Question
Data Structure: entire java(Data structures and algorithm) project must be contained in a single class called StringList Program Description: You are required to write a
Data Structure:
entire java(Data structures and algorithm) project must be contained in a single class called StringList
Program Description:
You are required to write a program that reads a text file and maintains a linked list of strings read from the input file. The program must also be interactive, i.e. it will read commands (described below) from stdin and print the results to stdout. The program should continue to process commands until the exit command is given, in which case your program should shut down. The purpose of this project is to observe the behavior of the linked list when maintaining a large number of objects. In particular, your program must perform the following steps:
1. When started, your program must prompt the user to enter the name of the file to be read. If the file does not exist, then your program should print an error message and shut down.
2. Once the file is opened, then your program must read the file line by line and insert each line into a linked list. The linked list must be in the same order as the lines in the file, i.e. the first line should be the first element in the linked list, then the second line, and so on.
3. After the file is processed, your program should prompt the user to enter commands. The program should wait for the user to enter the next command. Commands must be processed until the user enters the exit command. The result of each command must be displayed to stdout.
4. If the exit command is given, then your program must shut down.
Commands:
The following is the list of the 5 commands that your program must support. The only error cases that your program must handle are those mentioned in the command descriptions themselves.
(i) The Count Command: The syntax for the count command is" count " and when the count command is read from the user, your program must display the number of strings currently in the linked list.
(ii) The drop Command: The syntax for the drop command is "drop position" where position is either first or last. The behavior of this command depends on the value of position: If the argument first is provided, then your program must remove the string at the head of the linked list and display it to stdout. If the argument last is provided, then your program must remove the string at the tail of the linked list and display it to the stdout.
If an unrecognized argument is encountered to the drop command, then your program should print an error message and continue to process further commands. Additionally, if the list is currently empty, then your program must display an appropriate error message and continue to process further commands. (iii) The cut Command: The syntax for the cut command is "cut k " where k is an integer k >= 0. The behavior of this command depends on the state of the list. If the size of the list is at least k, then your program must remove the first k elements of the linked list and display them to stdout. If the size of the list is less than k, then your program must display an appropriate error message and then continue to process further commands. (iv) The cons Command: The syntax for the cons command is cons s where s is any string. To process this command your program must insert the string s to the front of the linked list currently being maintained. (v) The exit Command: The syntax for the exit command is "exit"which signals to the program to shut down. Additional Requirements: 1. Your program must import and use one of the linked list implementations that has been completed in class. Programs that re-implement a linked list will receive zero credit. You must use the implementations already completed in class. 2. Your class must follow standard OOP practice. For example, all necessary fields must be private, and methods should have the appropriate visibility specifiers. Your class must also provide a main method which contains all of the code necessary to read the commands from the user.
Program Outline: A high level outline of the program is provided below. 1 Prompt the user to enter the filename 2 if (file from the user exists) { 3 Read the file and insert each line into a linked list. 4 while(command != exit) { 5 process command 6 } 7 } else { 8 display an error message and exit. 9 }
SinglyLinkedList.java
package linkedLists; public class SinglyLinkedList { private static class Node { private E element; private Node next; public Node(E e, Node n) { element = e; next = n; } public E getElement() { return element; } public Node getNext() { return next; } public void setNext(Node n) { next = n; }
} private Node head = null; private Node tail = null; private int size = 0; public SinglyLinkedList() { } public int size() { return size; } public boolean isEmpty() { return size == 0; } public E first() { if(isEmpty()) return null; return head.getElement(); } public E last() { if(isEmpty()) return null; return tail.getElement(); } public void addFirst(E e) { head = new Node<>(e, head); if(isEmpty()) tail = head; size++; } public void addLast(E e) { Node newest = new Node<>(e, null); if(isEmpty()) head = newest; else tail.setNext(newest); tail = newest; size++; } public E removeFirst() { if(isEmpty()) return null; E temp = head.getElement(); head = head.getNext(); size--; if(size == 0) tail = null; return temp; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
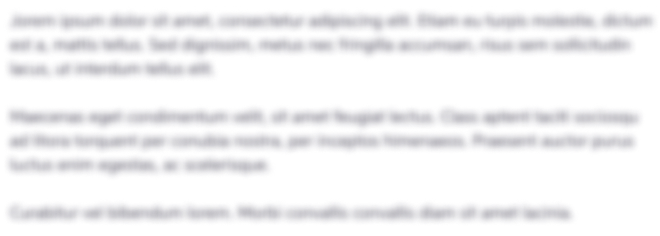
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started