Question
CSIS C++ - Polymorphism In lab 11 you created a class called Number that was derived from string and was used as a base class
CSIS C++ - Polymorphism
In lab 11 you created a class called Number that was derived from string and was used as a base class for both Integer and Double. In this assignment you are going to be asked to add some functionality to the Number class.
As different number types are being derived it may be necessary for some to need their own toString function. Create a virtual toString function that returns the data section as a string. You have already written the toString function in both of your classes. To get rid of the duplicate code we want to move the common code to the base class. Simply remove these functions from the Integer and Double classes and move one of them to the Number class. You should try to keep in mind the "isa" relationship here. You are being asked to return a string and the reality is that Number now "isa" string.
You currently have overloaded isNaN functions in both the Integer and Double classes. We want to get rid of some of this duplicate functionality. Move the no argument isNaN function and the nan variable to the Number class and out of Integer and Double.
The isNaN function that takes a string is something that will need to be handled differently by the different number types derived from Number. Make the isNaN function a virtual function in the Number class and have it call the recursiveNaN functions you created in the Integer and Double classes. Because of the way polymorphism works once isNaN is called it will then call the correct recursiveNaN function.
This is my lab 11 code(that i am supposed to work off of):
Double.h
#ifndef DOUBLE_H
#define DOUBLE_H
#include
#include "Number.h"
using std::string;
class Double :public Numbers
{
private:
void NaN(string s);
bool nan;
public:
Double() :Numbers() {};
Double(double d);
Double(const Double&d) : Numbers(d.data()) {};
Double(const Integer&i) : Numbers(i.data()) {};
Double(string s);
void equals(double d);
void equals(string s);
Double add(const Double &d);
Double sub(const Double &d);
Double mul(const Double &d);
Double div(const Double &d);
Double add(double d);
Double sub(double d);
Double mul(double d);
Double div(double d);
double toDbl() const;
Double operator + (const Double &d);
Double operator - (const Double &d);
Double operator * (const Double &d);
Double operator / (const Double &d);
string toString() const;
Double &operator =(double d);
Double &operator =(const Double &d);
Double &operator = (const string &s);
bool operator == (const Double &d);
bool operator == (double d);
bool operator !=(const Double &d);
bool operator !=(double d);
bool isNan();
};
#endif
Double.cpp
#include
#include"Double.h"
#include
#include "Integer.h"
using namespace std;
Double::Double(double d)
: Numbers(std::to_string(d)), nan(false)
{
}
Double::Double(string s)
{
this->equals(s);
}
void Double::equals(double d)
{
*this = std::to_string(d);
this->nan = false;
}
void Double::equals(string s)
{
this->NaN(s);
if (!this->isNan())
this->assign(s);
else
this->assign("0.0");
}
Double Double::add(const Double &d)
{
return add(d.toDbl());
}
Double Double::add(double d)
{
return Double(this->toDbl() + d);
}
Double Double::sub(const Double &d)
{
Double tmp;
*tmp.data = data* - *d.data;
return tmp;
}
Double Double::sub(double d)
{
Double tmp;
tmp.equals(this->toDbl() - d);
return tmp;
}
Double Double::mul(const Double &d)
{
Double tmp;
*tmp.data = data * * *d.data;
return tmp;
}
Double Double::mul(double d)
{
Double tmp;
tmp.equals(this->toDbl()* d);
return tmp;
}
Double Double::div(const Double &d)
{
Double tmp;
*tmp.data = data / *d.data;
return tmp;
}
Double Double::div(double d)
{
Double tmp;
tmp.equals(this->toDbl() / d);
return tmp;
}
double Double::toDbl() const
{
return std::stod(*this);
}
Double Double::operator + (const Double &d)
{
return this->add(d);
}
Double Double::operator - (const Double & d)
{
return this->sub(d);
}
Double Double::operator * (const Double & d)
{
return this->mul(d);
}
Double Double::operator / (const Double &d)
{
return this->div(d);
}
Double &Double::operator = (const Double &d)
{
equals(d);
return *this;
}
Double &Double::operator =(double d)
{
this->equals(d);
return *this;
}
Double &Double::operator = (const string &s)
{
equals(s);
return *this;
}
bool Double::operator == (const Double &d)
{
return this->compare(d) == 0;
}
bool Double::operator == (double d)
{
return operator == (Double(d));
}
bool Double::operator != (const Double &d)
{
}
bool Double::operator != (double d)
{
return *this->data != d;
}
string Double::toString() const
{
return *this;
}
bool Double::isNan()
{
return this->nan;
}
void Double::NaN(string s)
{
int pos;
pos = s.find(" . ", 0);
if (pos != string::npos)
{
pos = s.find(" . ", pos + 1);
if (pos != string::npos)
{
this->nan = true;
return;
}
}
string::iterator p;
for (p = s.begin(); p < s.end(); p++)
{
if (!isdigit(*p) && *p != ' . ')
{
this->nan = true;
return;
}
}
this->nan = false;
return;
};
Integer.h
#ifndef INTEGER_H
#define INTEGER_H
#include
#include "Number.h"
using std::string;
class Integer :public Numbers
{
private:
bool nan;
void Nan(string str);
public:
Integer() :Numbers() {};
Integer(int i);
Integer(const Integer &i) : Numbers(i.data()) {};
Integer(const Double & d) : Numbers(d.data()) {};
Integer(string s);
void equals(double d);
void equals(string s);
Double add(const Double &d);
Double sub(const Double &d);
Double mul(const Double &d);
Double div(const Double &d);
void equals(int i);
void equals(string s);
Integer add(const Integer &i);
Integer sub(const Integer &i);
Integer mul(const Integer &i);
Integer div(const Integer &i);
Integer add(int i);
Integer sub(int i);
Integer mul(int i);
Integer div(int i);
int toInt() const;
Integer operator +(const Integer &i);
Integer operator -(const Integer &i);
Integer operator *(const Integer &i);
Integer operator /(const Integer &i);
string toString() const;
Integer &operator =(int i);
Integer &operator =(const Integer &i);
Integer &operator =(const string &s);
bool operator ==(const int &i);
bool operator ==(int i);
bool operator !=(const Integer &i);
bool operator !=(int i);
bool isNan();
};
#endif
Integer.cpp
#include
#include"Integer.h"
#include
#include "Double.h"
using namespace std;
Integer::Integer(int i)
:Numbers(std::to_string(i)), nan(false)
{
}
Integer::Integer(string s)
{
this->equals(s);
}
void Integer::equals(double i)
{
*this = std::to_string(i);
this->nan = false;
}
void Integer::equals(string s)
{
this->NaN(s);
if (!this->isNan())
this->assign(s);
this->assign("0.0");
}
Integer Integer::add(const Integer &i)
{
return add(i.toInt());
}
Integer Integer::add(int i)
{
return Integer(this->toInt() + i);
}
Integer Integer::sub(const Integer &i)
{
Integer tmp;
*tmp.data = data* - *i.data;
return tmp;
}
Integer Integer::sub(int i)
{
Integer tmp;
tmp.equals(this->toInt() - i);
return tmp;
}
Integer Integer::mul(const Integer &i)
{
Integer tmp;
*tmp.data = data* * *i.data;
return tmp;
}
Integer Integer::mul(int i)
{
Integer tmp;
tmp.equals(this->toInt()* i);
return tmp;
}
Integer Integer::div(const Integer &i)
{
Integer tmp;
*tmp.data = data / *i.data;
return tmp;
}
Integer Integer::div(int i)
{
Integer tmp;
tmp.equals(this->toInt() / i);
return tmp;
}
int Integer::toInt()const
{
return std::stod(*this);
}
Integer Integer::operator + (const Integer &i)
{
return this->add(i);
}
Integer Integer::operator - (const Integer &i)
{
return this->sub(i);
}
Integer Integer::operator * (const Integer & i)
{
return this->mul(i);
}
Integer Integer::operator / (const Integer & i)
{
return this->div(i);
}
Integer & Integer::operator = (const Integer& i)
{
equals(i);
return *this;
}
Integer & Integer::operator=(int i)
{
this->equals(i);
return *this;
}
Integer & Integer::operator = (const string &s)
{
equals(s);
return *this;
}
bool Integer::operator == (const Integer &i)
{
return this->compare(i) == 0;
}
bool Integer::operator == (int i)
{
return this-> operator == (Integer(i));
}
bool Integer::operator != (const Integer &i)
{
}
bool Integer::operator != (int i)
{
return *this->data != i;
}
string Integer::toString() const
{
return *this;
}
bool Integer::isNan()
{
return this->nan;
}
void Integer::NaN(string s)
{
int pos;
pos = s.find(" . ", 0);
if (pos != string::npos)
{
pos = s.find(" . ", pos + 1);
if (pos != string::npos)
{
this->nan = true;
return;
}
}
string::iterator p;
for (p = s.begin(); p < s.end(); p++)
{
if (!isdigit(*p) && *p != ' . ')
{
this->nan = true;
return;
}
}
this->nan = false;
return;
};
Number.h
#ifndef NUMBERS_H
#define NUMBERS_H
#include
using std::string;
class Numbers : public string
{
public:
Numbers() { this->assign("0"); }
Numbers(string str) { this->assign(str); }
};
#endif
Main.cpp
#include
#include "Double.h"
#include "Integer.h"
using namespace std;
int main()
{
Double D("17");
Double E(16);
if (D == E)
cout << "true" << endl;
else
cout << "false" << endl;
return 0;
}
I would really appreciate some help. Thank You!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
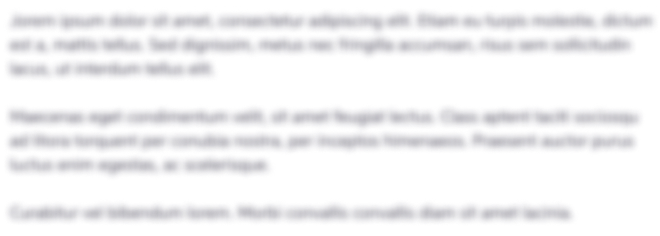
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started