Question
[Data Structure C++] Need help finishing this exercise. <3 Excercise description : Write a pseudocode function that uses LinkedStack to determine whether a string is
[Data Structure C++] Need help finishing this exercise. <3
Excercise description: Write a pseudocode function that uses LinkedStack to determine whether a string is in the language L, where
a. L = {s : s contains equal numbers of As and Bs} (already made)
b. L = {s : s is of the form AnBnfor some n>= 0} (this is the fucntion that needs to be added)
Also create a main with output to test both functions.
Code:
#ifndef STACK_INTERFACE_H
#define STACK_INTERFACE_H
#include
#include
#include
#include
using namespace std;
#include
template
class StackInterface
{
public:
/** Sees whether this stack is empty.
@return True if the stack is empty, or false if not. */
virtual bool isEmpty() const = 0;
/** Adds a new entry to the top of this stack.
@post If the operation was successful, newEntry is at the top of the stack.
@param newEntry The object to be added as a new entry.
@return True if the addition is successful or false if not. */
virtual bool push(const ItemType& newEntry) = 0;
/** Removes the top of this stack.
@post If the operation was successful, the top of the stack
has been removed.
@return True if the removal is successful or false if not. */
virtual bool pop() = 0;
/** Returns the top of this stack.
@pre The stack is not empty.
@post The top of the stack has been returned, and
the stack is unchanged.
@return The top of the stack. */
virtual ItemType peek() const = 0;
}; // end StackInterface
#endif
//////////////////////////////////////////////////////////////
#ifndef NODE_H
#define NODE_H
template
class Node
{
private:
ItemType item; // A data item
Node
public:
Node();
Node(const ItemType& anItem);
Node(const ItemType& anItem, Node
void setItem(const ItemType& anItem);
void setNext(Node
ItemType getItem() const ;
Node
}; // end Node
#endif
///////////////////////////////////////////////////////////////////////////////////
//#include "Node.h"
//#define nullptr NULL
#include
template
Node
{
} // end default constructor
template
Node
{
}
// end constructor
template
Node
item(anItem), next(nextNodePtr)
{
} // end constructor
template
void Node
{
item = anItem;
} // end setItem
template
void Node
{
next = nextNodePtr;
} // end setNext
template
ItemType Node
{
return item;
} // end getItem
template
Node
{
return next;
} // end getNext
///////////////////////////////////////////////////////////////////////////////////
#ifndef _LINKED_STACK
#define _LINKED_STACK
//#include "StackInterface.h"
//#include "Node.cpp"
//#define nullptr NULL
template
class LinkedStack : public StackInterface
{
private:
Node
// this node contains the stack's top
public:
// Constructors and destructor:
LinkedStack(); // Default constructor
LinkedStack(const LinkedStack
virtual ~LinkedStack(); // Destructor
// Stack operations:
bool isEmpty() const;
bool push(const ItemType& newItem);
bool pop();
bool checkString(string s);
ItemType peek() const;
}; // end LinkedStack
#endif
///////////////////////////////////////////////////////////////
#include
//#include "LinkedStack.h" // Header file
template
LinkedStack
{
} // end default constructor
template
LinkedStack
{
// Point to nodes in original chain
Node
if (origChainPtr == nullptr)
topPtr = nullptr; // Original stack is empty
else
{
// Copy first node
topPtr = new Node
topPtr->setItem(origChainPtr->getItem());
// Point to last node in new chain
Node
// Advance original-chain pointer
origChainPtr = origChainPtr->getNext();
// Copy remaining nodes
while (origChainPtr != nullptr)
{
// Get next item from original chain
ItemType nextItem = origChainPtr->getItem();
// Create a new node containing the next item
Node
// Link new node to end of new chain
newChainPtr->setNext(newNodePtr);
// Advance pointer to new last node
newChainPtr = newChainPtr->getNext();
// Advance original-chain pointer
origChainPtr = origChainPtr->getNext();
} // end while
newChainPtr->setNext(nullptr); // Flag end of chain
} // end if
}
// end copy constructor
template
LinkedStack
{
// Pop until stack is empty
while (!isEmpty())
pop();
}
// end destructor
template
bool LinkedStack
{
return topPtr == nullptr;
} // end isEmpty
template
bool LinkedStack
{
Node
topPtr = newNodePtr;
newNodePtr = nullptr;
return true;
} // end push
template
bool LinkedStack
{
bool result = false;
if (!isEmpty())
{
// Stack is not empty; delete top
Node
topPtr = topPtr->getNext();
// Return deleted node to system
nodeToDeletePtr->setNext(nullptr);
delete nodeToDeletePtr;
nodeToDeletePtr = nullptr;
result = true;
} // end if
return result;
} // end pop
template
ItemType LinkedStack
{
assert(!isEmpty()); // Enforce precondition
// Stack is not empty; return top
return topPtr->getItem();
} // end getTop
// End of implementation file.
/////////////////////////////////////
//a. L = {s : s contains equal numbers of As and Bs}
template
bool LinkedStack
{
//variables to store the count of A and B
int countA=0, countB=0;
//create the instance of linked stack to hold characters
LinkedStack
//loop invariant
int i=0;
//push the characters of the string into the stack
while(i { //push the character into the stack stackObj->push(s[i++]); } //pop the characters from the stack while(!stackObj->isEmpty()) { //extract the top element char top=stackObj->peek(); //if the character is A increment count of A if(top =='A') countA++; //if the character is B increment count of B if(top=='B') countB++; } //check the count of A and B, whether they are same if(countA == countB) return true; else return false; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
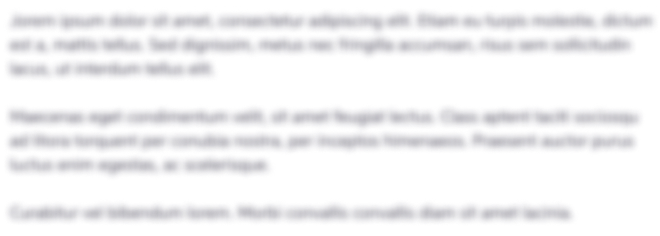
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started