Question
Data Structure in C++ Parsing Page Ranges I have a question regarding segmentation fault. I almost did this, but there is a segmentation fault when
Data Structure in C++
Parsing Page Ranges
I have a question regarding segmentation fault.
I almost did this, but there is a segmentation fault
when only I type 1 to 10
> 1,2,4,5 1,2,4,5 > 1 to 10 1,2,3,4,5,6,7,8,9,10 > 1,3 - 5,8 1, 3, 4, 5, 8 > 1 - 10 odd 1,3,5,7,9
How can I fix segmentation fault ?
I think the problem is in exprss function, when I do if (numbs(*start) && opps(*(start+1)) && numbs(*(start+2)) && is_qualifier(*(start+3))) { Teacher said: you haven't checked to make sure that finish >= start+3. I.e., there might not be four elements in the input sequence, in which case you'll read off the end.
Then how can I fix this
My code is :
pset.cc
#include
#include
using std::string;
using std::cout;
struct pset {
virtual void evaluate() = 0;
};
struct pset_num : public pset {
pset_num (int value) {
v = value;
}
void evaluate() {
cout << v << ", ";
}
int v;
};
struct pset_range : public pset {
pset_range(int start, int end) {
s = start;
e = end;
}
void evaluate() {
for (int i = s; i <= e; i++) {
cout << i << ", ";
}
}
int s;
int e;
};
struct pset_to : public pset {
pset_to(string to, int start, int end) {
st = to;
s = start;
e = end;
}
void evaluate() {
if(st == "-" || st == "to") {
for (int i = s; i <= e; i++) {
cout << i << ", ";
}
}
}
string st;
int s;
int e;
};
struct pset_r_odd : public pset {
pset_r_odd(int start, int end) {
s = start;
e = end;
}
void evaluate() {
for (int i = s; i <= e; i++) {
if (i % 2 == 1)
cout << i << ", ";
}
}
int s;
int e;
};
struct pset_r_even : public pset {
pset_r_even(int start, int end) {
s = start;
e = end;
}
void evaluate() {
for (int i = s; i <= e; i++) {
if (i % 2 == 0)
cout << i << ", ";
}
}
int s;
int e;
};
main.cc
#include "pset.cc"
#include
#include
using std::cin;
using std::cout;
using std::vector;
using std::string;
using std::endl;
using iter = vector::iterator;
bool numbs(string s) {
return (s.at(0) >= '0' && s.at(0) <= '9');
}
bool opps(string s) {
return (s == "to" || s == "-");
}
bool is_qualifier(string s) {
return (s == "even" || s == "odd");
}
bool exprss(iter start, iter finish);
void parse_pset(iter start, iter finish);
vector lex(string s);
vector ranges;
int main() {
string input;
while (true) {
cout << "> ";
std::getline(cin, input);
vector tokens = lex(input);
bool valid = exprss(tokens.begin(), tokens.end() - 1);
if (valid) {
cout << "Valid" << std::endl;
parse_pset(tokens.begin(), tokens.end() - 1);
for (pset* exp : ranges) {
exp->evaluate();
}
ranges.clear();
cout << std::endl;
}
return 0;
}
vector lex(string s) {
const int SPACE = 0;
const int NUM = 1;
const int WORD = 2;
const int OP = 3;
int state = SPACE;
vector words;
string word;
for (char c : s) {
if (state == SPACE) {
if (c == ' ' || c == ',')
continue;
else if (c >= '0' && c <= '9')
state = NUM;
else if (c >= 'a' && c <'z')
state = WORD;
else if (c == '-')
state = OP;
word.push_back(c);
}
else {
int nstate = state;
if (c == ' ' || c == ',')
nstate = SPACE;
else if (c >= '0' && c <= '9')
nstate = NUM;
else if (c >= 'a' && c <= 'z')
nstate = WORD;
else if (c == '-')
nstate = OP;
if (nstate == SPACE) {
words.push_back(word);
word.clear();
state = SPACE;
continue;
}
if (nstate == state && state != OP)
word.push_back(c);
else if (nstate != state || state == OP) {
words.push_back(word);
word.clear();
word.push_back(c);
}
state = nstate;
}
}
if (!word.empty())
words.push_back(word);
return words;
}
void parse_pset(iter start, iter finish) {
if (start > finish)
return;
else if (start == finish) {
if(numbs(*start))
ranges.push_back(new pset_num{ stoi(*start) });
}
else {
if (numbs(*start) && opps(*(start+1)) &&
numbs(*(start+2)) && is_qualifier(*(start+3))) {
if (*(start+3) == "odd") {
ranges.push_back(new pset_r_odd{stoi(*start),
stoi(*(start+2))});
parse_pset(start+4, finish);
}
else if (*(start+3) == "even") {
ranges.push_back(new pset_r_even{stoi(*start),
stoi(*(start+2))});
parse_pset(start+4, finish);
}
}
else if (numbs(*start) && opps(*(start+1)) &&
numbs(*(start+2))) {
ranges.push_back(new pset_range{stoi(*start),stoi(*(start+2))});
parse_pset(start+3, finish);
}
else if (numbs(*start)){
ranges.push_back(new pset_num{ stoi(*start)});
parse_pset(start+1, finish);
}
}
return;
}
bool exprss(iter start, iter finish) {
if (start > finish)
return true;
else if (start == finish) {
if(numbs(*start))
return true;
else
return false;
}
else {
if (numbs(*start) && opps(*(start+1)) &&
numbs(*(start+2)) && is_qualifier(*(start+3))) {
return exprss(start+4, finish);
} else if (numbs(*start) && opps(*(start+1)) &&
numbs(*(start+2))) {
return exprss(start+3, finish);
} else if (numbs(*start)) {
return exprss(start+1, finish);
}
}
return false;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
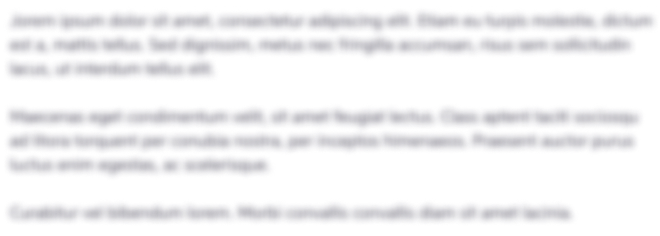
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started