Question
Data Structures and Object-Oriented Programming (C++) The IntArray class holds an integer array. The class interface makes it easy to copy, assign, lookup and modify
Data Structures and Object-Oriented Programming (C++)
The IntArray class holds an integer array. The class interface makes it easy to copy, assign, lookup and modify elements of the array. Build an IntArray class with the following member functions and operators.
Member Function | Description of how it works |
IntArray(const char* str) | Make an IntArray such that each element as the ASCII value of each character in the str. For example: IntArray(ABC) produces an array with the values: {65, 66, 67} /* note, not legal syntax, just a representation */ |
IntArray(int size, int val=0) | Create an array of length size all initialized to val |
int& operator[](int index) | Return a reference to the indexed location in the array. IntArray a(3,0); a[0] = 5; // set first location to 5 a[1] = a[0]; // calls operator[] twice |
IntArray& operator+=(const IntArray &rhs); | Accumulate rhs into the object and a return a reference to the object. Keep size of this object fixed even if rhs is bigger. If rhss array is larger, just ignore those elements. |
IntArray operator+(const IntArray &rhs) const | Add two IntArray objects together in a new IntArray object, and return the new object. The new object has an array the same size as that in the lhs object. If the rhs is smaller, just copy over the extra ones unmodified. |
IntArray operator-(const IntArray &rhs) const; | Subtract two IntArray objects into a new IntArray object, and return the new object. For example, if the lhs was {1, 2, 3} and the rhs was {1, 2, 3}, the new array would be {0,0,0}, because we pair-wise subtracted the elements. The new object has an array the same size as that in the lhs object. If the rhs is smaller, just copy over the extra ones unmodified. |
IntArray operator*(int x) const; | Multiply array by integer x. If the array was {1, 2, 3} and x=3, then the return value is a new array with {3, 6, 9}. The new object has an array the same size as that in the lhs object. |
IntArray operator/(int x) const; | Divide array by integer x. If the array was {2, 4, 6} and x=3, then the return value is a new array with {0, 1, 2}. The new object has an array the same size as that in the lhs object. |
IntArray operator<<(int count) const; | Rotate the contents of the array toward higher indices by count amount. If the array was length 3 and started out as {1, 2, 3}, it now becomes {3, 1, 2}. IntArray a(3); a[0] = 1; a[1] = 2; a[2] = 3; IntArray b(3,0); b += a << 2; // now b has {2, 3, 1} The new object has an array the same size as that in the lhs object. |
operator char*() const; | Type convert the IntArray to a string on the heap and return a pointer to it. If an integer value is out of range ( less than 0 or greater than 255), make it a space. 0 becomes the null character. If there is no 0 in the array, add a null character to the end. For example an array with {65, 9000, 67}, becomes A C. |
operator int() const; | Return the average value of the array.
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
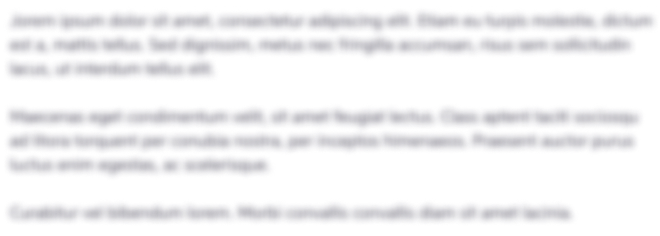
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started