Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Data Abstraction & Problem Solving with CFrank M. Carrano; Timothy M. Henry 1 /** @file BagInterface.h */ 2 #ifndef BAG_INTERFACE_ 3 #define BAG_INTERFACE_ 4 5
Data Abstraction & Problem Solving with CFrank M. Carrano; Timothy M. Henry
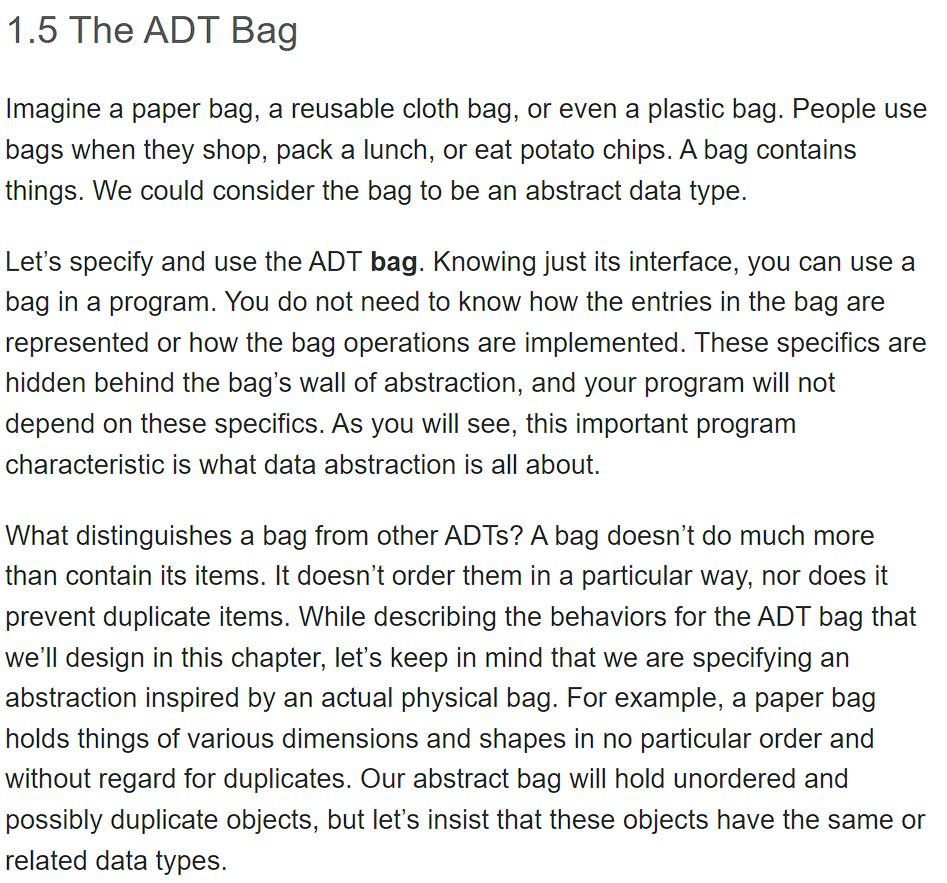
1 /** @file BagInterface.h */
2 #ifndef BAG_INTERFACE_
3 #define BAG_INTERFACE_
4
5 #include
6
7 template
8 class BagInterface
9 {
10 public:
11 /** Gets the current number of entries in this bag.
12 @return The integer number of entries currently in the bag. */
13 virtual int getCurrentSize() const = 0;
14
15 /** Sees whether this bag is empty.
16 @return True if the bag is empty, or false if not. */
17 virtual bool isEmpty() const = 0;
18
19 /** Adds a new entry to this bag.
20 @post If successful, newEntry is stored in the bag and
21 the count of items in the bag has increased by 1.
22 @param newEntry The object to be added as a new entry.
23 @return True if addition was successful, or false if not. */
24 virtual bool add(const ItemType& newEntry) = 0;
25
26 /** Removes one occurrence of a given entry from this bag,
27 if possible.
28 @post If successful, anEntry has been removed from the bag
29 and the count of items in the bag has decreased by 1.
30 @param anEntry The entry to be removed.
31 @return True if removal was successful, or false if not. */
32 virtual bool remove(const ItemType& anEntry) = 0;
33
34 /** Removes all entries from this bag.
35 @post Bag contains no items, and the count of items is 0. */
36 virtual void clear() = 0;
37
38 /** Counts the number of times a given entry appears in this bag.
39 @param anEntry The entry to be counted.
40 @return The number of times anEntry appears in the bag. */
41 virtual int getFrequencyOf(const ItemType& anEntry) const = 0;
42
43 /** Tests whether this bag contains a given entry.
44 @param anEntry The entry to locate.
45 @return True if bag contains anEntry, or false otherwise. */
46 virtual bool contains(const ItemType& anEntry) const = 0;
47
48 /** Empties and then fills a given vector with all entries that
49 are in this bag.
50 @return A vector containing copies of all the entries in this bag. */
51 virtual std::vector toVector() const = 0;
52
53 /** Destroys this bag and frees its assigned memory. (See C++ Interlude 2.) */
54 virtual ~BagInterface() { }
55 }; // end BagInterface
2 #ifndef BAG_INTERFACE_
3 #define BAG_INTERFACE_
4
5 #include
6
7 template
8 class BagInterface
9 {
10 public:
11 /** Gets the current number of entries in this bag.
12 @return The integer number of entries currently in the bag. */
13 virtual int getCurrentSize() const = 0;
14
15 /** Sees whether this bag is empty.
16 @return True if the bag is empty, or false if not. */
17 virtual bool isEmpty() const = 0;
18
19 /** Adds a new entry to this bag.
20 @post If successful, newEntry is stored in the bag and
21 the count of items in the bag has increased by 1.
22 @param newEntry The object to be added as a new entry.
23 @return True if addition was successful, or false if not. */
24 virtual bool add(const ItemType& newEntry) = 0;
25
26 /** Removes one occurrence of a given entry from this bag,
27 if possible.
28 @post If successful, anEntry has been removed from the bag
29 and the count of items in the bag has decreased by 1.
30 @param anEntry The entry to be removed.
31 @return True if removal was successful, or false if not. */
32 virtual bool remove(const ItemType& anEntry) = 0;
33
34 /** Removes all entries from this bag.
35 @post Bag contains no items, and the count of items is 0. */
36 virtual void clear() = 0;
37
38 /** Counts the number of times a given entry appears in this bag.
39 @param anEntry The entry to be counted.
40 @return The number of times anEntry appears in the bag. */
41 virtual int getFrequencyOf(const ItemType& anEntry) const = 0;
42
43 /** Tests whether this bag contains a given entry.
44 @param anEntry The entry to locate.
45 @return True if bag contains anEntry, or false otherwise. */
46 virtual bool contains(const ItemType& anEntry) const = 0;
47
48 /** Empties and then fills a given vector with all entries that
49 are in this bag.
50 @return A vector containing copies of all the entries in this bag. */
51 virtual std::vector
52
53 /** Destroys this bag and frees its assigned memory. (See C++ Interlude 2.) */
54 virtual ~BagInterface() { }
55 }; // end BagInterface
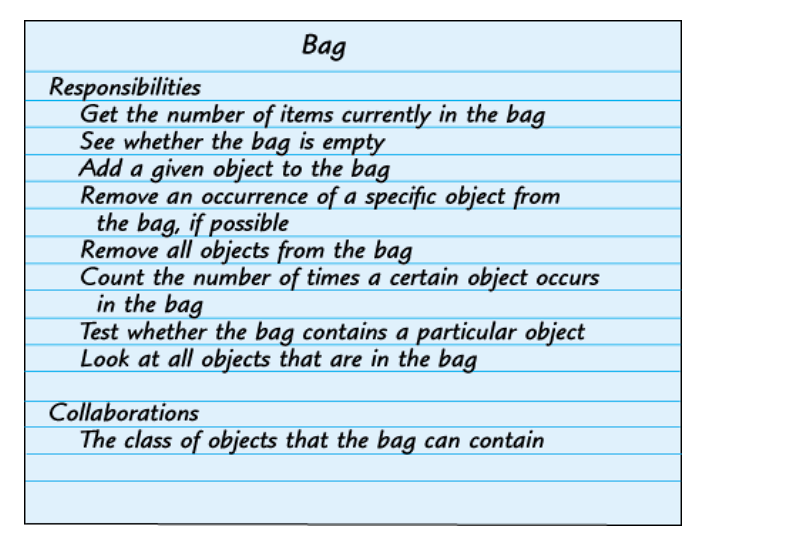
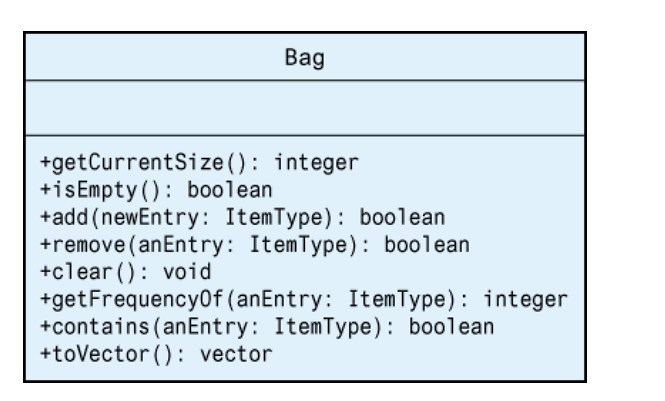
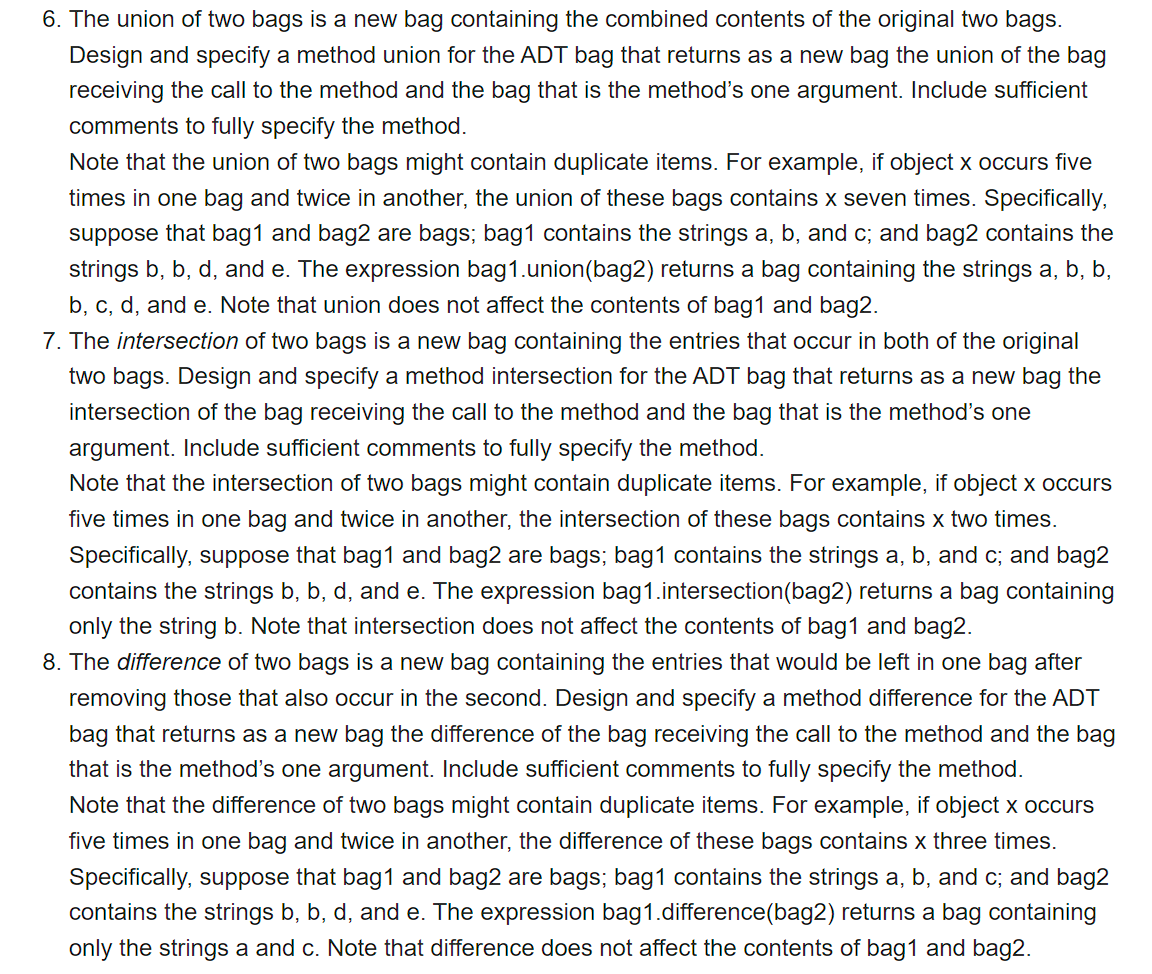
implement the many bag operations: union, intersection, and difference. Make the three functions as member functions, have three files BagInterface.h , Bag.h, and Bag.cpp. Keep these separated.
Give a contract for each function created and make a tester as well
1.5 The ADT Bag Imagine a paper bag, a reusable cloth bag, or even a plastic bag. People use bags when they shop, pack a lunch, or eat potato chips. A bag contains things. We could consider the bag to be an abstract data type. Let's specify and use the ADT bag. Knowing just its interface, you can use a bag in a program. You do not need to know how the entries in the bag are represented or how the bag operations are implemented. These specifics are hidden behind the bag's wall of abstraction, and your program will not depend on these specifics. As you will see, this important program characteristic is what data abstraction is all about. What distinguishes a bag from other ADTS? A bag doesn't do much more than contain its items. It doesn't order them in a particular way, nor does it prevent duplicate items. While describing the behaviors for the ADT bag that we'll design in this chapter, let's keep in mind that we are specifying an abstraction inspired by an actual physical bag. For example, a paper bag holds things of various dimensions and shapes in no particular order and without regard for duplicates. Our abstract bag will hold unordered and possibly duplicate objects, but let's insist that these objects have the same or related data types.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
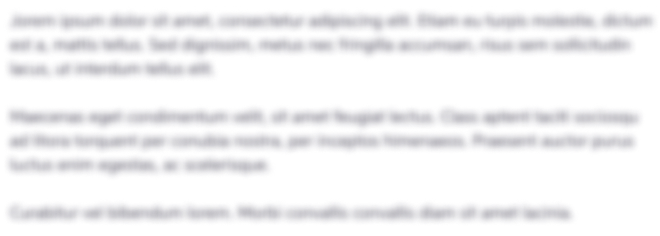
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started