Question
Data Structure in C++ Doubly Linked Lists of ints This is my code below: dlist.cc ------------------------------------------------------------------------------------------------------- #include #include dlist.h typedef dlist::node node; dlist::node* dlist::at(int n){
Data Structure in C++
Doubly Linked Lists of ints
This is my code below: dlist.cc
-------------------------------------------------------------------------------------------------------
#include
typedef dlist::node node;
dlist::node* dlist::at(int n){ node* c = _head; while(n > 0 && c){ c = c->next; n--; } return c; }
void dlist::insert(node* previous, int value){ node* n = new node{value, previous->next, previous}; previous->next = n; n->next->prev = n; }
void dlist::del(node* which){ node* nn = which->next; which->next = nn->next; delete nn; }
void dlist::push_back(int value){ node* n = new node{value, _tail, _tail->prev}; _tail->prev->next = n; _tail->prev-> n; }
void dlist::push_front(int value){ node* n = new node(value, nullptr); _head->prev = n; _head-> n; }
void dlist::pop_front(){ node* n = _head; _head = _head->next; delete n; }
void dlist::pop_back(){ node* n = _tail; _tail = _tail->prev; delete n; }
bool dlist::empty(){ return size == 0; }
/* out next;
while (c != l.tail()){ out value; out next; } out
bool operator== (dlist&a, dlist&b){ if(a.getSize() != b.getSize()){ return false; }
node* c1 = a.head(); node* c2 = b.head();
while(c1 != a.tail() && c2 != b.tail()){ if(c1->value != c2->value){ return false; }
c1 = c1->next; c2 = c2->next; }
return true; }
dlist operator+ (dlist&a, dlist&b){ dlist l; node* c = a.head(); node* d = b.head();
while(c->next != a.tail()){ l.push_back(c->next->value); c = c->next; }
return l; }
dlist reverse(dlist& l){ dlist a; node* c = l.head();
while(c->next != l.tail()){ a.push_front(c->next->value); c = c->next; }
return a; }
--------------------------------------------------------------------------------
I have two problems.
1. del() doesn't update the prev pointer.
2. see several syntax errors that would prevent it from compiling successfully.
Please fix and complete my code, and I also need whole code containing main function(list_tests.cc) for testing dlist.cc
--------------------------------------------------------------------------------
Sample output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
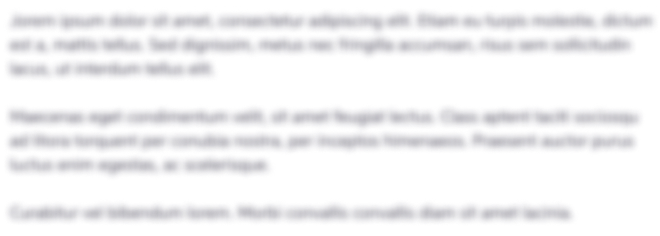
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started