Question
Data Structure in C++ The Problem with my code.. dlist.cc: In function 'std::ostream& operator <
Data Structure in C++
The Problem with my code..
dlist.cc: In function 'std::ostream& operator<<(std::ostream&, dlist&)': dlist.cc:66:10: error: invalid initialization of reference of type 'std::ostream& {aka std::basic_ostream&}' from expression of type 'dlist::node*' dlist.cc: In function 'dlist operator+(dlist&, dlist&)': dlist.cc:93:8: error: invalid operands of types 'dlist::node*' and 'dlist::node*' to binary 'operator+' dlist.cc:97:8: error: could not convert 'result' from 'int' to 'dlist'
and also my professor pointed out...
1. In your insert you are not updating previous->next->prev to point to the new node.
2. Similarly for del. Check your list-modifying methods to make sure they are correctly updating *both* the next and prev pointers.
3. operator+ returns a dlist, not an int.
How can I fix these problems??
My code:
#include "dlist.h"
dlist::node*dlist::at(int n){
node*current = head();
while(n != 0){
current= current->next;
n--;
}
return current;
}
void dlist::insert(node *previous, int value){
node*n = new node{value, previous -> next,previous};
previous -> next = n;
}
void dlist::del(node* which){
node*c= which -> next;
which -> next = which-> next-> next;
delete c;
}
void dlist::push_back(int value){
node*c = tail();
insert(c,value);
}
void dlist::push_front(int value){
insert(head(), value);
}
void dlist::pop_front(){
node*n = head();
n = head() -> next;
delete n;
}
void dlist::pop_back(){
node*n = tail();
n = tail() -> prev;
delete n;
}
int dlist::size(){
node*c = head();
int s = 0;
while(c){
c = c -> next;
s++;
}
return s;
}
bool dlist::empty(){
if(size() == 0)
return true;
else
return false;
}
std::ostream& operator<< (std::ostream& out, dlist& l){
dlist::node*c = l.head();
while(true){
out << c;
c = c-> next;
return c;
}
}
bool operator== (dlist& a, dlist& b){
dlist::node* ca = a.head();
dlist::node* cb = b.head();
while(ca&&cb){
if(ca-> next != cb->next)
return false;
else{
ca = ca->next;
cb = cb->next;
}
}
if(ca== nullptr && cb == nullptr)
return true;
else
return false;
}
dlist operator+ (dlist& a, dlist& b){
dlist::node* ca = a.head();
dlist::node* cb = b.head();
int result;
while(ca&&cb){
ca + cb = result;
ca = ca -> next;
cb = cb -> next;
}
return result;
}
dlist reverse(dlist& l){
dlist l2;
dlist::node* c = l.head();
while(c->next!=l.tail()){
l2.push_front(c->next->value);
c = c-> next;
}
return l2;
}
"dlist.h"
#pragma once
/*
dlist.h
Doubly-linked lists of ints
*/
#include
class dlist {
public:
dlist() { }
// Implement the destructor, to delete all the nodes
~dlist();
struct node {
int value;
node* next;
node* prev;
};
node* head() const { return _head; }
node* tail() const { return _tail; }
// **** Implement ALL the following methods ****
// Returns the node at a particular index (0 is the head).
node* at(int);
// Insert a new value, after an existing one
void insert(node *previous, int value);
// Delete the given node
void del(node* which);
// Add a new element to the *end* of the list
void push_back(int value);
// Add a new element to the *beginning* of the list
void push_front(int value);
// Remove the first element
void pop_front();
// Remove the last element
void pop_back();
// Get the size of the list
int size();
// Returns true if the list is empty (size == 0)
bool empty();
private:
node* _head = nullptr;
node* _tail = nullptr;
};
// **** Implement ALL the following functions ****
/* out << l
Prints a list to the ostream out. This is mostly for your convenience in
testing your code; it's much easier to figure out what's going on if you
can easily print out lists!
*/
std::ostream& operator<< (std::ostream& out, dlist& l);
/* a == b
Compares two lists for equality, returning true if they have the same
elements in the same positions. (Hint: it is *not* enough to just compare
pointers! You have to compare the values stored in the nodes.)
*/
bool operator== (dlist& a, dlist& b);
/* a + b
Returns a new list consisting of all the elements of a, followed by all the
elements of b (i.e., the list concatenation).
*/
dlist operator+ (dlist& a, dlist& b);
/* reverse(l)
Returns a new list that is the *reversal* of l; that is, a new list
containing the same elements as l but in the reverse order.
*/
dlist reverse(dlist& l);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
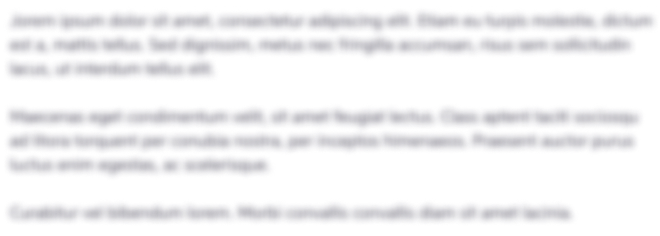
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started