; subtract its amount from the card balance, add the Transaction to the ArrayList of transactions, and return true.ii. If the Transaction type is "debit" and its amount is greater than the card balance, the transaction has been declined. Do not change the card balance or the ArrayList; just return false. iii. If the Transaction type is "credit", subtract its amount from the card balance (credits have negative amounts, so this effectively adds money back to the PrepaidCard), add the Transaction to the ArrayList of transactions, and return true. iv. If the Transaction has any other type (like "redemption"), do not change the card balance or the ArrayList; just return false. d. public boolean addFunds(double amount). This method works as follows: i. If the parameter is positive, it is added to the card's balance, and a new Transaction is added to the PrepaidCard's ArrayList with a type of "top-up", a merchant name of "User payment", an amount equal to the argument, and no notes. Return true to indicate success. ii. Otherwise, do not modify the balance or the transaction list. Return false to indicate failure. e. public String toString(). This method returns a String that contains, at minimum, the card number and its current balance. f. public void printStatement(). This method implements the abstract method from BankCard. It prints out a neatly-formatted list of card transactions, along with the rest of the card's information (cardholder name, card number, and ending balance). 6. Define a new CardList class. This class contains the following elements:a. Instance variables: i. a private ArrayList that can hold BankCard objects (including any of its subclasses). b. Constructors and instance methods: i. public CardList(). This constructor assigns a new (empty) ArrayList object to the instance variable above. ii. public void add(BankCard b). This method adds its parameter to the internal ArrayList (you may add at any fixed position you want; we suggest the end for simplicity). iii. public void add(int index, BankCard b). Index values are 0-based, just like traditional arrays and ArrayLists. If the specified index is between 0 and the internal ArrayList's current size (inclusive), this method inserts the BankCard at that index. Otherwise, the BankCard is added at the end of the ArrayList. This method is OPTIONAL; you are NOT required to implement it for this program (although it may be nice to have)! iv. public int size(). This method returns the current size of the internal ArrayList (meaning the number of elements it contains). v. public BankCard get(int index). Index values are 0-based, just like traditional arrays and ArrayLists. If the specified index is valid, this method returns a reference to the BankCard at that index within the internal ArrayList (it does not alter the ArrayList's contents in any way). Otherwise (meaning that the index value is negative or greater than or equal to the CardList's current size), this method returns null. vi. public long indexOf(long cardNumber). This method uses linear search (or a different search technique, if you wish, as long as it works correctly) to return the (0-based) index of the first (and ideally only) BankCard in the ArrayList whose card number matches the parameter value. If the ArrayList does not contain a BankCard object with a matching card number, this method returns -1. Part 2: Implementing The Main Program Now that our program foundations are (finally!) complete, it's time to develop the main program. Our program will perform the following tasks (full details are provided below, including the specific format for each type of data file): c. Prompt the user for and read in the name of a (plain text) data file containing information describing a number of BankCards. Each line of the data file describes one BankCard. d. Read and parse the contents of this file to create and populate (fill in) a new CardList. e. Prompt the user for and read in the name of a (plain text) data file containing a list of transactions. Each line of the file describes a separate transaction. f. Process this second file, one line at a time. For each transaction, locate the matching card in your CardList and apply the transaction to it. g. After the transaction file has been completely processed, display a final monthly statement for each card in your CardList. We have provided a program skeleton (TransactionProcessor.java) for you to complete this part of the project. It includes a method named getCardType(), which returns a String identifying the type of card that a given card number represents. Complete the TransactionProcessor class as follows:1. Add a method with the header: public static BankCard convertToCard(String data) This method uses a Scanner to break up the input String into a long (the card number), a String (the cardholder name), and possibly a double (the card limit) followed by an int (the expiration date). It returns a subclass of BankCard built using that data: a. Start by creating a Scanner to read from the parameter. Recall that you can initialize a Scanner with a String as its argument instead of the more commonly-used System.in. If you do this, the Scanner will only collect input from that string, rather than from standard input (the keyboard). Use the Scanner method nextLong() to retrieve the card number. b. Use the provided getCardType() method to get a String corresponding to the type of card this data belongs to. If the card type is null, skip the next step and move to the next line of the file. c. Using an if-else chain, determine which of the three BankCard subclasses you are dealing with. Then read the remaining fields from the parameter (see the end of this document for details on the data file format) and create and return a new card object of the appropriate type. 2. Add a method with the header: public static CardList loadCardData(String fName) This method takes the name of a card data file as its argument. It opens and processes that file, one line at a time, before returning a CardList of BankCards based on the data file contents (you may add the cards to the CardList in any order you want, not necessarily the order they are listed in the card data file). Use the convertToCard() method you defined in the previous step to process each individual line of the data file. If you encounter into an IOException, your method should return null to indicate failure. 3. Add a method with the header: public static void processTransactions(String filename, CardList c) This method opens the card transaction file named filename for reading. For each line in the file: a. Use the String method split() to divide the line into an array of smaller substrings (use a single space as the separator/delimiter). b. Use the Long.parseLong() utility method to convert the first substring (the card number) from a String into a long value. c. Find the index of the card with that card number in your CardList. d. The second substring indicates what type of transaction we are working with (you may assume that all transactions are performed on the correct type of card): i. If the transaction type is "redeem": 1. Convert the third substring to an integer value (the number of points to redeem) with Integer.parseInt(). 2. Retrieve the card at the specified index and cast it back to a RewardsCard. 3. Call the card's redeemPoints() method with the number of points. ii. If the transaction type is "top-up": 1. Convert the third substring to a double value (the amount of money to add) with Double.parseDouble(). 2. Retrieve the card at the specified index and cast it to a PrepaidCard. 3. Call the card's addFunds() method with the top-up value. iii. Otherwise: 1. Create a new Transaction from the second, fourth, and third substrings (the third substring should be converted to a double value using Double.parseDouble()). Leave this Transaction's notes field empty. 2. Retrieve the card at the specified index (as a BankCard object). 3. Call that card's addTransaction() method with the Transaction object you just created. e. If you encounter an IOException, stop processing the file and return immediately from the method (do not try to reverse any transactions that you have already processed successfully). 4. Add a main() method to your TransactionProcessor class. main() should use your helper methods from the previous steps to perform the following sequence of tasks: a. Read in the name of the card data file from the user. b. Use loadCardData() to create a CardList from the contents of the card data file. c. If loadCardData() successfully creates a list of cards: i. Read in the name of a transaction data file from the user. ii. Use processTransactions() to read the contents of the transaction file and apply them to the list of cards. iii. Once you have finished processing the transaction file, print out a summary statement for each card in the CardList (using its printStatement() method).Data File Formats The card data file is a plain text file with no blank lines. Each line of the file has two required values (the card number and cardholder name, in that order), possibly followed by one or two optional values. There is a single space between each field: card_number cardholder (for a PrepaidCard with the default starting balance) or card_number cardholder expiration (for a CreditCard or RewardsCard with the default credit limit) or card_number cardholder balance (for a PrepaidCard with a specific starting balance) or card_number cardholder expiration limit (for a CreditCard or RewardsCard with a specified credit limit) Every field has a specific type: card_number is a long. cardholder is a String where all spaces have been replaced by underscore ('_') characters (so, for example, "John Smith" will be represented as "John_Smith"). expiration is an int. balance and limit are both of type double. For example: 8434567812345678 John_Smith 318 12345.67 represents a CreditCard whose expiration date is March 2018 and whose credit limit is $12345.67. Likewise, 8902345682910224 Mary_Doe represents a PrepaidCard issued to "Mary Doe" with an initial balance of $0The transaction data file is a plain text file with no blank lines. Each line of the file begins with two required fields (the card number and transaction type, in that order), followed by one or two additional fields (depending on the transaction type). Each field is separated by a single space. The card number is a long value. There are four transaction types (each of which is a String): redeem, top-up, debit, and credit redeem transactions have a third field (an int) representing the number of points being redeemed. top-up transactions include a third field (a double) representing the amount of money to add. debit and credit transactions include two additional fields representing the transaction amount (a double) and the merchant name (a String where all spaces have been replaced by underscore ('_') characters), respectively. Sample transaction records: 8634567812345678 redeem 2048 8853946610327745 top-up 500.00 8734621893246154 debit 397.45 Amazon 8419567820318835 credit -98.72 Gas_N_Go
public class Transaction { private String type; private double amount; private String merchant; private String notes; public Transaction(String type, String from, double amt) { this.type = type.toLowerCase(); // accept "Debit" as well as "debit", for example // Note: Since the majority of BankCard subtypes accumulate debit // amounts each billing cycle, debits are always positive values, // while credits are listed as negative values. We enforce that here. amount = roundToTwoPlaces(amt); if (type.equals("debit") && amount < 0) { amount = -1.0 * amount; // make the debit a positive value } if (type.equals("credit") && amount > 0) { amount = -1.0 * amount; // make the credit a negative value } if (type.equals("redemption") && amount > 0) { amount = -1.0 * amount; // redemptions are credits, and therefore negative } merchant = from; notes = ""; } public String type() { return type; } public double amount() { return amount; } public String merchant() { return merchant; } public String notes() { return notes; } // Append date to the existing notes field public void addNotes(String newData) { if (notes.length() > 0) { notes += " "; // start a new line for the new information } notes += newData; } // Replace the existing notes for this Transaction public void setNotes (String newData) { notes = newData; } public String toString() { String result = type + "\t" + merchant + "\t"; String transAmount = "" + amount; // If the transaction amount ends with a single digit after the // decimal point, add a trailing 0 to force it to two decimal places if (transAmount.charAt(transAmount.length()-2) == '.') { // Add on a second trailing 0 transAmount += "0"; } result = result + transAmount; if (notes.length() > 0) // add notes on a new line, if present { result = result + " \t" + notes; } return result; } // Make sure that the Transaction's value has exactly two decimal places private double roundToTwoPlaces (double value) { value = value * 100; double truncatedValue = Math.floor(value); return truncatedValue / 100.0; } }
// Basic test harness for the CreditCard class import java.util.*; public class CreditCardTest { private static Random r; public static void main (String [] args) { r = new Random(); // REMINDER: Add a lowercase 'l' after a number to make it a 'long' rather than an 'int' CreditCard c1 = new CreditCard("John Smith", 8525028613937723l, 1015, 10000); CreditCard c2 = new CreditCard("Mary Doe", 8488968564565858l, 216); CreditCard c3 = new CreditCard("Bob Williams", 8444730419040650l, 315, 25000); // technically invalid # (will only be caught by one of the extra-credit options) // Add some sample transactions to each card for (int i = 0; i < 5; i++) { Transaction t = createTransaction(); System.out.println("Added to c1: " + t); c1.addTransaction(t); t = createTransaction(); System.out.println("Added to c2: " + t); c2.addTransaction(t); t = createTransaction(); System.out.println("Added to c3: " + t); c3.addTransaction(t); } // Check the results c1.printStatement(); System.out.println(" "); c2.printStatement(); System.out.println(" "); c3.printStatement(); } private static Transaction createTransaction() { // Create and return a randomly-generated transaction String [] merchants = {"Amazon", "NewEgg", "Office Supplies Plus", "Pet Depot", "Cherry Computer", "Groceries R Us", "Gas N Go"}; String from = merchants[r.nextInt(merchants.length)]; int amountTemp = r.nextInt(50000) - 10000; double amount = amountTemp / 100.00; String type = ""; if (amount < 0) { type = "credit"; } else { type = "debit"; } return new Transaction(type, from, amount); } }
// Basic test harness for the CreditCard class import java.util.*; public class CreditCardTest { private static Random r; public static void main (String [] args) { r = new Random(); // REMINDER: Add a lowercase 'l' after a number to make it a 'long' rather than an 'int' CreditCard c1 = new CreditCard("John Smith", 8525028613937723l, 1015, 10000); CreditCard c2 = new CreditCard("Mary Doe", 8488968564565858l, 216); CreditCard c3 = new CreditCard("Bob Williams", 8444730419040650l, 315, 25000); // technically invalid # (will only be caught by one of the extra-credit options) // Add some sample transactions to each card for (int i = 0; i < 5; i++) { Transaction t = createTransaction(); System.out.println("Added to c1: " + t); c1.addTransaction(t); t = createTransaction(); System.out.println("Added to c2: " + t); c2.addTransaction(t); t = createTransaction(); System.out.println("Added to c3: " + t); c3.addTransaction(t); } // Check the results c1.printStatement(); System.out.println(" "); c2.printStatement(); System.out.println(" "); c3.printStatement(); } private static Transaction createTransaction() { // Create and return a randomly-generated transaction String [] merchants = {"Amazon", "NewEgg", "Office Supplies Plus", "Pet Depot", "Cherry Computer", "Groceries R Us", "Gas N Go"}; String from = merchants[r.nextInt(merchants.length)]; int amountTemp = r.nextInt(50000) - 10000; double amount = amountTemp / 100.00; String type = ""; if (amount < 0) { type = "credit"; } else { type = "debit"; } return new Transaction(type, from, amount); } }
// Basic test harness for the RewardsCard class import java.util.*; public class RewardsCardTest { private static Random r; public static void main (String [] args) { r = new Random(); // REMINDER: Add a lowercase 'l' after a number to make it a 'long' rather than an 'int' RewardsCard c1 = new RewardsCard("John Smith", 8655060645070813l, 1015, 10000); RewardsCard c2 = new RewardsCard("Mary Doe", 8762574107106867l, 216); RewardsCard c3 = new RewardsCard("Bob Williams", 8752474634030982l, 315, 25000); // technically invalid # (will only be caught by a particular extra-credit option) // Add some sample transactions to each card for (int i = 0; i < 5; i++) { if (i == 3) { // Redeem rewards points instead of adding a debit/credit transaction int pts = r.nextInt(7500) + 500; System.out.println("c1 redeemed " + pts + " points"); c1.redeemPoints(pts); pts = r.nextInt(7500) + 500; System.out.println("c2 redeemed " + pts + " points"); c2.redeemPoints(pts); pts = r.nextInt(7500) + 500; System.out.println("c3 redeemed " + pts + " points"); c3.redeemPoints(pts); } else { Transaction t = createTransaction(); System.out.println("Added to c1: " + t); c1.addTransaction(t); t = createTransaction(); System.out.println("Added to c2: " + t); c2.addTransaction(t); t = createTransaction(); System.out.println("Added to c3: " + t); c3.addTransaction(t); } } // Check the results c1.printStatement(); System.out.println(" "); c2.printStatement(); System.out.println(" "); c3.printStatement(); } private static Transaction createTransaction() { // Create and return a randomly-generated transaction String [] merchants = {"Amazon", "NewEgg", "Office Supplies Plus", "Pet Depot", "Cherry Computer", "Groceries R Us", "Gas N Go"}; String from = merchants[r.nextInt(merchants.length)]; int amountTemp = r.nextInt(50000) - 10000; double amount = amountTemp / 100.00; String type = ""; if (amount < 0) { type = "credit"; } else { type = "debit"; } return new Transaction(type, from, amount); } }
// Basic test harness for the PrepaidCard class import java.util.*; public class PrepaidCardTest { private static Random r; public static void main (String [] args) { r = new Random(); // REMINDER: Add a lowercase 'l' after a number to make it a 'long' rather than an 'int' PrepaidCard c1 = new PrepaidCard("John Smith", 8844408184802583l, 1000); PrepaidCard c2 = new PrepaidCard("Mary Doe", 8955060645070813l); PrepaidCard c3 = new PrepaidCard("Bob Williams", 8948293193669982l, 2500); // technically invalid # (will only be caught by one of the extra credit options) c2.addFunds(1200.38); // Add some sample transactions to each card for (int i = 0; i < 5; i++) { Transaction t = createTransaction(); System.out.println("Added to c1: " + t); c1.addTransaction(t); t = createTransaction(); System.out.println("Added to c2: " + t); c2.addTransaction(t); t = createTransaction(); System.out.println("Added to c3: " + t); c3.addTransaction(t); } // Check the results c1.printStatement(); System.out.println(" "); c2.printStatement(); System.out.println(" "); c3.printStatement(); } private static Transaction createTransaction() { // Create and return a randomly-generated transaction String [] merchants = {"Amazon", "NewEgg", "Office Supplies Plus", "Pet Depot", "Cherry Computer", "Groceries R Us", "Gas N Go"}; String from = merchants[r.nextInt(merchants.length)]; int amountTemp = r.nextInt(50000) - 10000; double amount = amountTemp / 100.00; String type = ""; if (amount < 0) { type = "credit"; } else { type = "debit"; } return new Transaction(type, from, amount); } }
card data 1 .txt
8556022105572013 Mary_Miller 319 48900.0 8508800862328799 Joseph_Garcia 615 56400.0 8449757775821421 Susan_Williams 618 19500.0 8916165640442692 David_Brown 8856566249906322 Joseph_Jackson 8451660265733891 John_Jackson 1217 19200.0 8872896560788595 Patricia_Jones 8719263467960933 Barbara_Brown 619 67900.0 8479719735802785 Christopher_Thomas 215 63200.0 8906187402790575 Charles_Williams
card data 2.txt
8759584267314077 Margaret_Williams 216 4500.0 8581063421148570 William_Harris 1117 27300.0 8698805977296417 Susan_Taylor 1218 48800.0 8575451042939192 Barbara_Garcia 217 52100.0 8682059703866834 Richard_Taylor 418 2300.0 8936243436070492 Joseph_Miller 8795679644539289 Susan_White 819 64600.0 8962390562622049 James_Jones 8899342595966184 Richard_Brown 8479979080188788 Charles_White 419 68100.0 8668449391881086 Dorothy_Davis 1217 46700.0 8522467870270496 Mary_White 415 39700.0 8603002861451157 Christopher_Anderson 1117 41200.0 8978571163741023 William_Thompson 8574834270460638 Patricia_White 119 17700.0 8976449426358947 Linda_Thompson 8740008867464316 David_Brown 1219 30900.0 8603865194700047 Robert_Harris 415 10400.0 8458930310710342 Robert_Robinson 219 9700.0 8607038705438596 Christopher_Brown 515 52300.0 8524906615421823 Margaret_Wilson 1219 20500.0 8553132768960731 Christopher_Brown 817 20700.0 8920512504553108 Mary_Robinson 8909971450196719 Jennifer_White 8540673557146376 Christopher_Thompson 1218 32000.0 8640627513986761 Patricia_Moore 115 24900.0 8579311153854358 Richard_Thompson 118 29900.0 8984562839294673 Michael_Martinez 8535187875019814 Mary_Harris 1215 27500.0 8888691777988940 John_Jones 8489981925227953 Susan_Brown 317 59600.0 8715429616914016 Joseph_Moore 816 35500.0 8873009944682061 John_White 8879934483519616 Linda_White 8657755187767454 Mary_Johnson 1216 4100.0 8790698932254497 Patricia_Jackson 1117 16700.0 8806819751853938 Linda_Miller 8473474823659978 Barbara_Smith 317 17500.0 8556436322082842 Elizabeth_Johnson 218 29200.0 8931257730606420 David_Thompson 8947787973530216 David_Jackson 8638942563534615 Patricia_Taylor 919 41500.0 8603100485227884 Dorothy_Smith 1215 12300.0 8760957627014653 Linda_Thomas 1119 51900.0 8813591658082746 David_Taylor 8743716577743673 Robert_Jackson 1018 45400.0 8597982773221790 Charles_Brown 319 43700.0 8642541327644969 William_White 1016 21600.0 8909307355770740 John_Johnson 8510374578402963 William_Jones 917 30200.0 8400219437311688 Elizabeth_Harris 716 39200.0 8472089627481297 Maria_Robinson 615 41300.0 8918107127768116 Mary_Moore 8542624931903921 Robert_Robinson 918 43400.0 8466267549793959 William_Davis 718 28000.0 8902544285239126 Barbara_Johnson 8853180770348855 Robert_Brown 8541727209509208 Patricia_White 119 17700.0 8652104306470838 Joseph_Anderson 918 42100.0 8813372724353704 David_Brown
transaction data 1.txt
8916165640442692 top-up 800.0 8856566249906322 top-up 1600.0 8872896560788595 top-up 1900.0 8906187402790575 top-up 1500.0 8856566249906322 debit 52.39 Trask_Heavy_Industries 8856566249906322 credit -202.6 XYZ_Inc 8451660265733891 debit 140.29 Zorg_Corporation 8508800862328799 debit 238.72 XYZ_Inc 8449757775821421 credit -33.47 Acme 8906187402790575 credit -116.9 XYZ_Inc 8451660265733891 debit 46.48 Zorg_Corporation 8556022105572013 credit -241.2 Acme 8449757775821421 credit -246.71 Lemon_Computer 8856566249906322 debit 186.57 Trask_Heavy_Industries 8872896560788595 debit 92.52 Geek_Gear 8556022105572013 credit -5.8 Weyland_Research 8508800862328799 debit 171.16 Trask_Heavy_Industries 8451660265733891 credit -75.02 Lemon_Computer 8451660265733891 debit 122.87 Lemon_Computer 8451660265733891 debit 177.54 XYZ_Inc 8916165640442692 credit -234.07 SuperMegaCorp 8508800862328799 credit -61.2 Trask_Heavy_Industries 8479719735802785 credit -219.04 XYZ_Inc 8508800862328799 debit 174.08 Amazon 8856566249906322 credit -178.0 Gas_N_Go 8719263467960933 debit 173.66 Lemon_Computer 8449757775821421 debit 6.94 Amazon 8508800862328799 debit 134.14 Geek_Gear 8508800862328799 credit -169.56 SuperMegaCorp 8856566249906322 credit -168.78 XYZ_Inc 8451660265733891 credit -199.98 Lemon_Computer 8916165640442692 debit 30.8 Amazon 8719263467960933 debit 131.29 Amazon 8449757775821421 debit 43.19 Zorg_Corporation 8872896560788595 debit 227.99 Lemon_Computer 8556022105572013 debit 25.99 Amazon 8906187402790575 debit 235.44 Weyland_Research 8508800862328799 debit 150.87 XYZ_Inc 8508800862328799 credit -29.84 Zorg_Corporation 8451660265733891 credit -122.95 Trask_Heavy_Industries 8719263467960933 credit -122.61 Trask_Heavy_Industries 8916165640442692 credit -206.2 Acme 8906187402790575 credit -201.04 Weyland_Research 8451660265733891 credit -72.09 SuperMegaCorp 8916165640442692 credit -116.16 Geek_Gear 8906187402790575 debit 133.42 Lemon_Computer 8449757775821421 credit -203.24 Geek_Gear 8451660265733891 credit -102.66 Geek_Gear 8449757775821421 credit -58.0 SuperMegaCorp 8451660265733891 credit -123.86 Gas_N_Go
teansaction data 2.txt
8936243436070492 top-up 1600.0 8962390562622049 top-up 300.0 8899342595966184 top-up 1200.0 8978571163741023 top-up 1600.0 8976449426358947 top-up 1700.0 8920512504553108 top-up 600.0 8909971450196719 top-up 1100.0 8984562839294673 top-up 1500.0 8888691777988940 top-up 500.0 8873009944682061 top-up 800.0 8879934483519616 top-up 1100.0 8806819751853938 top-up 1700.0 8931257730606420 top-up 1500.0 8947787973530216 top-up 300.0 8813591658082746 top-up 1800.0 8909307355770740 top-up 1000.0 8918107127768116 top-up 1800.0 8902544285239126 top-up 1100.0 8853180770348855 top-up 1500.0 8813372724353704 top-up 1900.0 8743716577743673 credit -35.16 Amazon 8759584267314077 credit -144.35 Lemon_Computer 8542624931903921 debit 173.86 Gas_N_Go 8790698932254497 credit -84.21 SuperMegaCorp 8603002861451157 debit 131.26 Gas_N_Go 8510374578402963 debit 148.28 Geek_Gear 8813591658082746 credit -73.34 Geek_Gear 8579311153854358 credit -165.46 Acme 8466267549793959 debit 82.87 Weyland_Research 8597982773221790 debit 205.71 Acme 8489981925227953 debit 20.74 Gas_N_Go 8458930310710342 credit -95.8 Lemon_Computer 8888691777988940 debit 69.29 Geek_Gear 8899342595966184 credit -75.47 XYZ_Inc 8759584267314077 credit -14.8 Geek_Gear 8640627513986761 credit -194.27 Gas_N_Go 8899342595966184 credit -130.22 Amazon 8902544285239126 credit -207.58 Gas_N_Go 8740008867464316 credit -16.35 Zorg_Corporation 8638942563534615 credit -68.27 Geek_Gear 8873009944682061 debit 57.14 Lemon_Computer 8873009944682061 debit 156.31 Acme 8489981925227953 debit 131.98 Weyland_Research 8888691777988940 credit -133.57 Gas_N_Go 8638942563534615 debit 20.22 Trask_Heavy_Industries 8902544285239126 debit 123.84 Acme 8947787973530216 debit 237.54 Lemon_Computer 8976449426358947 credit -121.57 Amazon 8936243436070492 credit -68.53 XYZ_Inc 8873009944682061 credit -7.56 Geek_Gear 8682059703866834 credit -233.31 Geek_Gear 8813372724353704 credit -140.78 Geek_Gear 8760957627014653 debit 217.94 XYZ_Inc 8888691777988940 credit -69.25 Geek_Gear 8638942563534615 credit -234.1 SuperMegaCorp 8581063421148570 debit 117.68 XYZ_Inc 8918107127768116 top-up 1900.0 8813591658082746 top-up 300.0 8603100485227884 debit 192.45 Lemon_Computer 8931257730606420 credit -118.62 Trask_Heavy_Industries 8540673557146376 debit 92.41 Weyland_Research 8466267549793959 credit -100.46 Gas_N_Go 8640627513986761 redeem 4055 8638942563534615 debit 29.1 SuperMegaCorp 8510374578402963 credit -71.99 Geek_Gear 8698805977296417 credit -126.95 SuperMegaCorp 8540673557146376 debit 184.31 Lemon_Computer 8524906615421823 credit -118.92 Amazon 8984562839294673 debit 81.13 SuperMegaCorp 8603002861451157 credit -132.05 Geek_Gear 8909307355770740 debit 117.16 Gas_N_Go 8790698932254497 debit 235.56 Gas_N_Go 8813591658082746 debit 27.26 Lemon_Computer 8936243436070492 credit -242.04 XYZ_Inc 8510374578402963 debit 187.3 Geek_Gear 8931257730606420 debit 99.01 Acme 8909971450196719 credit -214.72 Lemon_Computer 8888691777988940 top-up 2000.0 8909307355770740 credit -195.33 Geek_Gear 8657755187767454 debit 63.31 SuperMegaCorp 8489981925227953 credit -137.92 XYZ_Inc 8715429616914016 debit 14.02 Trask_Heavy_Industries 8640627513986761 debit 170.09 Weyland_Research 8909971450196719 credit -217.53 Gas_N_Go 8579311153854358 credit -85.94 Trask_Heavy_Industries 8715429616914016 redeem 575 8640627513986761 credit -229.85 XYZ_Inc 8524906615421823 debit 188.06 Lemon_Computer 8853180770348855 credit -248.82 XYZ_Inc 8899342595966184 debit 58.97 Amazon 8936243436070492 top-up 1500.0 8574834270460638 credit -117.6 Acme 8472089627481297 debit 180.92 XYZ_Inc 8524906615421823 debit 171.69 Zorg_Corporation 8931257730606420 credit -155.33 Gas_N_Go 8542624931903921 debit 79.18 Trask_Heavy_Industries 8909307355770740 debit 67.87 XYZ_Inc 8978571163741023 top-up 600.0 8489981925227953 debit 49.77 Zorg_Corporation 8715429616914016 debit 179.71 Amazon 8522467870270496 credit -66.09 XYZ_Inc 8400219437311688 credit -103.79 Gas_N_Go 8535187875019814 credit -124.14 Amazon 8759584267314077 debit 55.11 XYZ_Inc 8873009944682061 debit 81.53 Zorg_Corporation 8603865194700047 debit 70.42 Zorg_Corporation 8978571163741023 debit 109.35 Acme 8978571163741023 debit 19.04 SuperMegaCorp 8458930310710342 credit -114.63 Weyland_Research 8603002861451157 debit 70.17 SuperMegaCorp 8743716577743673 redeem 4523 8853180770348855 debit 38.25 XYZ_Inc 8790698932254497 credit -35.07 Gas_N_Go 8642541327644969 debit 6.33 Gas_N_Go 8472089627481297 debit 34.37 Trask_Heavy_Industries 8853180770348855 top-up 1100.0 8510374578402963 credit -223.13 Zorg_Corporation 8472089627481297 credit -165.18 SuperMegaCorp 8902544285239126 debit 96.42 Geek_Gear 8947787973530216 top-up 1600.0 8978571163741023 credit -154.45 Zorg_Corporation 8458930310710342 credit -18.5 Zorg_Corporation 8909971450196719 debit 179.64 Weyland_Research 8668449391881086 credit -109.18 Amazon 8936243436070492 debit 35.18 Gas_N_Go 8541727209509208 credit -133.41 Zorg_Corporation 8795679644539289 debit 187.81 Weyland_Research 8962390562622049 debit 32.06 Gas_N_Go 8759584267314077 credit -182.04 XYZ_Inc 8976449426358947 top-up 600.0 8873009944682061 credit -165.34 Amazon 8795679644539289 credit -52.0 Amazon 8579311153854358 credit -24.74 Amazon 8642541327644969 credit -31.07 Gas_N_Go 8743716577743673 credit -34.1 XYZ_Inc 8638942563534615 debit 158.43 SuperMegaCorp 8682059703866834 debit 142.07 Zorg_Corporation 8603100485227884 debit 28.99 Gas_N_Go 8909307355770740 debit 151.95 Weyland_Research 8466267549793959 credit -116.79 Weyland_Research 8556436322082842 debit 67.07 Acme 8657755187767454 debit 252.21 Geek_Gear 8638942563534615 debit 24.88 SuperMegaCorp 8581063421148570 credit -50.45 Acme 8400219437311688 credit -183.21 XYZ_Inc 8962390562622049 credit -142.17 Trask_Heavy_Industries 8936243436070492 debit 94.65 XYZ_Inc 8540673557146376 credit -189.73 Lemon_Computer 8489981925227953 debit 206.87 Zorg_Corporation 8473474823659978 debit 149.91 Amazon 8640627513986761 redeem 2752 8931257730606420 debit 174.97 SuperMegaCorp 8813591658082746 credit -19.07 SuperMegaCorp 8936243436070492 credit -185.86 XYZ_Inc 8682059703866834 redeem 1131 8489981925227953 debit 47.54 Geek_Gear 8638942563534615 redeem 4453 8931257730606420 debit 184.14 Trask_Heavy_Industries 8542624931903921 credit -9.05 SuperMegaCorp 8902544285239126 credit -102.03 Geek_Gear 8489981925227953 credit -206.89 XYZ_Inc 8581063421148570 debit 162.61 Acme 8540673557146376 credit -173.62 Lemon_Computer 8603002861451157 credit -130.91 Lemon_Computer 8984562839294673 debit 11.0 Amazon 8638942563534615 credit -85.48 Weyland_Research 8489981925227953 credit -15.74 Geek_Gear 8400219437311688 debit 174.74 SuperMegaCorp 8540673557146376 credit -24.05 Acme 8790698932254497 credit -188.58 Zorg_Corporation 8899342595966184 top-up 1600.0 8489981925227953 credit -232.8 Acme 8603100485227884 debit 139.87 Trask_Heavy_Industries 8759584267314077 debit 79.71 Zorg_Corporation 8920512504553108 credit -157.12 Acme 8603100485227884 credit -19.78 Trask_Heavy_Industries 8657755187767454 credit -201.84 Zorg_Corporation 8740008867464316 credit -149.68 Trask_Heavy_Industries 8879934483519616 debit 182.12 Trask_Heavy_Industries 8657755187767454 debit 55.18 Geek_Gear 8603865194700047 debit 220.02 Lemon_Computer 8652104306470838 redeem 1233 8638942563534615 credit -52.33 Weyland_Research 8682059703866834 debit 193.5 Acme 8607038705438596 credit -139.44 SuperMegaCorp 8909307355770740 credit -90.55 Weyland_Research 8976449426358947 debit 35.88 XYZ_Inc 8524906615421823 credit -51.2 Weyland_Research 8936243436070492 debit 102.56 Lemon_Computer 8510374578402963 debit 239.83 Zorg_Corporation 8603865194700047 redeem 655 8879934483519616 debit 57.76 Trask_Heavy_Industries 8795679644539289 debit 39.27 Amazon 8931257730606420 credit -119.45 Weyland_Research 8603002861451157 credit -10.61 XYZ_Inc 8579311153854358 credit -5.06 Weyland_Research 8472089627481297 debit 122.32 SuperMegaCorp 8574834270460638 credit -65.33 Acme 8909307355770740 debit 200.92 SuperMegaCorp 8575451042939192 debit 109.52 Lemon_Computer 8603100485227884 debit 38.79 Geek_Gear 8813372724353704 credit -70.01 XYZ_Inc 8813372724353704 credit -218.96 XYZ_Inc 8541727209509208 credit -122.49 XYZ_Inc 8652104306470838 credit -179.84 XYZ_Inc 8535187875019814 debit 118.42 Geek_Gear 8715429616914016 credit -136.75 Gas_N_Go 8918107127768116 credit -148.41 Gas_N_Go 8920512504553108 credit -63.36 Acme 8902544285239126 credit -254.67 Lemon_Computer 8813372724353704 credit -71.64 Amazon 8899342595966184 credit -91.39 SuperMegaCorp 8920512504553108 debit 107.07 Lemon_Computer 8603002861451157 debit 68.58 Trask_Heavy_Industries 8888691777988940 debit 46.71 Amazon 8873009944682061 credit -111.57 Geek_Gear 8553132768960731 debit 136.3 Gas_N_Go 8899342595966184 credit -251.95 Acme 8909307355770740 debit 83.67 Lemon_Computer 8652104306470838 credit -23.8 Geek_Gear 8510374578402963 credit -97.89 Amazon 8920512504553108 debit 184.9 Lemon_Computer 8541727209509208 debit 209.62 Geek_Gear 8522467870270496 debit 77.71 Geek_Gear 8853180770348855 debit 129.57 Gas_N_Go 8899342595966184 top-up 1700.0 8899342595966184 credit -211.33 Gas_N_Go 8642541327644969 credit -190.58 SuperMegaCorp 8899342595966184 credit -142.18 Trask_Heavy_Industries 8479979080188788 credit -109.21 Trask_Heavy_Industries 8579311153854358 debit 47.01 Zorg_Corporation 8556436322082842 credit -219.45 Lemon_Computer 8607038705438596 debit 10.95 Amazon 8984562839294673 credit -156.09 Weyland_Research 8759584267314077 credit -135.4 SuperMegaCorp 8920512504553108 debit 254.81 Weyland_Research 8888691777988940 credit -251.45 XYZ_Inc 8918107127768116 top-up 400.0 8638942563534615 credit -183.59 Weyland_Research 8541727209509208 credit -154.44 Amazon 8607038705438596 credit -218.61 Gas_N_Go 8652104306470838 debit 127.23 XYZ_Inc 8909971450196719 top-up 1800.0 8642541327644969 credit -58.66 Weyland_Research 8902544285239126 credit -117.66 Acme 8489981925227953 credit -84.77 Geek_Gear 8603865194700047 credit -81.87 XYZ_Inc 8743716577743673 redeem 1207 8581063421148570 credit -32.46 Lemon_Computer 8947787973530216 debit 8.08 Gas_N_Go 8759584267314077 credit -141.77 Lemon_Computer 8472089627481297 debit 65.51 Gas_N_Go 8760957627014653 debit 175.92 Trask_Heavy_Industries 8466267549793959 debit 231.63 Acme 8603100485227884 debit 234.45 SuperMegaCorp 8813591658082746 debit 34.4 Trask_Heavy_Industries 8581063421148570 debit 135.18 Zorg_Corporation 8603100485227884 credit -52.67 Gas_N_Go 8597982773221790 debit 145.68 Acme 8873009944682061 credit -218.28 Acme 8715429616914016 debit 211.01 Zorg_Corporation 8553132768960731 credit -150.2 Acme 8522467870270496 credit -19.51 Weyland_Research 8909971450196719 debit 6.95 Geek_Gear 8879934483519616 debit 77.7 Lemon_Computer 8740008867464316 debit 213.31 Zorg_Corporation 8640627513986761 credit -31.89 Weyland_Research 8553132768960731 credit -174.95 Acme 8740008867464316 debit 139.13 Weyland_Research 8909971450196719 debit 33.24 Gas_N_Go 8472089627481297 debit 67.28 Geek_Gear 8947787973530216 debit 115.51 Amazon 8853180770348855 credit -33.58 Trask_Heavy_Industries 8574834270460638 credit -238.76 Acme 8715429616914016 redeem 658 8535187875019814 credit -104.32 Acme 8607038705438596 debit 133.81 Lemon_Computer 8962390562622049 credit -124.11 Zorg_Corporation 8603865194700047 debit 149.25 Geek_Gear 8984562839294673 debit 231.98 Gas_N_Go 8541727209509208 debit 210.65 SuperMegaCorp 8806819751853938 debit 231.17 Gas_N_Go 8542624931903921 credit -64.89 Weyland_Research 8790698932254497 credit -45.45 Weyland_Research 8698805977296417 debit 125.65 XYZ_Inc 8607038705438596 debit 129.86 Trask_Heavy_Industries 8574834270460638 debit 179.36 Trask_Heavy_Industries 8698805977296417 credit -233.92 Acme 8479979080188788 credit -110.63 Weyland_Research 8806819751853938 debit 65.31 Geek_Gear 8978571163741023 credit -195.28 Acme 8873009944682061 top-up 1300.0 8535187875019814 debit 243.58 Lemon_Computer 8575451042939192 credit -15.13 XYZ_Inc 8575451042939192 credit -142.28 XYZ_Inc 8918107127768116 debit 74.29 Acme 8652104306470838 debit 242.77 Acme 8813372724353704 top-up 900.0 8542624931903921 debit 234.81 Acme 8458930310710342 debit 144.32 Lemon_Computer 8760957627014653 credit -221.65 Trask_Heavy_Industries 8541727209509208 credit -57.59 Geek_Gear 8603002861451157 debit 67.07 Amazon 8879934483519616 credit -219.27 Acme 8740008867464316 debit 89.58 Lemon_Computer 8759584267314077 debit 167.61 Gas_N_Go 8740008867464316 credit -192.54 Gas_N_Go 8760957627014653 credit -171.75 Zorg_Corporation 8873009944682061 debit 122.89 Gas_N_Go 8553132768960731 credit -17.75 Geek_Gear 8574834270460638 debit 22.83 Zorg_Corporation 8813372724353704 top-up 800.0 8581063421148570 credit -130.57 Trask_Heavy_Industries 8936243436070492 debit 144.97 XYZ_Inc 8575451042939192 debit 107.02 Lemon_Computer 8540673557146376 debit 113.26 SuperMegaCorp 8522467870270496 debit 158.0 Gas_N_Go 8535187875019814 credit -149.77 Weyland_Research 8947787973530216 debit 149.29 Trask_Heavy_Industries 8795679644539289 credit -153.48 Gas_N_Go 8522467870270496 debit 79.49 Geek_Gear 8760957627014653 debit 71.97 Zorg_Corporation 8472089627481297 debit 103.53 Trask_Heavy_Industries 8740008867464316 credit -94.81 Acme 8668449391881086 credit -122.69 Amazon 8466267549793959 credit -105.46 SuperMegaCorp 8795679644539289 debit 138.58 Weyland_Research 8795679644539289 credit -133.46 Trask_Heavy_Industries 8899342595966184 credit -178.61 Amazon 8888691777988940 top-up 600.0 8400219437311688 debit 226.08 Amazon 8556436322082842 credit -191.93 XYZ_Inc 8978571163741023 debit 199.77 Trask_Heavy_Industries 8743716577743673 redeem 973 8698805977296417 debit 161.49 Amazon 8790698932254497 redeem 227 8760957627014653 credit -6.93 Geek_Gear 8574834270460638 credit -236.19 Weyland_Research 8541727209509208 credit -182.33 XYZ_Inc 8931257730606420 debit 13.64 Weyland_Research 8603002861451157 credit -210.96 Gas_N_Go 8579311153854358 credit -49.82 Acme 8976449426358947 debit 91.5 Amazon 8806819751853938 credit -182.44 Trask_Heavy_Industries 8962390562622049 credit -43.62 XYZ_Inc 8888691777988940 credit -128.74 Weyland_Research 8466267549793959 credit -237.42 Zorg_Corporation 8489981925227953 credit -242.22 Acme 8920512504553108 debit 56.61 Trask_Heavy_Industries 8553132768960731 debit 135.29 Amazon 8603865194700047 redeem 1424 8489981925227953 credit -141.21 Lemon_Computer 8902544285239126 credit -136.96 Zorg_Corporation 8642541327644969 debit 103.64 Zorg_Corporation 8790698932254497 debit 34.89 Zorg_Corporation 8962390562622049 credit -62.49 Lemon_Computer 8522467870270496 debit 171.19 Geek_Gear 8920512504553108 credit -94.46 Amazon 8466267549793959 credit -6.18 Geek_Gear 8607038705438596 credit -101.58 Zorg_Corporation 8603002861451157 debit 241.32 XYZ_Inc 8962390562622049 debit 153.64 Gas_N_Go 8795679644539289 debit 179.8 Acme 8466267549793959 debit 31.09 Amazon 8542624931903921 debit 249.42 Zorg_Corporation 8542624931903921 debit 189.62 SuperMegaCorp 8522467870270496 debit 119.88 Geek_Gear 8579311153854358 debit 141.39 Acme 8715429616914016 debit 225.28 Trask_Heavy_Industries 8920512504553108 credit -138.76 Trask_Heavy_Industries 8473474823659978 credit -27.83 Gas_N_Go 8879934483519616 credit -129.07 Zorg_Corporation 8760957627014653 credit -111.51 XYZ_Inc 8853180770348855 debit 117.83 Acme 8603002861451157 redeem 1009 8909971450196719 debit 194.6 Gas_N_Go 8899342595966184 credit -63.14 Zorg_Corporation 8542624931903921 credit -12.78 Trask_Heavy_Industries 8760957627014653 redeem 1764 8458930310710342 debit 57.0 Lemon_Computer 8553132768960731 credit -17.29 Amazon 8603865194700047 debit 28.37 Geek_Gear 8556436322082842 credit -44.51 Gas_N_Go 8535187875019814 credit -102.52 Acme 8510374578402963 credit -156.96 Gas_N_Go 8888691777988940 credit -207.12 Amazon 8813591658082746 debit 254.47 Gas_N_Go 8489981925227953 debit 201.11 Weyland_Research 8473474823659978 credit -147.83 Geek_Gear 8936243436070492 credit -150.42 Trask_Heavy_Industries 8909307355770740 credit -112.75 Trask_Heavy_Industries 8556436322082842 debit 181.08 Gas_N_Go 8466267549793959 credit -5.03 XYZ_Inc 8909971450196719 debit 71.55 Amazon 8947787973530216 debit 86.0 Weyland_Research 8931257730606420 debit 45.33 SuperMegaCorp 8579311153854358 credit -218.38 Acme 8466267549793959 credit -212.04 SuperMegaCorp 8472089627481297 credit -203.33 XYZ_Inc 8920512504553108 credit -62.66 SuperMegaCorp
Step by Step Solution
There are 3 Steps involved in it
Step: 1
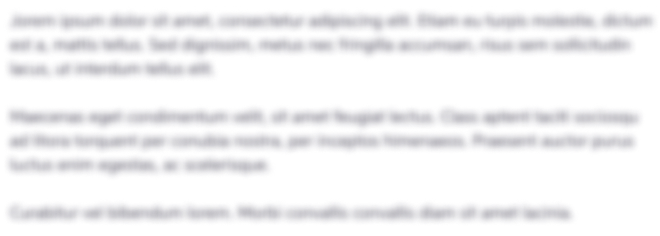
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started